AP Computer Science - Midterm Solutions
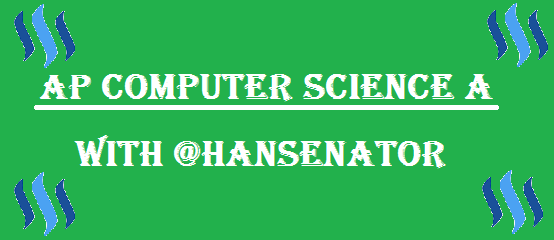
True/False:
F 1. There are 8 bytes in a bit.
T 2. An example of an output device would be a monitor.
T 3. An interpreter or compiler translates a source program into a readable output for the user.
T 4. An integer can have the type double in Java.
F 5. This line in a program will display the number 45 for its output… System.out.println(“95”);
F 6. Keyboards are considered software.
T 7. In Java, // This is an appropriate symbol to make a comment.
T 8. A Java program is executed from the main method in the class.
T 9. double $tarter$; // This could be an identifier or variable.
F 10. double 4star; // This could be an identifier or variable.
F 11. System.out.printf(“%.2f” + 25.444); will print 25.44
T 12. System.out.printf(“%.2f%10s”, 2.2, “Hello”); will display: 2.20 Hello
T 13. If you want a number formatted in scientific notation you use %e.
F 14. Strings can be inserted into the formatting method by concatenating with +’s.
F 15. String is known as a primitive data type in Java.
T 16. char would be the best data type to input a letter grade (A, B, C…).
F 17. 3 + 25 – 1 would give the output 24.
T 18. If x=2 and z=3, the following will be false: (x <= z && x > 20)
F 19. The short circuit operations are true && (anything) and false || (anything).
T 20. Math.pow(Math.abs(3-6), Math.sqrt(4)) yields 9.
Short Answer:
Number 1
Determine if the following are equivalent using truth tables:
A | B | ~(A and B) | ~A or ~B |
---|---|---|---|
T | T | F | F |
T | F | T | T |
F | T | T | T |
F | F | T | T |
Circle: Yes or No
Number 2
Write a for loop that displays the following: 0 3 6 9 12 15 18 21 24
for ( int i=0 ; i <= 8 ; i++ )
System.out.print( i*3 + “ “ );
Number 3
Write the output (as the computer would) of the following program section:
int number = 0;
for ( i = 1; i <=4; i++) {
while ( i <= 2 ) { number = number * 2; i++ }
System.out.print(number + “ ”);
number = number + 2;
}
ANSWER:
0 2
Number 4
Draw a Venn Diagram for (A && !B) || !(A || B)
Number 5
Write the output (as the computer would) for: System.out.printf(“%.2f%6s\n%s”,24.5624,”Hello”,”GoodBye”);
ANSWER:
24.56 Hello
GoodBye
Vocabulary:
G 1. Boolean
F 2. Syntax
I 3. Truncate
H 4. Constructor
E 5. Class
K 6. final
L 7. Object
C 8. for
B 9. Method
J 10. while
Vocabulary Bank:
a. The section of a code in which a variable exists. SCOPE
b. A set of code which is referred to by name and can be called (invoked) at any point in a program simply by utilizing its name.
c. Used when you know how many times you need to loop.
d. A collection of data items, all the same type, in which each item's position is uniquely designated by an integer. ARRAY
e. The blueprint for which individual objects are created.
f. The rules of combining words to create statements, in other words how a Java program is written and interpreted.
g. The expression in an if or else-if statement must be of this type.
h. Creates new objects, has the same name as the class, and has no return type.
i. To shorten or cut off part of.
j. Used to loop until an event occurs.
k. A keyword in Java that declares a variable that cannot change.
l. A value of a class type.
Bonus: Identify the definitions that were not used by circling the letter and giving the definition.
Free Response
Finish the program where indicated:
public abstract class Shape {
private String name;
// Constructor
public Shape(String shapeName)
{ name = shapeName; }
public String getName()
{ return name; }
public abstract double area();
public abstract double perimeter();
public double semiPerimeter()
{ return perimeter()/2; }
}
public class Square extends Shape {
private double side;
// Constructor
public Square(double squareSide, String squareName) {
super(squareName);
side = squareSide;
}
public double perimeter()
{
// Write a return statement for perimeter
return side4;
}
public double area()
{
// Write a return statement for area
return sideside;
}
}
public class MAIN {
public static void main(String[] args) {
// Create (one line) a Square Object side length of 4 named “MySquare”.
// Hint: SUPERCLASS name = new SUBCLASS(side, “name”);
Shape MySquare = new Square(4, “MySquare);
// Print the perimeter, semiperimeter, area, and name of the new object in four separate print statements that also describe what it is printing.
System.out.println(“The perimeter is: ” + MySquare.perimeter());
System.out.println(“The semiperimeter is: ” + MySquare.semiPerimeter());
System.out.println(“The area is: ” + MySquare.area());
System.out.println(“The name is: ” + MySquare.getName());
}
}

All SteemitEducation Posts
Physics
Kinematics Video, Circular Motion Video, Forces in 1 & 2 Dimensions Videos, Kinematics Full Lesson, Circular Motion and Gravitation Full Lesson, Kinematics and Forces Quiz, Forces in 1-Dimension, Forces in 2-Dimensions, Basic Physics, Kinematics, and Forces Review, AP Physics Exam 1
Computer Science
1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15
SAT
Asymptotes, Composite Functions, Contest 1 - Area, Contest 1 – Winners and Solution, PEMDAS, Systems of Equations, Functions, Exponents, Contest 2 - More Functions, Contest 2 – Winners and Solution, Percents, The Interest Equation, All About Division, Contest 3 - Factoring, Contest 3 – Winners and Solution, Fractional and Negative Exponents, Basic Trig, Contest 4 - Math Vocab, Contest - 5, Contest 5 – Winners and Solution, Contest 6,
General Math
Basics of Algebra, Trigonometry Video, Proof of Quadratic Formula, Factoring Video, Unions and Intersections, Surface Area of a Cylinder, Substitution Video, Combinatorics Basics
Graphing from Algebra to Calculus
Algebra 1, Algebra 2, Pre-Calculus, Calculus
Piloting Airplanes
Introduction, Preflight, Requirements, Instruments, Take-Offs and Landings, Why a Plane Can Fly, Reading the Runway, Maneuvers
Other
Engineering (Isometric) Drawing, Pressure & SCUBA Diving

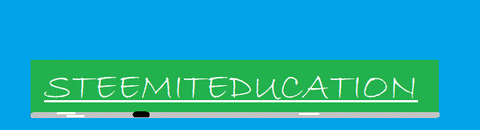
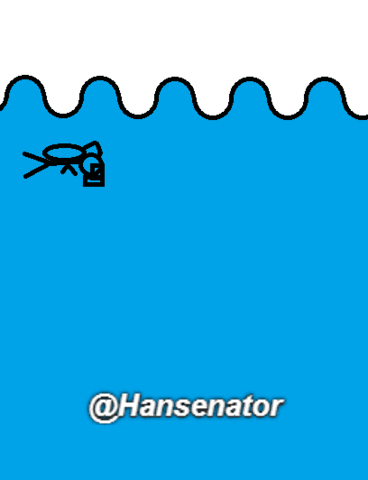
excellent information & thnx for sharing friend @hansenator
thanks for sharing@hansenator
Thanks for the comment!
Hello sir its @xabi here....
When you will be hosting the next contest...I would love to participate...
Thanks for my first contest win on Steemit...your contest win really encouraged me to keep steeming...
I will get one out soon for you xabi!
I will be waiting
Great post @hansenator.
Thank you for visiting my blog and also provide upvote.
Best Regards @nafazul.
Hey nafazul! Happy to be there for you!
Thanks @hansenator.
beautiful teaching system.thanks for sharing @hansenator
Thank you reyjahmedrazu! Hoping some of it sticks in their brains. Haha.
very informative hansen
This post has received a 100% upvote worth $0.35 from @minnowspower thanks to: @theguruasia
MinnowsPower is not a bot, I am a Crowdfunding Hybrid
One small UpVote of Yours build MinnowsPower and MinnowsPower will Not Forget Your Support...!!!
I will follow the following person and give my phone number
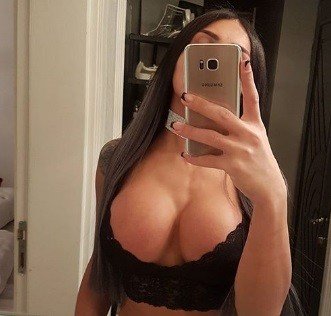
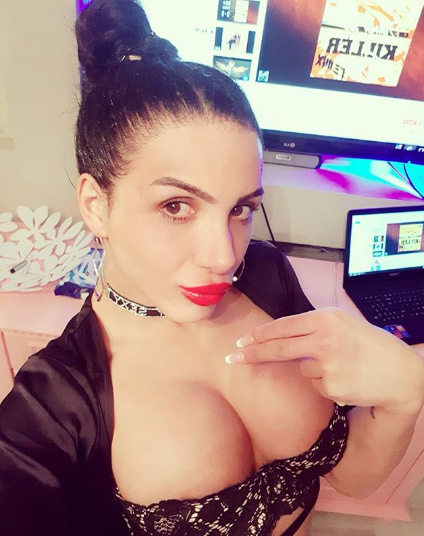
nice... Follow me ... i will give my phone number <3