AP Computer Science Java Programming - Algorithms and Arrays
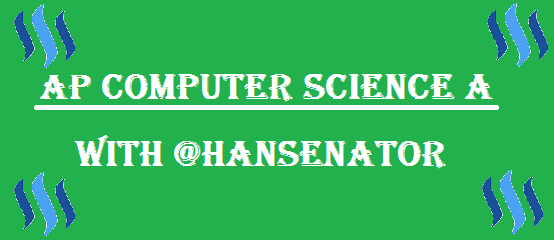
Know this Steemers, when learning to read algorithms you need to read line by line, like a book, very slowly. If you do not it will be way harder than it has to be! Note: due to html/steemit some of the code is spaced because otherwise it will not show up (the programs will not run by if copy/pasted). Enjoy today's lesson!
Arrays
• An array is a “list” of strings or numbers.
• Designated List< int> name = new ArrayList< int>(); // Replace int with String, double, etc. (no spaces in < int >)
• Ex. – Say we had an array called names = [“John”, “Billy”, “Tom”, “Jerry”]. What is names[1]? Billy (because we count 0, 1, 2...)
AP Sample Problems
Example 1
Consider the following partial code:
for (int k = 0; k < 20; k = k + 2) { // k += 2 also works
if (k % 3 == 1) {
System.out.print(k + “ “);
}
}
What does it print?
- Remember the % operator gives you the remainder, so all multiples of two, with remainder 1 when divided by 3, is what the program prints... Up to less than 20. (k + “ “) adds a space between each number.
- Answer: 4 10 16
Example 2
Consider the partial program:
List< String> animals = new ArrayList< String>;
animals.add(“dog”);
animals.add(“cat”);
animals.add(“snake”);
animals.set(2, “lizard”);
animals.set(1, “fish”);
animals.remove(2);
System.out.println(animals);
- The real thing to remember here is that element 0 is the first element, so the last element is the size of the array minus 1.
- Answer: ["dog", "fish"]
Example 3
Consider the following method:
public static void main(String[] args) {
for (int k = 0; k < nums.size(); k++) {
if (nums.get(k).intValue() == 0) {
nums.remove(k);
}
}
}
Assume that a List< integer> values initially contains the following
values:
[0, 0, 4, 2, 5, 0, 3, 0]
What will the main method print when it executes?
- [0, 4, 2, 5, 3] because when the loop runs it deletes the first zero, skips the second zero because it moves on the the second element, but gets rid of the rest of the zeros.
All SteemitEducation Posts
Physics
Kinematics Video, Circular Motion Video, Forces in 1 & 2 Dimensions Videos, Kinematics Full Lesson, Circular Motion and Gravitation Full Lesson
Computer Science
SAT
Asymptotes, Composite Functions, Contest 1 - Area, Contest 1 – Winners and Solution, PEMDAS, Systems of Equations, Functions, Exponents, Contest 2 - More Functions, Contest 2 – Winners and Solution, Percents, The Interest Equation, All About Division, Contest 3 - Factoring, Contest 3 – Winners and Solution, Fractional and Negative Exponents, Basic Trig
General Math
Basics of Algebra, Trigonometry Video, Proof of Quadratic Formula, Factoring Video, Unions and Intersections, Surface Area of a Cylinder, Substitution Video, Combinatorics Basics
Graphing from Algebra to Calculus
Algebra 1, Algebra 2, Pre-Calculus, Calculus
Piloting Airplanes
Introduction, Preflight, Requirements, Instruments, Take-Offs and Landings, Why a Plane Can Fly, Reading the Runway, Maneuvers
Other
Engineering (Isometric) Drawing, Pressure & SCUBA Diving

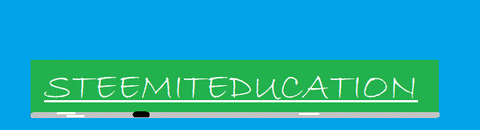
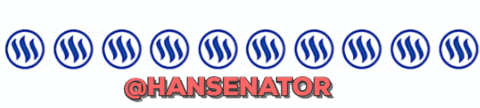