AP Computer Science - Java Language Features (6)
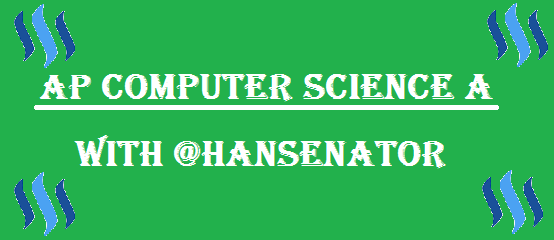
Another productive day in class. I need to get better at posting my lesson plans before class. But hey, as long as they get here, right? We mainly had a practice day with some programming labs. I gave the students 2 for a quiz and did one with them myself... I will also include the program that prints what time it is! Here was my Q&A with them today!
The Quiz
- Make a program that asks the user how many times they want the words "Hello World" to appear on the screen.
import java.util.Scanner;
public class HelloWorldX {
public static void main(String[] args) {
// Ask the user
System.out.println("How many times would you like to print "Hello World"?);
// Call the scanner function
Scanner input = new Scanner(System.in);
int number = input.nextInt();
// A for loop with their number
for (i = 1; i <= number; i++) {
System.out.println("Hellow World");
}
}
}
- Make a program that asks the user to find the area of a triangle with their given parameters. (My student Joey wrote this program and got a 100 on the quiz. His prize was a 1 oz piece of copper. Haha.)
import java.util.Scanner;
public Class AreaTriangle {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Please provide a height and the base length of the triangle you wish to solve: ");
int base = input.nextInt();
int length = input.nextInt();
int area = base * length / 2;
System.out.println("The area of the triangle is: " + area);
}
}
Classwork
- Randy Marsh wants a program that tells him to buy/sell/hold bitcoin. Have the user enter the price of bitcoin and tell Randy what he needs to do. If the price is less than 3000, then buy. If the price is greater than 5000, then sell. Otherwise, hold.
import java.util.Scanner;
public class StockMarket {
public static void main(String[] args) {
System.out.print("Enter the stock price: ");
Scanner input = new Scanner(System.in);
double stock = input.nextDouble();
String suggestion;
if (stock > 5000) {
suggestion = "sell";
}
else if (stock < 3000) {
suggestion = "buy";
}
else {
suggestion = "hold";
}
System.out.println("Randy, you should " + suggestion);
}
}
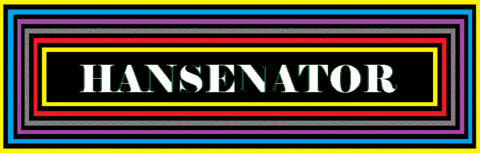
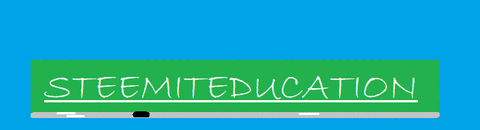.gif)