Part 24: An In Dept Look At Steem Operation Types Part I
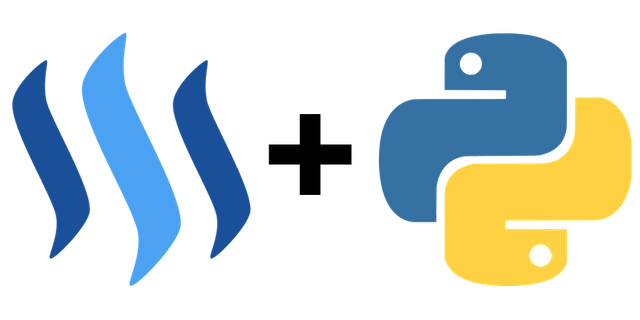
This tutorial is part of a series where different aspects of programming with steem-python
are explained. Links to the other tutorials can be found in the curriculum section below. This part is a direct continuation of Part 23: Retrieve And Process Full Blocks From The Steem Blockchain and will go into more detail following operation types
: transfer
, transfer_to_vesting
, withdraw_vesting
and convert
.
Repository
https://github.com/steemit/steem-python
What will I learn
- How to access data in a operation
- What is a transfer?
- What is a transfer_to_vesting
- What is a withdraw_vesting
- What is a convert?
Requirements
- Python3.6
steem-python
Difficulty
- basic
Tutorial
Setup
Download the files from Github. There 2 are files get_blocks.py
which contains the code. The main file takes 3 arguments from the command line which sets the starting_block
, block_count
and what operation
to filter for.
Run scripts as following:
> python get_block.py 22224731 10 transfer
How to access data in a operation
The previous tutorial went into great detail how to extract operations
from blocks
. There are 19 different operations
in total and each has a different structure. However, the data for the operation
is always stored in the same spot.
{
'ref_block_num': 8004,
'ref_block_prefix': 1744181707,
'expiration': '2018-05-07T15:29:54',
'operations': [
['vote', {
'voter': 'acehjaya',
'author': 'zunaofficial79',
'permlink': 'kabut-pagi',
'weight': 10000
}]
],
'extensions': [],
'signatures': ['1f205086670c27365738697f0bc7cfde8b6e976608dc12b0d391b2b85ad7870a002313be4b09a358c30010be2a09bebacead866ba180afc348ce40c70566f4ed88']
}
Under operations
is an array, the first index is the operation type
and the second index is a json table
with the operation data
.
if transaction['operations'][0][0] == self.tag:
self.process_operation(transaction['operations']
[0][1],
self.tag)
Filter for the required operation type and process the json data
. For this example tutorial a different class
is made for each operation type
which stores the data in respective variables
and has a print function
. These classes can be found in the operations.py
file.
What is a transfer?
Every transfer
of either Steem
or SBD
between two different user accounts is a transfer
operation. This operation has 4 variables. from
is the account that sends the funds, to
the receiving account, amount
the valuation and which currency, and optionally memo
. In which messages can be added.
{
'from': 'yusril-steem',
'to': 'postpromoter',
'amount': '5.000 SBD',
'memo': 'https://steemit.com/life/@yusril-steem/our-challenges-and-trials-in-life'
}
By using a class
to process the operation
all the variables
can be neatly kept and additional functions
can be added as well. Like the print_operation()
which allows for easy printing to the terminal. A separate class
is made for each of the operations
discussed in this tutorial.
class Transfer():
def __init__(self, operation, block, timestamp):
self.account = operation['from']
self.to = operation['to']
self.amount = operation['amount']
self.memo = operation['memo']
self.block = block
self.timestamp = timestamp
def print_operation(self):
print('\nBlock:', self.block, self.timestamp)
print('Operation: transfer')
print('From:', self.account)
print('To:', self.to)
print('Amount:', self.amount)
print('Memo:', self.memo)
print('')
Additional data that normally falls outside the scope of the operation
can also be stored in the class
. Like the block
in which the operation
took place and the timestamp of the operation
.
What is a transfer_to_vesting
A power up
of Steem
by a user is known as a transfer_to_vesting
and contains 3 variables
. from
the user account itself, to
the receiving account and amount
the amount being powered up. This operation
locks up the Steem
for 13 weeks.
{
'from': 'flxsupport',
'to': 'ltcil',
'amount': '0.719 STEEM'
}
What is a withdraw_vesting
Opposite to a transfer_to_vesting
is a withdraw_vesting
. When a user initiates a power down
the amount of steem power
will be powered down in 13 weekly increments. Vested steem power
is displayed in VESTS
and has to be converted to Steem
based on the current conversion rate. This is outside the scope of this tutorial but can be found here. This operation
holds 2 different variables
. account
the user account and vesting_shares
the amount of VESTS
to be converted to Steem
at the time of the operation.
{
'account': 'bazimir',
'vesting_shares': '44671.906021 VESTS'
}
What is a convert?
It is possible to convert SBD
to Steem
on the internal market
. This operation
assumes that the value of SBD
is at $1.0, which may not be true. Always check the external markets
before doing such an operation
. After 3 days the operation
matures and the user will receive the Steem
. This operation
holds 3 different variables
: amount
the amount of SBD
to be converted, owner
the account initiating the conversion and requestid
the id for the system to perform the conversion after 3 days.
{
'amount': '5000.0 SBD',
'owner': 'steemit',
'requestid': 1467592156
}
Running the script
Running the script will print the operations
, for which the filter has been set, to the terminal starting from the starting_block
for block_count
amount of blocks
. A class
object is made for the operation
and the build in print_operation()
function is called. The data of the operation
is printed to the terminal with the additional information of the block
in which the operation
is contained and the timestamp
of the operation
.
python get_blocks.py 22224731 10 transfer
Booted
Connected to: https://rpc.buildteam.io
Block: 22224731
Block: 22224731 2018-05-07T15:20:03
Operation: transfer
From: hottopic
To: jgullinese
Amount: 0.001 SBD
Memo: Hello Friend, Your post will be more popular and you will find new friends.We provide "Resteem upvote and promo" service.Resteem to 18.000+ Follower,Min 45+ Upvote.Send 1 SBD or 1 STEEM to @hottopic (URL as memo) Service Active
Block: 22224731 2018-05-07T15:20:03
Operation: transfer
From: minnowbooster
To: msena
Amount: 0.001 SBD
Memo: You got an upgoat that will be done by bycz,greece-lover,hemanthoj,percygeorge,bigdave2250,mexresorts,wanxlol,nickmorphew,thauerbyi,blackbergeron,aziz96,lovlu,utube,lowestdefinition. We detected an open value of 0.0$, worth 0.001 SBD in send and refunding that! Request-Id: 1176288
...
...
...
Curriculum
Set up:
- Part 0: How To Install Steem-python, The Official Steem Library For Python
- Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
Filtering
- Part 2: How To Stream And Filter The Blockchain Using Steem-Python
- Part 6: How To Automatically Reply To Mentions Using Steem-Python
- Part 23: Part 23: Retrieve And Process Full Blocks From The Steem Blockchain
Voting
- Part 3: Creating A Dynamic Autovoter That Runs 24/7
- Part 4: How To Follow A Voting Trail Using Steem-Python
- Part 8: How To Create Your Own Upvote Bot Using Steem-Python
Posting
- Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
- Part 7: How To Schedule Posts And Manually Upvote Posts For A Variable Voting Weight With Steem-Python
Constructing
Rewards
- Part 9: How To Calculate A Post's Total Rewards Using Steem-Python
- Part 12: How To Estimate Curation Rewards Using Steem-Python
- Part 14: How To Estimate All Rewards In Last N Days Using Steem-Python
Transfers
- Part 11: How To Build A List Of Transfers And Broadcast These In One Transaction With Steem-Python
- Part 13: Upvote Posts In Batches Based On Current Voting Power With Steem-Python
Account Analysis
- Part 15: How To Check If An Account Is Following Back And Retrieve Mutual Followers/Following Between Two Accounts
- Part 16: How To Analyse A User's Vote History In A Specific Time Period Using Steem-Python
- Part 18: How To Analyse An Account's Resteemers Using Steem-Python
The code for this tutorial can be found on GitHub!
This tutorial was written by @juliank.
I thank you for your contribution. Here are my thoughts on your post;
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @steempytutorials
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Thank you for your very good tutorial !