Part 13: Upvote Posts In Batches Based On Current Voting Power With Steem-Python
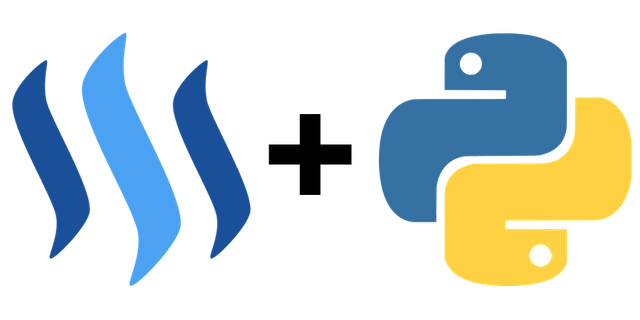
This tutorial is part of a series where different aspects of programming with steem-python
are explained. Links to the other tutorials can be found in the curriculum section below. This part is a direct continuation on Part 3: Creating A Dynamic Autovoter That Runs 24/7. In part 3 a dynamic automated upvoter is built that upvotes authors
from a list with a set upvote weight
and total upvote
per author
limit. The upvotes are executed directly after the post is seen on the blockchain. This part will instead add the post from the author
to a queue and upvotes the entire queue when 100% voting power
for the account
is reached.
What will I learn
- Build a queue
- Upvote a batch of posts
- Retrieve account voting power
Requirements
- Python3.6
steem-python
Difficulty
- Basic
Tutorial
Setup
Download the files from Github.batch_upvoter.py
contains the script while upvote_list.json
is a list of author that the script will upvote.
author in upvote_list.json
"steempytutorials": {
"upvote_weight": 100,
"upvote_limit": 1
}
Run the script as normal
> python batch_upvoter.py
Build a queue
Instead of directly upvoting the post. The post will be added to a queue. The queue is a dict and for every post the identifier
and upvote_weight
for the given author
are used as a pair.
upvote_queue = {}
for each post:
if post['identifier'] not in upvote_queue:
upvote_queue[post['identifier']] = upvote_list[author]["upvote_weight"]
Upvote a batch of posts
When an upvote cycle is started all posts are taken from the dict and individually upvoted. As there is a 3 second cooldown on upvoting on the Steem blockchain after the upvote the script waits for 3 seconds.
for identifier in upvote_queue:
upvote_weight = upvote_queue[identifier]
try:
print ("Upvoting {} for {} %".format(identifier, upvote_weight))
steem.vote(identifier, upvote_weight, account)
print ("Succes, sleeping for 3 seconds")
time.sleep(3)
except Exception as e:
print (repr(e))
Retrieve account voting power
The account
object contains valuable information for each account, like the current voting power
. The standard function voting_power()
retrieves the height of the voting power for the last upvote made by the account. To get the current voting power this function has to be replaced with the following code.
The function is located in account.py
from steem-Python
def voting_power(self):
diff_in_seconds = (datetime.datetime.utcnow() - parse_time(
self["last_vote_time"])).seconds
regenerated_vp = diff_in_seconds * 10000 / 86400 / 5
total_vp = (self["voting_power"] + regenerated_vp) / 100
if total_vp>100:
return 100
else:
return "%.2f" % total_vp
Now the current height of the accounts voting power
can be used to start an upvote cycle.
from steem.account import Account
account = Account(account)
# start upvoting cycle at 100% voting power
if account.voting_power() == 100:
start_upvote_cycle(upvote_queue, account)
Running the script
Set account
to your own account. Running the script will have the program listen in on the Steem blockchain for posts made by authors
in upvote_list.json
. These posts are added to a queue wich is emptied when 100% voting power
is reached. Use the standard list or alter the list to your own liking.
Curriculum
The code for this tutorial can be found on GitHub!
This tutorial was written by @juliank in conjunction with @amosbastian.
Posted on Utopian.io - Rewarding Open Source Contributors
Postingan perfeck
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
This post has been upvoted for free by @nanobot with 5%!
Get better upvotes by bidding on me.
Hey, I guess there are a few things that could be improved.
A dict is not a queue and not ordered. This means that the voter will not necessarily vote the posts in the same order they were added to the dict. If you want to maintain the order, use OrderedDict, list or multiprocessing.queue. If you're fine with any order, then calling it a "queue" is a little misleading.
For the VP calculation:
.seconds
will give wrong results if the time difference is larger than a day. Use.total_seconds()
instead.voting_power()
as shown here returns a string for <100% and an integer for =100%. Things like starting the voter at >80% VP for example get nasty with this implementation.Additionally, you should really look into the
datetime
package. Converting a timediff to string to extract hours and minutes is not the most elegant way :)Perfeck post @steempytutorial...Introductions i am @crokkon
useful info I will resteem let my friends can join.