Part 18: How To Analyse An Account's Resteemers Using Steem-Python
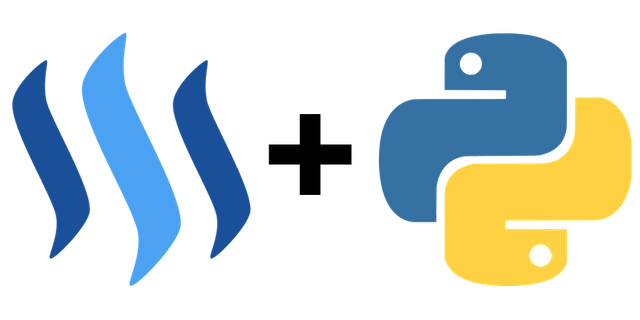
This tutorial is part of a series where we explain different aspects of programming with steem-python
. Links to the other tutorials can be found in the curriculum section below. In part 9 of this series we learned how to calculate the total rewards of a post and in this tutorial we will learn how to estimate the curation rewards of a post!
What will I learn
- How to iterate over an account's blog posts
- How to find out who resteemed a post
- How to print your top 10 resteemers
Requirements
- Python3.6
steem-python
Difficulty
- Basic
Tutorial
If you find it easier to follow along this way you can get the entire code for this tutorial from here.
Getting an account's blog posts
In the previous tutorials we used the function get_history_reverse()
to iterate over an account's history and filtered it by "comments" to get the posts they made. Today we are going to use a different, and better way to do this. Instead of using the get_history_reverse()
function to get all operations by an account and then filtering it, we can simply use the get_blog()
function, that takes an account
, entry_id
and limit
as arguments. So if you wanted to iterate over the last 20 posts an account made, you could use
for post in steem.get_blog(account, 0, 20):
post = Post(post["comment"])
# DO SOMETHING
It's that easy! Of course you still need to check if the post is a main post or a comment, and also if it's not someone else's post (resteemed posts occur here as well). So, if we wanted to iterate over all posts that this account has made, we could use the following code:
from steem import Steem
from steem.post import Post
account = "steempytutorials"
for post in steem.get_blog(account, 0, 500):
post = Post(post["comment"])
# Make sure it's a main post and also not a resteemed post
if post.is_main_post() and post["author"] == account:
# DO SOMETHNIG
Finding out who resteemed a post
To find out who resteemed a post is really simple as well. To do this we can use the aptly named get_reblogged_by()
function that takes an account's name and a permlink as arguments and returns a list of account names that resteemed the given post. So for each post in an account's history we simply need to extract the permlink and pass this to the function alongside the account's name. For some reason posting something counts as resteeming something, so we need to make sure to remove our own account's name from the list of people that resteemed the post as well. After doing this we can just extend our list of resteemers with this list! To do this we can use the following code
resteemers = []
for post in steem.get_blog(account, 0, 500):
post = Post(post["comment"])
if post.is_main_post() and post["author"] == account:
permlink = post["permlink"]
resteemed_by = steem.get_reblogged_by(account, permlink)
if not resteemed_by == []:
resteemed_by.remove(account)
resteemers.extend(resteemed_by)
print(resteemers)
which gives a huge list for @steempytutorials, so I'll show the output for my account @amosbastian instead
['munaa', 'cryptonewstoday', 'gazuua', 'vot', 'xnpbgsw', 'resteemsupport', 'datascience', 'jaronmct', 'juliank']
What if the account has > 500 posts?
The function get_blog()
can only get a maximum of 500 posts, so if an account has more than 500, then you need to do it in batches. To do this we can simply create a while loop that keeps iterating over posts while the posts analysed are less than the account's total posts. We can do this with the following code
post_limit = 0
while post_limit < Account(account)["post_count"]:
for post in steem.get_blog(account, post_limit, 500):
# DO STUFF
post_limit += 500
To test this I found someone I follow with more than 500 posts, @jestemkioskiem, and used him as an example. This gives us the following output
['tiotioahmad', 'avenal', 'ceybiicien', 'fitzgibbon', 'j4nke', 'promo-steem', 'simon-paluch', 'dufriadi', 'wens', 'martwykotek', 'pf-coin', 'shello', 'tiotioahmad', 'avenal', 'ceybiicien', 'fitzgibbon', 'j4nke', 'promo-steem', 'simon-paluch', 'dufriadi', 'wens', 'martwykotek', 'pf-coin', 'shello']
Creating and printing the top 10
An easy way to do this is to use a Counter
from the collections
package. We can create a Counter
of our resteemers
list and use the following code to print our top 10
for resteemer in Counter(resteemers).most_common(10):
print("{0:16} has resteemed {1:16} {2:>2} times!".format(
resteemer[0], account, resteemer[1]))
which for @steempytutorials gives the following
amosbastian has resteemed steempytutorials 30 times!
muksal.mina has resteemed steempytutorials 8 times!
photo-trail has resteemed steempytutorials 5 times!
juliank has resteemed steempytutorials 3 times!
isnamahyazani has resteemed steempytutorials 2 times!
pinay has resteemed steempytutorials 2 times!
vot has resteemed steempytutorials 2 times!
cryptoryno33 has resteemed steempytutorials 1 times!
davidamsterdam has resteemed steempytutorials 1 times!
abu-banderas has resteemed steempytutorials 1 times!
Note: if an account has a lot of posts then this can really take a while. I tested this with @juliank's account as well, at it took forever, but it does work as seen below
sun-flower has resteemed juliank 602 times!
pixresteemer has resteemed juliank 348 times!
jaladin717 has resteemed juliank 312 times!
devasish has resteemed juliank 300 times!
nasional has resteemed juliank 180 times!
deerjay has resteemed juliank 180 times!
photo-trail has resteemed juliank 169 times!
fesbukan has resteemed juliank 144 times!
janellelanae has resteemed juliank 144 times!
steemsyndicate has resteemed juliank 132 times!
Congratulations, you've now learned how iterate over all blog posts by an account using steem-python
, how to get a list of people that resteemed each post and print an account's top 10 resteemers!
Curriculum
Set up:
- Part 0: How To Install Steem-python, The Official Steem Library For Python
- Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
Filtering
- Part 2: How To Stream And Filter The Blockchain Using Steem-Python
- Part 6: How To Automatically Reply To Mentions Using Steem-Python
Voting
- Part 3: Creating A Dynamic Autovoter That Runs 24/7
- Part 4: How To Follow A Voting Trail Using Steem-Python
- Part 8: How To Create Your Own Upvote Bot Using Steem-Python
- Part 17: 3 Methods To Prevent Double Voting On Posts
Posting
- Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
- Part 7: How To Schedule Posts And Manually Upvote Posts For A Variable Voting Weight With Steem-Python
Constructing
Rewards
- Part 9: How To Calculate A Post's Total Rewards Using Steem-Python
- Part 12: How To Estimate Curation Rewards Using Steem-Python
- Part 14: How To Estimate All Rewards In Last N Days Using Steem-Python
Transfers
- Part 11: How To Build A List Of Transfers And Broadcast These In One Transaction With Steem-Python
- Part 13: Upvote Posts In Batches Based On Current Voting Power With Steem-Python
Account Analysis
- Part 15: How To Check If An Account Is Following Back And Retrieve Mutual Followers/Following Between Two Accounts
- Part 16: How To Analyse A User's Vote History In A Specific Time Period Using Steem-Python
The code for this tutorial can be found on GitHub!
This tutorial was written by @amosbastian in conjunction with @juliank.
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x