Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
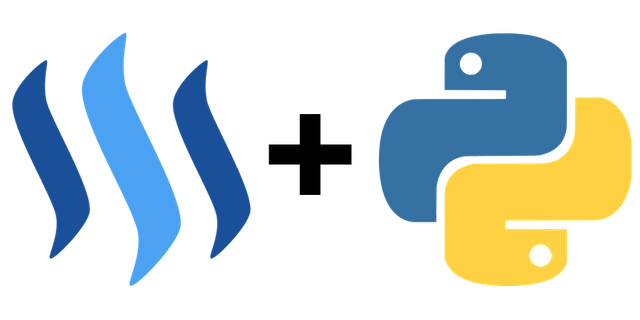
This tutorial is part of a series where we explain different aspects of programming with steem-python
. Links to the other tutorials can be found below. You will learn how steem-python
can be used to post an article from file directly to the Steem blockchain as well as built and broadcast a transaction to purchase an upvote for the article posterd.
What will I learn
- What submitting a post looks like in
steem-python
- Creating an unique permlink
- How a transaction is built
- How to broadcast a transaction
Requirements
- Python 3.6
steem-python
Difficulty
- Basic
Tutorial
Set up
Start by downloading both files from github. The post.txt
is used to store the post we want to submit and the title and tags we would like to use. The first line is used for the title and the second line is for the tags, separated by a whitespace. The rest of the file is the body of the post.
#1 Title 'Test' Buy Upvote
#2 blog test steemdev
#3 <center>
#4 Test buying upvote from minnowbooster
#5 </center>
In scheduler.py
you will have to set the author, the upvote bot you want to use and what amount in SBD you want to buy upvotes for.
author = 'sttest2'
upvote_bot = 'minnowbooster'
amount = 0.05
What does submitting a post look like?
Submitting a post with steem-python
requires several variables. Most of them are optional. We will be using the title, body, author, permlink and self_vote.
def post(self,
title,
body,
author,
permlink=None,
reply_identifier=None,
json_metadata=None,
comment_options=None,
community=None,
tags=None,
beneficiaries=None,
self_vote=False):
Calling the post function to submit the post then looks like:
steem.post(title, body, author, permlink, None, None, None, None, tags, None, True)
Permlink
The permlink is an unique identifier for each post, which is linked to the author of the post. Leaving this blank steem-python
will automatically assign a permlink based on the title. However, the permlink is needed to buy an upvote, therefor a permlink needs be to created from the title. To make sure the permlink created is unique and valid all characters except for letters and numbers will be removed, as well as capitals. A random number and a timestamp will be added as well.
permlink_title = ''.join(e for e in title if e.isalnum()).lower()
permlink = "{}-%s%s".format(permlink_title) % (time.strftime("%Y%m%d%H%M%S"), random.randrange(0,9999,1))
How is a transaction built?
A transaction is built up by a sender, receiver, amount in SBD/STEEM and an optional memo in a dict. For sender the author will b used and the receiver will be the upvote_bot. SBD will be used and the memo will be full url to the article, including permlink and author.
transfers =[{
'from': author,
'to': upvote_bot,
'amount': 'amount SBD',
'memo': 'full url to post'
}]
The full url to the post can be created with the permlink and author as follows:
'https://steemit.com/@{}/{}'.format(author, permlink)
The next code is then used to sign and broadcast the transaction
tb = Transactionbuilter()
operation = [operations.Transfer(**x) for x in transfers]
tb.appendOps(operation)
tb.appendSigner(author, 'active')
tb.sign()
tx = tb.broadcast()
Note: transfers
is a list with transactions. Each transaction is put in a dict. This allows for multiple transactions to be broadcasted at once. Like for example if you wish to purchase multiple upvotes from several upvote bots.
Running the code
Now that you have configured the code to your own liking you can test it for yourself.
python scheduler.py
Submitted post
Buying vote succes
Curriculum
- Part 0: How To Install Steem-python, The Official Steem Library For Python
- Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
- Part 2: How To Stream And Filter The Blockchain Using Steem-Python
- Part 3: Creating A Dynamic Upvote Bot That Runs 24/7
- Part 4: How To Follow A Voting Trail Using Steem-Python
Credits
This tutorial was written by @juliank in conjunction with @amosbastian
The code for this tutorial can be found on GitHub!
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Much appreciated!
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Good post, I am a photographer, it passes for my blog and sees my content, I hope that it should be of your taste :D greetings
iss it right
@steempytutorials,
How will I specify the POSTING Key of the steem user? To auto post the article on a certain schedule.
This is a great article, I wonder if its still current with the latest Steem hard forks. But definetly a great post.