Part 6: How To Automatically Reply To Mentions Using Steem-Python
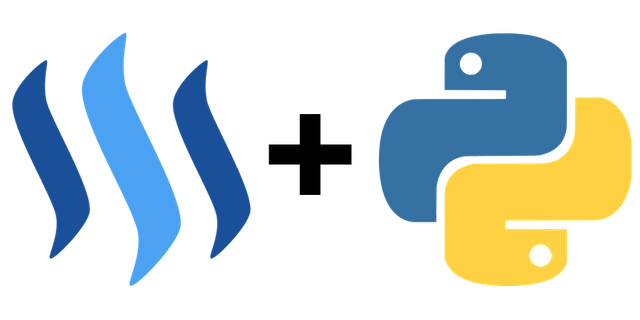
This tutorial is part of a series where we explain different aspects of programming with steem-python
. Links to the other tutorials can be found in the curriculum section below. Today we will learn how to stream the blockchain and see who mentions you using steem-python
!
What will I learn
- How to create a regular expression
- How to find people mentioning you
- How to reply to them
Requirements
- Python3.6
steem-python
re
Difficulty
- Basic
Tutorial
Streaming the blockchain for mentions
We have already gone over how to stream the blockchain in part 2 of this tutorial series, so if you need to, read up about it there. In the previous tutorials we could simply filter the blockchain for the operations we wanted, for example posts or votes, but unfortunately mentions aren't an operation on the blockchain. What this means is that the best way to find out who mentions you is to stream all posts on the blockchain and then check its body to see if someone has mentioned you. So once again, we simply stream all posts on the blockchain like so
from steem import Steem
from steem.blockchain import Blockchain
from steem.post import Post
steem = Steem()
blockchain = Blockchain()
stream = map(Post, blockchain.stream(filter_by=["comment"]))
for post in stream:
# DO SOMETHING
Creating a regular expression
To find out who mentions us we should read the body of each post and find out if it contains @username
. A great way to do this is by using a regular expression. We can use a regular expression to specify the rules for the string that we want to match, a mention in this case. Luckily a good regex to do this can be found here
(?<=^|(?<=[^a-zA-Z0-9-_\.]))@([A-Za-z]+[A-Za-z0-9]+)
We can then use the function findall()
to create a list of all mentions in a post. Once we have this we can simply check if our username is in the given list! An example is given below
text = "hello @amosbastian and @juliank I love @steempytutorials, contact me at [email protected]"
mentions = re.findall("(?<=^|(?<=[^a-zA-Z0-9-_\.]))@([A-Za-z]+[A-Za-z0-9]+)", text)
print(mentions)
which outputs
['amosbastian', 'juliank', 'steempytutorials']
Mentions on the blockchain
So now we know how to find mentions in a string we can use this to find mentions in the body of posts. To do this we can use the regex we created above and do the following
REGEX = "(?<=^|(?<=[^a-zA-Z0-9-_\.]))@([A-Za-z]+[A-Za-z0-9]+)"
username = "steempytutorials"
while True:
try:
for post in stream:
mentions = re.findall(REGEX, post["body"])
if username in mentions:
print(f"{post['author']} just mentioned you!")
except Exception as error:
print(repr(error))
continue
Running this program and testing it showed the following
$ python mentions.py
amosbastian just mentioned you!
Replying to mentions
If someone mentions you, you could do many things, but for the sake of this tutorial we are simply going to reply to them. To this we can use the reply()
function, which makes replying to a post very simple! Instead of printing the post we will change it to
post.reply(f"Hey @{post['author']} thanks for mentioning me!")
and indeed, it works as expected
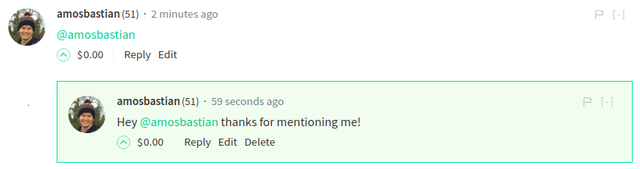
Congratulations, you can now automatically reply to anyone who mentions you using steem-python
!
Curriculum
- Part 0: How To Install Steem-python, The Official Steem Library For Python
- Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
- Part 2: How To Stream And Filter The Blockchain Using Steem-Python
- Part 3: Creating A Dynamic Upvote Bot That Runs 24/7
- Part 4: How To Follow A Voting Trail Using Steem-Python
- Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
The code for this tutorial can be found on GitHub!
This tutorial was written by @amosbastian in conjunction with @juliank.
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
Btw, I have a couple of ideas about this post:
Node issue
stream = map(Post, blockchain.stream(filter_by=["comment"]))
This shouldn't work since default node defined at steem-python is steemd.steemit.com and it's down for a while. So, if you make it compatible with the jussi or another public node for the potential readers trying out the code, that would be perfect.
Mentions
We can use
post.get("json_metadata", {}).get("users")
to get mentions. I am not sure twitter username regex has a full compliance with steemit blockchain's username restrictions. Are there any specific reason to use a regex pattern?Great job on the series! Looking forward to see more.
You can contact us on Discord.
[utopian-moderator]
Thanks! Regarding the node issue, in part 1 of the series @juliank made sure to mention the default node was deprecated and to set a new one using
steempy set nodes https://rpc.steemviz.com
for example. About the mentions, I never realised that! Funny thing is my mind jumped to regex, because I think I recalled that you used it for the mentions on steem.rocks. Guess I learned something today as well, so thanks for that! 😄Yeah, actually I wasn't aware of that and the regex pattern is still in place at steem.rocks. @favcau warned me lately that info can be obtained at json_metadata. :)
Sorry about the node issue, couldn't have time to read the whole series yet.
Haha, no worries, I had to go to part 1 myself and check if @juliank had actually mentioned it.
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
I'm wondering about the line:
When I look at the github source it's defined on line 13 yet it is never used.
Can that line just be removed?
Also I'm trying to figure out the node error issue. I was able to solve in on another one of your tutorial by passing my keys into the Steem object:
I'm doing the same with this example but I still get an error about the nodes:
Do I have to pass the nodes array into the
blockchain
object too?I'm a little further now but I keep getting:
When the script runs
post.reply
.I managed to fix it by specifying my username in the post function: