Part 9: How To Calculate A Post's Total Rewards Using Steem-Python
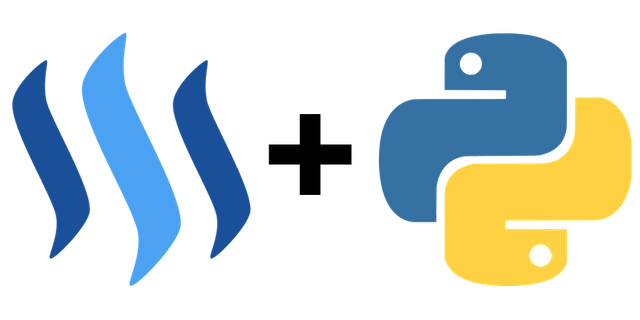
This tutorial is part of a series where we explain different aspects of programming with steem-python
. Links to the other tutorials can be found in the curriculum section below. Today we will learn how to calculate the rewards of a post!
What will I learn
- What the reward fund looks like
- How to calculate a post's reward share
- How to calculate a posts actual reward
Requirements
- Python3.6
steem-python
Difficulty
- Basic
Tutorial
The reward fund
The way rewards are distributed on Steemit is by calculating the total reward shares of a post and using this to calculate how much a post should be rewarded. To find out all this information steem-python
provides a very handy function called get_reward_fund()
which gives us the following information
{
"id": 0,
"name": "post",
"reward_balance": "717950.323 STEEM",
"recent_claims": "373489696650140999",
"last_update": "2018-01-20T15:33:27",
"content_constant": "2000000000000",
"percent_curation_rewards": 2500,
"percent_content_rewards": 10000,
"author_reward_curve": "linear",
"curation_reward_curve": "square_root"
}
were we can see some useful information. Here reward_balance
is the total reward pool and recent_claims
is the amount of recent claims. We can use this to calculate how much one share of the reward balance is worth, like so
from steem import Steem
from steem.amount import Amount
steem = Steem()
reward_fund = steem.get_reward_fund()
reward_balance = Amount(reward_fund["reward_balance"]).amount
recent_claims = float(reward_fund["recent_claims"])
reward_share = reward_balance / recent_claims
print(f"One reward share is currently worth: {reward_share}")
where we use Amount
to automatically convert 707222.264 STEEM
to an actual number. This code outputs the following:
One reward share is currently worth: 1.922662039408618e-12
This currently doesn't mean that much to us, but in the next section it will become clear what we can use this for.
Calculating reward shares of a post
To calculate the total reward shares of a post we need to look at all the total reward shares of the votes on that post. You should recall that in part 2 of this tutorial series we looked at what a post actually looks like. A post contains a list called active_votes
with all the currently active votes on it, which all look something like this
{
"voter": "juliank",
"weight": 203149,
"rshares": "130300902876",
"percent": 1000,
"reputation": "65384949253746",
"time": "2018-01-12T17:27:42"
}
As you can see each vote in active_votes
has an attribute called "rshares". We can use this to calculate the total reward shares, or rshares
, of a post with the following code
post = Post("@steempytutorials/part-8-how-to-create-your-own-upvote-bot-using-steem-python")
total_rshares = 0
for vote in post["active_votes"]:
total_rshares += float(vote["rshares"])
print(f"The total reward shares of this post are: {total_rshares:.2f}")
which currently gives the following output
The total reward shares of this post are: 1734177616928.00
Calculating the actual reward of a post
Now we have the total reward shares of a post we should be able to simply multiply it with how much one reward share is worth, right? Let's see what this gives us
print(f"The post is worth ${total_rshares * reward_share:.2f}")
which outputs
The post is worth $3.33
Wait a minute... that doesn't seem right. Checking the post on Steemit shows a completely different value, so what's going wrong? The problem is that we still need to multiply this with the average STEEM/SBD price to get the actual value of the post. Thankfully we can find out this price by using the get_current_median_history_price()
function. This currently returns something like this:
{
"base": "4.323 SBD",
"quote": "1.000 STEEM"
}
The base price is what we need! We can simply take this and then multiply it with our total reward shares to get the actual reward of the post
base = Amount(steem.get_current_median_history_price()["base"]).amount
print(f"The post is actually worth ${total_rshares * reward_share * base:.2f}")
which outputs
The post is actually worth $14.42
Indeed, going to the post on Steemit shows that this is (roughly) the correct reward! Congratulations, you now know how to calculate the rewards of a post using steem-python
!
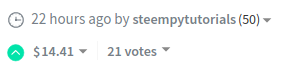
Curriculum
- Part 0: How To Install Steem-python, The Official Steem Library For Python
- Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
- Part 2: How To Stream And Filter The Blockchain Using Steem-Python
- Part 3: Creating A Dynamic Autovoter That Runs 24/7
- Part 4: How To Follow A Voting Trail Using Steem-Python
- Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
- Part 6: How To Automatically Reply To Mentions Using Steem-Python
- Part 7: How To Schedule Posts And Manually Upvote Posts For A Variable Voting Weight With Steem-Python
- Part 8: How To Create Your Own Upvote Bot Using Steem-Python
The code for this tutorial can be found on GitHub!
This tutorial was written by @amosbastian in conjunction with @juliank.
Posted on Utopian.io - Rewarding Open Source Contributors
Probably silly question, from someone new to Python, how come we can't use the same code for 'recent_claims' that we used for 'reward_balance' - code in second line:
It's because
Amount
is a class insteem-python
that splits a string like "10 SBD" intoamount
(10) andasset
(SBD) as you can see here. Thefloat()
function just converts a number or a string into a float! If you really wanted you could dorecent_claims = float(reward_fund["reward_balance"].split()[0])
instead, but it's easier to just useAmount
class.I see, thank you!
This example here shows better what is going on, nice, now I have two options!
There are no silly questions! I will let @amosbastian tackle this one though as he wrote this tutorial
This is great stuff, so hard to find good explicative content ! Please keep it up !
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x