Part 14: How To Estimate All Rewards In Last N Days Using Steem-Python
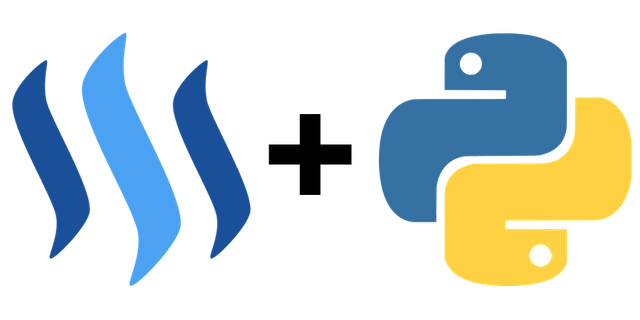
This tutorial is part of a series where we explain different aspects of programming with steem-python
. Links to the other tutorials can be found in the curriculum section below. In part 9 of this series we learned how to calculate the total rewards of a post and in part 12 we learned how to calculate curation rewards of a post. In this tutorial we will learn how to put this all together and estimate all rewards of posts made by a specific account in the last N
days!
What will I learn
- How to calculate beneficiary shares
- How to calculate author rewards and beneficiary rewards
- How to calculate rewards in the last
N
days
Requirements
- Python3.6
steem-python
Difficulty
- Intermediate
Tutorial
If you find it easier to follow along this way you can get the entire code for this tutorial from here, where I have split everything up into the relevant sections.
Calculating beneficiary shares
Something most people might not have realised is that Steemit has a thing called beneficiaries, where you can specify others that will get a part of your author rewards. For example, when posting via Utopian.io the utopian.pay account is set as a beneficiary and receives 25% percent of your author rewards. This looks like this
"beneficiaries": [
{
"account": "utopian.pay",
"weight": 2500
}
]
We need to use this information to get the percentage of our author rewards that goes to our benificiaries! To do this we can simply sum the weight of each beneficiary and divide by 10000
, like so
def beneficiaries_pct(post):
weight = sum([beneficiary["weight"] for beneficiary in post["beneficiaries"]])
return weight / 10000.0
which in this case would give 0.25
!
Calculating author and beneficiary rewards
Now that we can calculate the percentage of the author rewards that goes to beneficiaries we can easily calculate the author rewards. In part 9 we already calculated the total reward of a post, so to get the author reward we need to do the following:
- Subtract the curation reward calculated in part 12
- Multiply this by (1.0 - beneficiaries' percentage)
To do this we can use the code from part 9 and part 12
# Everything we need to calculate the reward
reward_fund = steem.get_reward_fund()
reward_balance = Amount(reward_fund["reward_balance"]).amount
recent_claims = float(reward_fund["recent_claims"])
reward_share = reward_balance / recent_claims
base = Amount(steem.get_current_median_history_price()["base"]).amount
votes = [vote for vote in post["active_votes"]]
### Curation reward penalty
def curation_penalty(post, vote):
post_time = post["created"]
vote_time = parse(vote["time"])
time_elapsed = vote_time - post_time
reward = time_elapsed / timedelta(minutes=30) * 1.0
if reward > 1.0:
reward = 1.0
return reward
### Calculating curation reward per vote
curation_pct = 0.25
def curation_reward(post, vote):
rshares = float(vote["rshares"])
base_share = reward_share * base
return (rshares * curation_penalty(post, vote) * curation_pct) * base_share
and create a new estimate_rewards(post)
function that looks like this
### Adding everything together
def estimate_rewards(post):
votes = [vote for vote in post["active_votes"]]
total_share = sum([float(vote["rshares"]) * reward_share * base for vote in votes])
curation_share = sum([curation_reward(post, vote) for vote in votes])
author_share = (total_share - curation_share) * (1.0 - beneficiaries_pct(post))
beneficiary_share = (total_share - curation_share) * beneficiaries_pct(post)
print(f"Estimated total reward for this post is ${total_share:.2f}")
print(f"Estimated author reward for this post is ${author_share:.2f}")
print(f"Estimated beneficiary reward for this post is ${beneficiary_share:.2f}")
print(f"Estimated curation reward for this post is ${curation_share:.2f}\n")
which outputs the following
Estimated total reward for this post is $29.46
Estimated author reward for this post is $17.22
Estimated beneficiary reward for this post is $5.74
Estimated curation reward for this post is $6.51
Estimating rewards in last N
days
Alright, so now we can calculate every single reward for a post, we can use this to calculate this for every post in the last N
days. To do this we need to do a few things; we need to set this time period somehow and iterate over all posts in our account's history. Setting the time period is pretty simple and can be done using timedelta()
. If we want to set this period to three days, then we could do the following
time_period = datetime.timedelta(days=3)
Iterating over all posts in an account's history can be done just like streaming the blockchain by using the history_reverse()
. As an example I will use the estimate_rewards()
for each post @steempytutorials made in the last three days
for post in Account(account).history_reverse(filter_by="comment"):
try:
post = Post(post)
# Check if post isn't too old
if post.time_elapsed() < time_period:
# Make sure post hasn't already been seen
if post.is_main_post() and not post["title"] in posts:
posts.add(post["title"])
print(post["title"])
estimate_rewards(post)
else:
break
except Exception as error:
continue
where we add each post's title to a set (an unordered collection with no duplicate elements), because when someone edits a post it counts as its own separate post, and we don't want to estimate rewards for the same post multiple times. Running this outputs the following at the time of writing this post
part 12: Upvote Posts In Batches Based On Current Voting Power With Steem-Python
Estimated total reward for this post is $23.18
Estimated author reward for this post is $13.67
Estimated beneficiary reward for this post is $4.56
Estimated curation reward for this post is $4.95
Daily Steem-Python Challenge #12, Win 1 Steem!
Estimated total reward for this post is $3.78
Estimated author reward for this post is $3.70
Estimated beneficiary reward for this post is $0.00
Estimated curation reward for this post is $0.08
Part 12: How To Estimate Curation Rewards Using Steem-Python
Estimated total reward for this post is $29.43
Estimated author reward for this post is $17.19
Estimated beneficiary reward for this post is $5.73
Estimated curation reward for this post is $6.50
Congratulations, you've now learned how to estimate each type of reward of a post using steem-python
. You have also learned how to iterate over an account's post history and print each post's estimated rewards!
Curriculum
- Part 9: How To Calculate A Post's Total Rewards Using Steem-Python
- Part 12: How To Estimate Curation Rewards Using Steem-Python
The code for this tutorial can be found on GitHub!
This tutorial was written by @amosbastian in conjunction with @juliank.
Posted on Utopian.io - Rewarding Open Source Contributors
I shamelessly plug here a little promotion for my python-based ongoing contest for you Python lovers. Best bot to play the Public Goods game wins the pot
I will definitely check it out!
What OS are you using for this steem-python library?
I tried it on my Windows 10 laptop but it doesn't work.
Is steem-python limited to Linux OS? or is there something I'm missing in my installation?
Personally I'm using Ubuntu and I think @juliank uses a Mac. You need Python3.6 to install
steem-python
, but you also needscrypt
, which is only available on Windows for Python3.5, so that's a really annoying problem... I will see if I can figure something out and get back to you!I'm using an Anaconda with python 3.5. This is an example of an error I encounter on using its functionalities.
Have you encountered something like this?
First things first, you need Python3.6 for
steem-python
. Also it's a lot easier for me to help you if you join our Discord!I use a mac os indeed for testing, but I run all the script from a server. You can get yourself a droplet at digital ocean for $5 a month. You can then mount the droplet drive this to your own pc and basically work on your own pc and run the files via a terminal on the droplet
$5 is expensive for a beginner like me. My goal for now is to learn how to use steem python library.
Thanks for your suggestion. I'll definitely try digital ocean when I have enough knowledge for automated script.
good post, I like your post ..
I need your support please visit my blog https://steemit.com/@muliadi
if you like my post please give upvote, resteem &follow me.
thank you, keep on steemit.
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x