Part 8: How To Create Your Own Upvote Bot Using Steem-Python
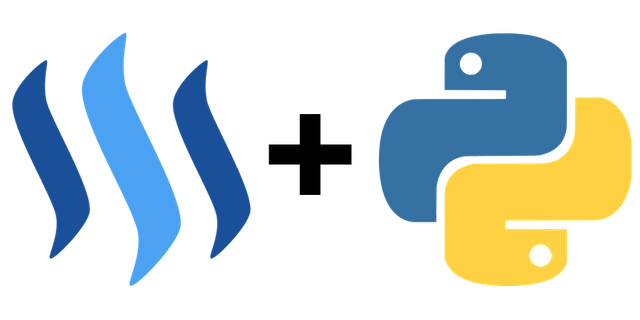
This tutorial is part of a series where we explain different aspects of programming with steem-python
. Links to the other tutorials can be found in the curriculum section below. Today we will learn how to stream the blockchain for transfers directed towards us and then perform actions based on its content!
What will I learn
- How to filter the blockchain for transfers
- What a transfer looks like
- How to handle the transfer
Requirements
- Python3.6
steem-python
Difficulty
- Basic
Tutorial
Streaming the blockchain for transfers
We have gone over this in previous tutorials, so you should know how to do this by heart! We simply set the operation to filter the blockchain by to transfers
, like so
from steem import Steem
from steem.blockchain import Blockchain
steem = Steem()
blockchain = Blockchain()
stream = blockchain.stream(filter_by=["transfer"])
for transfer in stream:
# DO SOMETHING
What does a transfer on the blockchain look like?
Before we can implement our program that replies to incoming transfers we need to know what a transfer actually looks like. Printing a transfer out gives the following example
{
"from": "ginabot",
"to": "elnazry",
"amount": "0.001 STEEM",
"memo": "Your account has been validated!",
"_id": "b2a57b62afa8b1a86bb7d42389e774cdd6949a75",
"type": "transfer",
"timestamp": "2018-01-17 14:59:39",
"block_num": 19059815,
"trx_id": "1af0c84531381911be9a6570455e881bcfa3b2fd"
}
As you can see there's a lot of useful information, but we are only interested in who sent the transfer, to whom the transfer was sent, what the amount is and what the memo is.
Checking the transfer
The first step when streaming transfers on the blockchain is to make sure the transfer is actually meant for us. To do this we can simply check every "to" key of each transfer and see if it matches our username, then print some information about the transfer, like so
for transfer in stream:
if transfer["to"] == username:
print(f"{transfer['from']} {transfer['amount']} {transfer['memo']}")
After testing it with another account it printed the following
$ python memo.py
amosbastian 0.001 SBD hi, this is a test!
and checking it on Steemit also shows it works

Upvoting the post in the memo
Since we are creating an upvote bot we should upvote whatever the URL in the memo is (if it's a valid Steemit URL of course). So how can we do this? First we need to extract the URL from the memo and make sure it's a valid Steemit URL. After doing this we need to upvote the given post. Seems pretty easy, right? We can use the following code to accomplish this
username = "amosbastian"
for transfer in stream:
if transfer["to"] == username:
url, permlink = transfer["memo"].split("@")
if "https://steemit.com/" in url:
steem.vote(f"@{permlink}", 100)
and indeed, after testing it with another account (made available to me via @juliank), it works as expected!

Currently we don't check the amount of the transfer (and our URL checking could fail), but that seemed beyong the scope of this tutorial. If you want to implement that yourself, then go ahead, but otherwise congratulations, you have created your very first upvote bot!
Curriculum
- Part 0: How To Install Steem-python, The Official Steem Library For Python
- Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
- Part 2: How To Stream And Filter The Blockchain Using Steem-Python
- Part 3: Creating A Dynamic Autovoter That Runs 24/7
- Part 4: How To Follow A Voting Trail Using Steem-Python
- Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
- Part 6: How To Automatically Reply To Mentions Using Steem-Python
- Part 7: How To Schedule Posts And Manually Upvote Posts For A Variable Voting Weight With Steem-Python
The code for this tutorial can be found on GitHub!
This tutorial was written by @amosbastian in conjunction with @juliank.
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hi bro, would you mind visiting my last post? I am looking for coders to work with. Is Juliank a coder too?
Hi, just checked it out, looks very cool! We are both coders, we actually met at university studying computer science. What can we help you with?
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x