Part 4: How To Follow A Voting Trail Using Steem-Python
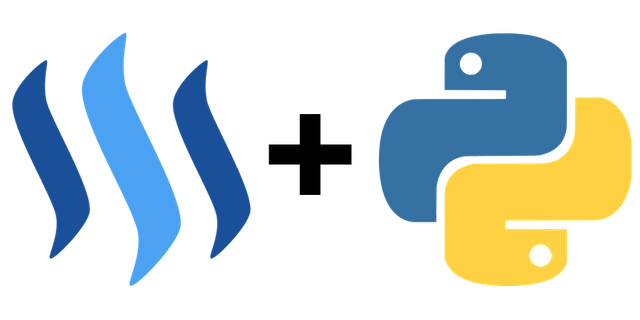
This tutorial is a continuation of Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python and Part 2: How To Stream And Filter The Blockchain Using Steem-Python, so make sure to read it before starting on this tutorial. Today we will use the knowledge we acquired from those tutorials to create a program that follows a voting trail!
What will I learn
- How to filter the blockchain for votes
- What a vote looks like in
steem-python
- How to following a voting trail
- How to make sure you don't follow votes on comments
Requirements
- Python3.6
steem-python
Difficulty
- Basic
Tutorial
Filtering the blockchain for votes
In part 2 of this tutorial series we learned how to filter the blockchain for comments. This time we will be filtering it for votes instead. As you should recall we used the stream()
function to stream the blockchain. So how can we stream votes instead? To do this we can use the filter_by
argument, which is a list of operations we want to filter the blockchain by. So what kind of operations, besides a comment, can we filter the blockchain by? All operations that we can filter by can be found here, and as you can see "vote" is one of them. This means we can simply stream all votes on the blockchain with the following code
from steem import Steem
from steem.blockchain import Blockchain
steem = Steem()
blockchain = Blockchain()
stream = blockchain.stream(filter_by=["vote"])
while True:
try:
for vote in stream:
# DO SOMETHING
except Exception as error:
continue
What does a vote look like?
Before we can do anything with the votes we are streaming we need to know what a vote actually looks like. An example of a printed vote is given below
{
"voter": "badfatcat",
"author": "claudene",
"permlink": "update-the-new-coins-in-the-philippines-the-p10-pesos-and-p5-pesos-2018-20171231t62751604z",
"weight": 10000,
"_id": "1af544c59ea75a816ed756578105d9befa442224",
"type": "vote",
"timestamp": "2018-01-15 14:32:33",
"block_num": 19001685,
"trx_id": "1d0490ac6aea885b373a5dc0f1bebb22d9f9fd96"
}
which includes some useful information about who the voter is, who the author is and what the permalink of the post he voted on is. To get this information you can simply do
voter = vote["voter"]
author = vote["author"]
permlink = vote["permlink"]
Following the voting trail
So now we can filter the blockchain on votes we want to make sure our program votes on the same posts as some of our favourite users. To do this we can simply create a list of users whose vote we want to follow and then use this to find the correct votes! We already learned how to upvote in part 1 of this tutorial series, so that shouldn't be a problem anymore! Putting this all together results in the following code
voting_trail = ["juliank"]
for vote in stream:
voter = vote["voter"]
author = vote["author"]
permlink = vote["permlink"]
if voter in voting_trail:
print("Voting on {} post that {} voted on!".format(permlink,
voter))
steem.vote(template.format(author, permlink), 100)
which outputs the following
Voting on re-juliank-what-is-beter-than-1-person-jumping-20180115t144010042z post that juliank voted on!
and indeed, I voted on the same post that @juliank upvoted, as seen below

Making sure it's not a comment
To do this we simply need to use what we learned in part 2 of this tutorial series and make sure the post is not a comment with the function is_main_post()
! We can simply change some of the code from above to be the following
post = template.format(author, permlink)
if Post(post).is_main_post():
print("Voting on {} post that {} voted on!".format(permlink,
voter))
steem.vote(post, 100)
Congratulations! You now know how to filter the blockchain for votes and upvote all posts (not comments!) that your favourite users vote on automatically!
Curriculum
Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
Part 2: How To Stream And Filter The Blockchain Using Steem-Python
Credits
This tutorial was written by @amosbastian in conjunction with @juliank
The code for this tutorial can be found on GitHub!
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thanks!
@steempytutorials great tutorial. Keep up the good work. As I am learning python, looking forward to follow your tutorials here to learn steem-python library. Happy coding
Love to hear this, have fun. We will keep more content coming
Ok @jukiank me also looking forward to contribute on open source project s
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Awesome tutorials - how that looks if I want to add the limitation of predefined categories.... so only trail people in categories X,Y,Z?
Would be great to get a hint :)