[Termux & Nodejs] Build A Discord Bot in Android - Part-1
Repository
https://github.com/kibriakk/discordbot
What Will You Learn?
- How to install termux in android
- How to install nodejs in android
- How to create a Discord bot by android
Requirements
- Android
- Termux
- Nodejs
- Discord Id
- Browser
Difficulty
- Basic
Tutorial Contents
STEP-1 : Install Termux on Android
At first download termux from google playstore which is free to use . You can search it in google playstore or download it from this link
initially it doesn’t require root access.
Now open termux , it will show you a welcome screen. Press OK.
To install nodejs we need to setup some initial staffs first.
First command to write :
apt update && apt upgrade
and press enter in our keyboard. This doesn’t update the software or apt version but download lists of all available repository
Now setup storage and allow storage permission
termux-setup-storage
Now we will need a text editor which you can install using command in termux.
There are many text editor available for termux. but we will work with 'nano'
Write this command
apt install nano
Now let’s make a file using termux
Write this command
touch new.js
This will create a file named "new.js" but where we can see the file ?
You can see the file if you have root access.
Open a file explorer and go to root of the storage. Then data/data/com.termux/files/home. But in this case you will need root access.
If you dont have root access , its OK to forget it .
Now we edit the file using text editor
Write this command and hit enter
nano new.js
You can see that a text editor open
Now write any javascript code here and save.
For example I write this code
console.log("Hi kibria365.Java script is working fine.");
Now save with CTRL+X
STEP-2: Install Node.js in Termux
Nodejs is nessesary for running javascript file in android.So now we are going to install nodejs in termux.
First Open Termux and write this command and hit "Enter".
pkg install nodejs
or apt install nodejs
It will ask you to press y/n , press y and enter so it will get permission to install nodejs.
Now additionally we can install express to have good package manager.
Write This command
npm init
And press enter. Now you will get some options to write information about your project. You can leave them blank by pressing enter aswell. Finally npm is initilized.
Now write :
npm install express --save
And press enter.
Finally Nodejs is installed.
You should check it before going the next step.
Do you remember we made a new.js file in previous step. Now we will check our installed nodejs by run this file.
Write this command and press Enter.
node new.js
You will see a message like this.
"Hi kibria365.Java script is working fine."
If you get any error comment on this post. I am available for help anytime.
STEP-3 : Create a discord Bot and add it on discord server.
Go to the link .
Your account should be logged in, so you’ll go straight to your account’s list of applications. Hit “New Application” to get started. Give the bot a name, then hit the button marked “Save Changes.”
Now, on the right-hand menu, click “Bot.” Once in the new menu, click “Add Bot” under the Build-a-bot option. If you only have one application — the one we just made — it should appear automatically. Otherwise, select it.
Then write bot username, select bot permission and upload profile picture.Copy The token. and keep it safe place.
Now we add this bot to our discord server. Login to your discord app.Create a server.
Then we need to generate a link.
So go to the application tab and open the “App Details” and find your “Client ID,” a long number. Copy the number and add it to this URL, in the place of word CLIENTID.
https://discordapp.com/oauth2/authorize?&client_id=CLIENTID&scope=bot&permissions=8
Now Enter into the link.That’ll take you to a website where you can tell Discord where to send your bot. Select your server name and press join bot. You will get a notification in your Discord server.
STEP-4 : Install node modules / packages for discord bot.
Open Termux and write the following command
npm install discord.io
Now press Enter.
discord.io node module will be installed.
Step-5 : Create a sample Bot
Create a new file with following command & hit Enter.
touch bot.js
Then Edit the "bot.js" file with text editor.
nano bot.js
Now write the following code one by one.
/*Variable area*/
var Discord = require('discord.io');
var bot = new Discord.Client({
token: "", // Write your Bot Token in ""
autorun: true
});
/*Event area*/
bot.on("ready", function(event) {
console.log("Connected!");
console.log("Logged in as: ");
console.log(bot.username + " - (" + bot.id + ")");
});
bot.on("message", function(user, userID, channelID, message, event) {
console.log(user + " - " + userID);
console.log("in " + channelID);
console.log(message);
console.log("----------");
Message function.
if (message === "ping") {
sendMessages(channelID, ["Pong"]); //Sending a message with our helper function
} else if (message === "picture") {
sendFiles(channelID, ["fillsquare.png"]); //Sending a file with our helper function
}
});
bot.on("presence", function(user, userID, status, game, event) {
/*console.log(user + " is now: " + status);*/
});
bot.on("any", function(event) {
/*console.log(rawEvent)*/ //Logs every event
});
bot.on("disconnect", function() {
console.log("Bot disconnected");
/*bot.connect()*/ //Auto reconnect
});
/*Function declaration area*/
function sendMessages(ID, messageArr, interval) {
var resArr = [], len = messageArr.length;
var callback = typeof(arguments[2]) === 'function' ? arguments[2] : arguments[3];
if (typeof(interval) !== 'number') interval = 1000;
function _sendMessages() {
setTimeout(function() {
if (messageArr[0]) {
bot.sendMessage({
to: ID,
message: messageArr.shift()
}, function(err, res) {
resArr.push(err || res);
if (resArr.length === len) if (typeof(callback) === 'function') callback(resArr);
});
_sendMessages();
}
}, interval);
}
_sendMessages();
}
function sendFiles(channelID, fileArr, interval) {
var resArr = [], len = fileArr.length;
var callback = typeof(arguments[2]) === 'function' ? arguments[2] : arguments[3];
if (typeof(interval) !== 'number') interval = 1000;
function _sendFiles() {
setTimeout(function() {
if (fileArr[0]) {
bot.uploadFile({
to: channelID,
file: fileArr.shift()
}, function(err, res) {
resArr.push(err || res);
if (resArr.length === len) if (typeof(callback) === 'function') callback(resArr);
});
_sendFiles();
}
}, interval);
}
_sendFiles();
}
Finally save the file By pressing CTRL+X and then Enter.
Now Write the command to Start The bot.
node bot.js
All message send to your bot will show here.
Open Discord App and send a message writing "ping" to your bot. You will get a reply "pong".
So Finally we have successfully created a Discord bot from android.
In the next part of the tutorial we will learn about bot function and Create some Bot with STEEM based.
The code of your contribution has been found to be plagiarized from the following source here. Plagiarism is a serious offense. Your account has been accordingly banned for 30 days from receiving utopian reviews.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 5 contributions. Keep up the good work!
Hi @kibria365!
Your post has been upvoted by @bdcommunity.
You can support us by following our curation trail or by delegating SP to us.
20 SP, 50 SP, 100 SP, 300 SP, 500 SP, 1000 SP.
If you are not actively voting for Steem Witnesses, please set us as your voting proxy.
Feel free to join BDCommunity Discord Server.
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
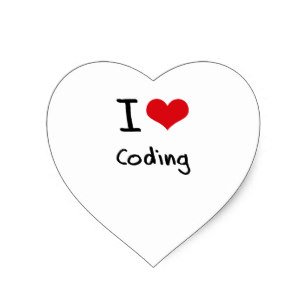
Reply !stop to disable the comment. Thanks!
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by kibria365 from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.
Congratulations @kibria365! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click here to view your Board of Honor
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last post from @steemitboard:
Congratulations @kibria365! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click here to view your Board of Honor
If you no longer want to receive notifications, reply to this comment with the word
STOP
Do not miss the last post from @steemitboard:
সবই মাত্থার উপ্রে দিয়ে গেল গা। যা হোক একটু স্বস্থি লাগলো যে এই সিরিয়ালে linux নাই। একবার লিনাক্স ইন্সটল দিতে গিয়ে পুরা কম্পিউটার ফাকা করে ফেলেছিলাম
Posted using Partiko Android