dsteem Tutorial (Part 3: Dissecting Discussions)
Repository
https://github.com/jnordberg/dsteem
You will learn how to:
- Retrieve Discussion Arrays from dsteem using JavaScript
- Dissect the Discussion Object to see what it contains.
- Interact with Steem without the complications of Node.JS
Requirements
- HTML
- JavaScript
Difficulty
- Basic
Curriculum
Proof of Work Done
https://gist.github.com/edict3d/fba4a4004c960b5549d8f38a7519ab60
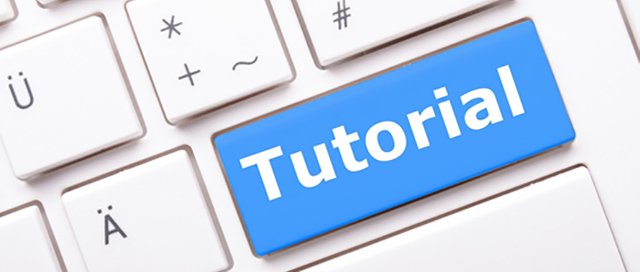
The Discussion Object that the dsteem API provides is the main tool when it comes to scanning the blockchain for information. It's obvious that we need to know exactly what properties this object contains to see what kind of information we can extract from it.
Retrieval
Both comments and root level blog posts count as a Discussion. In order to receive a Discussion from the Steem API using dsteem one must evoke the genaric getDiscussions() function.
var client = new dsteem.Client('https://api.steemit.com')
client.database.getDiscussions()
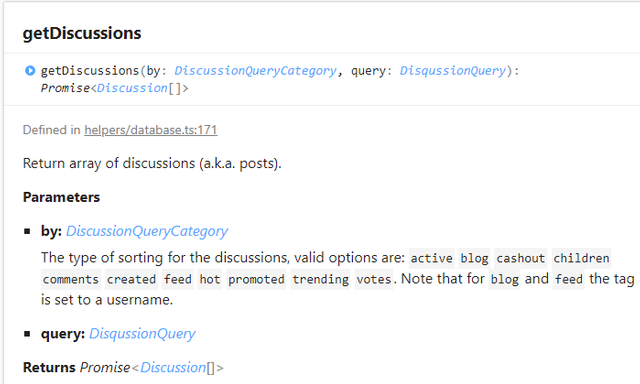
The getDiscussions() function receives a DiscussionQueryCategory and a DisqussionQuery. In return it provides an array of Discussions[] that match the data we provided.
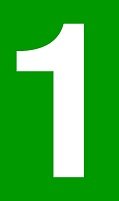
DiscussionQueryCategory
This is simply a string that matches one of the following categories:
- 'active', 'blog', 'cashout', 'children', 'comments',
- 'created', 'feed', 'hot', 'promoted', 'trending', 'votes'
I'm not quite sure what all of these do (active, cashout, children, votes).
I assume 'children' will grab the replies to the author/permlink provided.
Some of the answers can be found at here at the Steem API tutorial website
Active
Posts/Disscussions that are still actively being engaged
Cashout
Posts which was paid out recently
This is just a classic example of how incomplete Steem's tutorials are. That's why I'm here making my own. Created (new), feed, hot, trending, and promoted are at least self-explanatory. For this tutorial we'll be focusing on 'blog' and 'comments'.
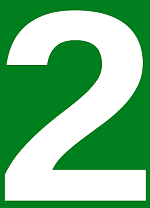
DisqussionQuery
This is the second variable required for calling the getDiscussions() function. It's an object and can contain quite a bit of information.
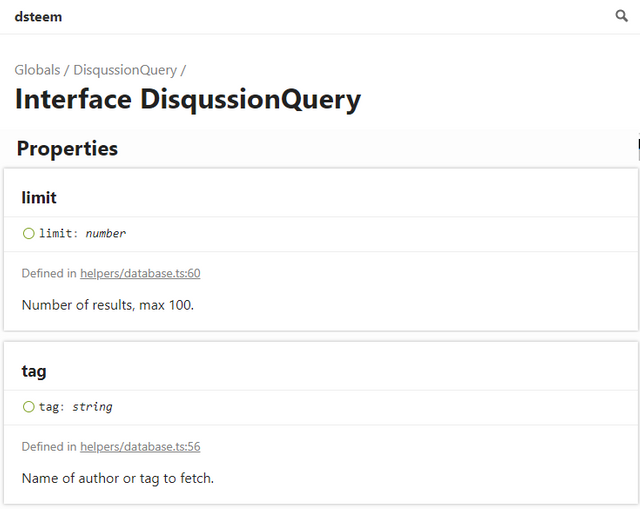
The only required properties of this object are 'tag' and 'limit'. Limit is simply a number from 1 to 100 signifying how many discussions you'd like the function to return. Tag is either the individual author you're looking to target or a generic tag identifier. The most popular Steemit tags can be found here.
There are several optional properties we can add to DisqussionQuery{} to narrow our search:
Options
start_author (string) and start_permlink (string)
These properties come in a pair. You can't use one without the other. In my opinion, these are the most important optional filters we can use. They are necessary for returning a single known post/comment and can also be used to sidestep the 100 post return limitation. Simply grab the first 100 posts and then grab the next 100 starting at the end of the first block of posts you just received.
parent_author (string) and parent_permlink (string)
Similar to start_author and start_permlink, these properties are useful if you're looking to grab the replies (children) of a certain comment/post.
select_authors(string[])
If you had a list of favorite authors you could add them to this array of strings and all discussions returned would be written by them.
select_tags (string[])
This array of strings filters the posts received by the given tags. Perhaps someone you follow posts a wide range of topics. This property would allow you to find content that you're interested in.
filter_tags(string[])
This array of strings will reject any posts with the given tags. It's usefulness works exactly like select_tags, but instead of opting in to the given tags it opts out.
truncate_body (number)
Just in case you're looking to only grab the beginning of post/reply. The number given represents the number of bytes (usually one byte per character) that you'd want to keep. The default is 0 which returns all bytes.
Example getDiscussion() calls
Here's what a real life example of the getDiscussion() function would look like:
This HTML script requests the discussion of my last tutorial and prints it's title to the body of the web page. On line 13 you can see there's a callback to a function:
.then(function(discussions){})
The Steem blockchain returned the object "discussions" containing the array we were looking for. We can then use that array of discussions inside the callback function.
What else?
Being able to print the title of a post that we already knew the author and permlink of is decidedly not impressive. What else do we have access to here? A full list of properties can be found here.
Most of these properties are self-explanatory and are expressed as a string, number, or boolean. Properties of note include author, permlink, title, parent_author, parent_title, body, total_payout_value, and curator_payout_value. Any of these attributes can be accessed using dot notation as shown:
discussions[0].total_payout_value
This would display a string looking something like "16.706 SBD". My post didn't actually receive 16.7 SBD. This is a bit misleading. Instead, my post received 16.70 dollars worth of Steem. There are many strange inconsistencies like this with dsteem. You'll have to keep a lookout for them.
This is the most important property of a discussion when it comes to my next tutorial. My next project will be to create a curation tracker to see exactly how curation is dispersed on the Steem blockchain.
Annoyingly enough, active_votes has zero documentation. The documentation that does exist simply says any[]. It's an array of objects. I had to scan the objects myself to see what was inside them:
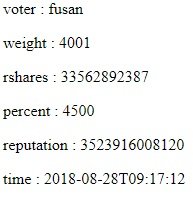
Turns out this is exactly the kind of information I would need to see where curation is going. A description of rshares can be found here. It's basically how the blockchain measures your percentage of the reward pool. I'm still not exactly sure what weight is, but it's clearly vote related.
Conclusion
Obviously it's not ideal that I'm a novice at both dsteem and JavaScript.
As I learn more I will edit this tutorial accordingly.
My next tutorial will explore these active_votes objects more in depth
along with account information in order to create a Steem curation tracker.
This tracker will show exactly how curation rewards taper off as a post receives upvotes.
Thank you for your contribution.
We've been reviewing your tutorial and suggest the following:
Code sections are better displayed using the code markup, instead of placing them as screenshots.
The result returned is a bit confusing.
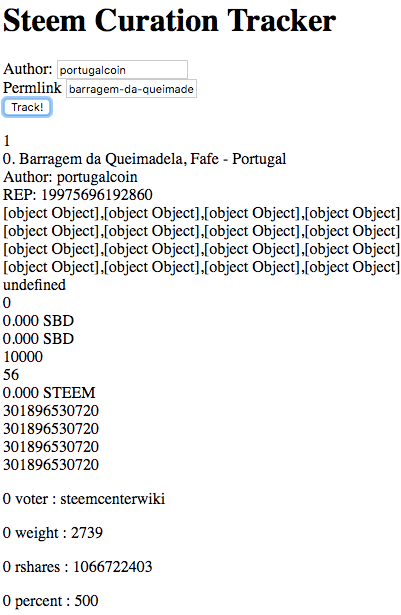
Your tutorial is interesting, thanks for your work.
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 2 contributions. Keep up the good work!
Very well written tutorial post. Here are some points.
The way you like to make more informative tutorial for already not good documented libraries is good approach , I my self doing same thing over my blog but i found that you are covering d-steem while i was covering steem official api. I found this and this is amazing, thanks for making such neat post :) I am not a mod but a utopian io mod can give you a lot of several tips to improve it even more!
Hey @genievot
Here's a tip for your valuable feedback! @Utopian-io loves and incentivises informative comments.
Contributing on Utopian
Learn how to contribute on our website.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hi @edicted!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Your post is drifting down the Resteem River! 🐠
This content has been shared by the BuddyUP team on our @resteemriver account because of the quality and value it provides to the Steem community. The post has also been shared in our discord channel, #curate-a-post, by one of our members.
Keep creating! We're looking forward to your next post.
If you don't appreciate this comment, reply to your post and tag @resteemriver so we can remove it.
Hey, @edicted!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!