JavaScript Dsteem Tutorial (Part 1: Filtering 'New' Tab)
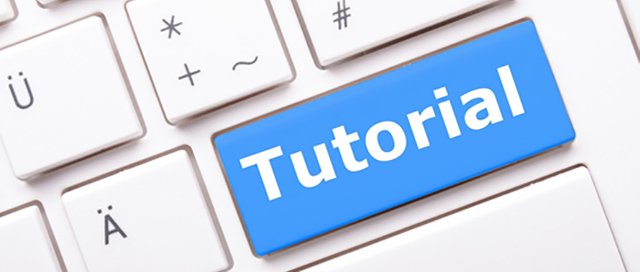
As I wait for an answer to my previous problem I realize that I still have code that works that I can build off of. Time to do something productive instead of banging my head against a wall.
<script src="https://unpkg.com/dsteem@latest/dist/dsteem.js"></script>
<script>
var client = new dsteem.Client('https://api.steemit.com')
client.database.getDiscussions(
'created', {tag: 'tutorial', limit: 100}).then(function(discussions){
var user = discussions[0].author
for (var i = 0; i < discussions.length; i++) {
document.body.innerHTML += '<p>' + i + ". "
+ discussions[i].title + '</p>'
}
})
</script>
Anyone can copy and paste this code into a file and save it as .html
When you open the file it will open a tab in your default browser and look something like this:
You can confirm that this is correct by looking directly on Steemit:
https://steemit.com/created/tutorial
We see that the script worked and returned exactly what we were looking for: The top 100 posts that were most recently created with the "tutorial" tag.
Apparently, the cap for how many posts you can request at a time is limited to 100. As someone who wants to build a filter for the Steemit tabs this is very annoying but I'm sure I'll find a workaround later.
Change the limit to more than 100 to see for yourself:
In order to see these errors you can hit F12 while in Google Chrome.
Okay! We got 100 posts! Let's filter them.
The JavaScript we ran returned 100 "Discussion" objects. What do we have to work with here? Well, first of all, each discussion has an "Author" object. Scratch that... it looks like the "Author" attribute is just a simple string. I wanted to filter these discussions by deleting posts of Authors that had accounts worth less than $100. However, scanning the dsteem github it appears that this information is stored in an object called "Account".
Discussions don't seem to have a link to Accounts, so I'd have to find a function that retrieves accounts from Steem using the Author string. This function might have to get called 100 times... which might take a while... so I'll just use a different filter for now.
Discussions have an attribute called "author_reputation". Gee, I wonder what that could be. Let's use this attribute to reorganize the 100 posts we got in order of highest reputation to least:
<script src="https://unpkg.com/dsteem@latest/dist/dsteem.js"></script>
<script>
var client = new dsteem.Client('https://api.steemit.com')
client.database.getDiscussions(
'created', {tag: 'tutorial', limit: 100}).then(function(discussions){
discussions.sort(function(a, b){
return b.author_reputation - a.author_reputation})
for (var i = 0; i < discussions.length; i++) {
var rep = discussions[i].author_reputation
document.body.innerHTML += '<p>' + i + ". "
+ discussions[i].title + "<br>"
+ "Author: " + discussions[i].author + "<br>"
+ " REP: " + rep + '</p>'
}
})
</script>
By running this code the order of all 100 posts has gone from highest reputation to least. I was very surprised to see that I was only #12 on this list; was expecting higher.
We can see that @ace108 (73) and @steemmonsters (71) are at the top of the list with massive reputations.
This isn't meant to be a smart way to order posts, it's meant to be the simplest example possible to show you all exactly how easy it is to filter the trending, hot, new, and promoted tab.
In the future I will extend this concept further to show just how simple it is to bring my ideas to life. The trending and hot tabs are an absolute joke. They are the reason why bid-bots and vote buying exist. I aim to make them obsolete with a superior filter and curation system. Of course, you are certainly willing to tag along or even help the process. Proof of brain for the win.
Just look at how much information is stored in a Discussion:
https://jnordberg.github.io/dsteem/interfaces/discussion.html
We could be filtering posts based on any of this massive amount of info (and more), but instead we are just ordering them according to how much they are getting paid. On a platform of vote buying, this means nothing. We need a better way.
Thank you for your contribution.
We have been reviewing your tutorial and suggest the following:
The information you put in this tutorial is basic, it can be found on the internet and with better quality.
Your tutorial is quite short for a good tutorial. We recommend you aim for capturing at least 2-3 concepts.
Include proof of work under the shape of a gist or your own github repository containing your code.
In the next tutorial we suggest you use the tutorials template. Link
Please read the guidelines here.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 7 contributions. Keep up the good work!
Nice work :)
I'm on the look out for a nice interface that can toggle posts boosted by a respected list of bot/pay for vote accounts on and off.
The main work then will be maintaining a list, but there are some already on github that are reasonably well looked after.
Good to see we have numerous devs/teams looking at this, cheers!
Hey @abh12345
Here's a tip for your valuable feedback! @Utopian-io loves and incentivises informative comments.
Contributing on Utopian
Learn how to contribute on our website.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Maybe using this we can create a better way to filter articles. Perhaps even some way to blacklist posts boosted by bots.
Yes, I'm going to make a decentralized reputation system of whitelist and blacklist voters.
posts with blacklisted votes can actually hurt them on which order they show up in. Whitelisted votes can be worth more than their displayed value.
We can all choose, in a custom way, which order posts are in.
I think this is awesome. 100% support this endeavour!
Hello World
Hello World!@@@
Congratulations @edicted! You have completed the following achievement on Steemit and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Hi @edicted!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @edicted!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!