dsteem Tutorial (Part 2: Hello World)
Repository
https://github.com/jnordberg/dsteem
What Will I Learn?
- How to reply programmatically using JavaScript and dsteem.
- How to upvote using JavaScript and dsteem.
Requirements
- HTML
- JavaScript
Difficulty
- Basic
Curriculum
Proof of Work Done
https://gist.github.com/edict3d/6513d9b122b3d0898b74958f449660bb
Tutorial Contents
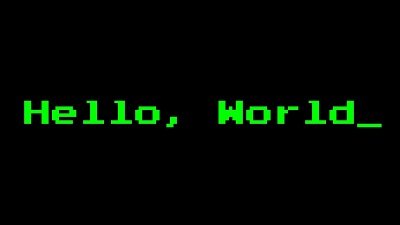
I've spent a lot of time looking for this information and never found it. I suppose we're just expected to look straight at the dsteem github and figure it out for ourselves. This is the Hello World tutorial for dsteem using only javascript (no Node.js). In my opinion, this tutorial (or one similar) should be listed directly an the Steem API tutorials site.
For simplicity, I've broken this up into two different files: pure HTML and pure JavaScript:
HTML File:
<!DOCTYPE html>
<html>
<body>
<h1 id="header"> Steem Login </h1>
<form>
Username@
<input type="text" id="username" value="smartasscards"><br>
Posting key:
<input type="password" id="posting_key" value=""><br>
Author:
<input type="text" id="author" value="edicted"><br>
Permlink:
<input type="text" id="permlink" value="dsteem-tutorial-part-2-hello-world">
</form>
<form>
<input type="text" id="reply" value="Hello World!">
</form>
<button id="hello_world"> Reply </button>
<form>
Vote Power (1-10000)
<input type="number" id="vote_power" min="1" max="10000" value="850"><br>
</form>
<button id="upvote"> upvote </button><br>
<script src="https://unpkg.com/dsteem@latest/dist/dsteem.js"></script>
<script src="HelloWorld.js"></script>
</body>
</html>
JavaScript File:
var client = new dsteem.Client('https://api.steemit.com')
// This function will reply to the given author/permlink
function comment(){
var key = dsteem.PrivateKey.fromString(
document.getElementById("posting_key").value)
client.broadcast.comment({
author: document.getElementById("username").value,
body: document.getElementById("reply").value,
json_metadata: "",
parent_author: document.getElementById("author").value,
parent_permlink: document.getElementById("permlink").value,
permlink: document.getElementById("permlink").value,
title: ""
}, key).then(function(result){
console.log('Included in block: ' + result.block_num)
}, function(error) {
console.error(error)
})
}
// This function will upvote the given author/permlink
function vote(){
var key = dsteem.PrivateKey.fromString(
document.getElementById("posting_key").value)
client.broadcast.vote({
voter: document.getElementById("username").value,
author: document.getElementById("author").value,
permlink: document.getElementById("permlink").value,
weight: Number(document.getElementById("vote_power").value)
}, key).then(function(result){
console.log('Included in block: ' + result.block_num)
}, function(error) {
console.error(error)
})
}
var POSTING_KEY = "5JmiyGm" + "BRbhhbZ" + "McE4vrm" + "utuy7rTuSG" + "AhHurT5Ujt" + "mhC1bgKRz1"
document.getElementById("posting_key").value = POSTING_KEY;
// Binds the hello_world button to function comment()
document.getElementById("hello_world").onclick = comment
// Binds the upvote button to function vote()
document.getElementById("upvote").onclick = vote
Copy/Paste the html code into a .html file and the JavaScript code into a .js file respectively. Put the files into the same folder. The .js file must be called HelloWorld.js unless this line is modified:
<script src="HelloWorld.js"></script>
Open the HTML file and this is what you should see:
As you can see, logging into the Steem blockchain is as easy as typing a few simple lines of code. This is one of the reasons why the blockchain is so powerful. All developers get easy access to an incredibly secure system that even has its own currency attached to it. The upvote system is revolutionary as well and provides the opportunity to create new business models that aren't possible on other blockchains.
You'll notice this HTML page has seven parts to it:
1. Username@
This is your username.
2. Posting key
This is the posting key that matches the username. I realized that some of you would be afraid to put your private key into this field. You shouldn't be; this code is running directly on your computer and no one else will see it. However, just in case you don't want to take my word for it I have provided my dummy account @smartasscards.
Originally I was thinking my Cards Against Humanity clone Smartass Cards should have its own account. Now I realize this wouldn't be decentralized. I've instead decided to use this account for testing purposes. Now anyone reading this tutorial can upvote and post under my dummy account @smartasscards. The username and password have been provided by default.
3. Author
This is the author of the post/comment you want to upvote/reply to.
4. Permlink
This is the link to the post/comment you want to upvote/reply. Steem does not use full links. Instead they use a combination of the author and permlink to create unique identifiers for posts and replies. The Permlink is the text that appears after the last forward slash of the full link:
An easy way to access the permlink from Steemit.com is to click on the time link of the reply:
5. Reply Button
This button will take the text in the field above it and use that text as the body of a comment. This comment will reply to the author/permlink combo given in the previous fields. It will use the Username@ and Posting key fields to sign the transaction. Using this simple code, you can programmatically reply to any post/comment on the Steem blockchain. All you need are valid values in the first four text fields.
6. Vote Power (1-10000)
This extra variable is required if you want to upvote the post or comment that we just replied to. You must choose a value between 1 and 10,000. Each point counts as 0.01% of a full vote.
This function has a "dust cutoff". If your vote would be so low that it rounds to zero the Steem blockchain will not allow you to cast it. If the account in question doesn't have enough Steem Power and you choose a vote power that is too low you will receive the following error (view errors with F12 in Chrome):
What I learned creating this tutorial is that my account (2100 SP) can vote as low as 2 (0.02%) vote power. My account is only one point away from being able to cast the minimum vote. A new account with only 15 SP can vote as low as 825 (8.25%) if that account is fully powered up. The more power you spend the bigger the minimum vote becomes in order to breach the dust threshold.
For some reason I had to cast the number received from the HTML number field back into a number in the .js file:
weight: Number(document.getElementById("vote_power").value)
It wasn't registering as a number. Don't forget that I'm pretty much an HTLM and JavaScript noob :D
7. upvote button
This button will upvote the author/permlink combo using the username/password credentials. It will vote with the power specified in the vote power number field. Feel free to play around with this. You can even use the @smartasscards account to upvote your own posts if you so choose. If you change the vote power and attempt to upvote the same post, this will not give an error. The new vote will override the old vote and the power from the old vote will simply be wasted. However, if you try to upvote the same post with the same voting power you'll receive this error:
Unknowns
The "client.broadcast.comment({" function contains a variable called "json_metadata". I set the value to an empty string because I don't know what this can do. I also set the "title" variable to an empty string. I believe giving the comment a title and setting the "parent_author" and "parent_permlink" to an empty string would create a top level blog post instead of a comment.
Knowns
For simplicity, the permlink of the reply is identical to the permlink of the original post/comment.
parent_permlink: document.getElementById("permlink").value,
permlink: document.getElementById("permlink").value,
This means that if you try to use this code to reply to yourself it will give an error because the author/permlink combo would be ambiguous. Also, it means that you can't use this code to reply more than once to the same post/comment (for the same reason). However, if you try to post a second time you will not receive an error, instead the blockchain will automatically edit the original reply to match the body of the new reply (instead of trying to create a new reply).
Default values
username/password for account @smartasscards has been provided.
This tutorial is meta and references itself.
Default author is edicted and default permlink is the permlink of this tutorial:
dsteem-tutorial-part-2-hello-world
Default vote power is 850 (which is close to the minimum possible for the 15 Steem Power account @smartasscards. If the upvote isn't working raise this number. Check account at https://steemd.com/@smartasscards to see if this shared account even has the power to vote.
Conclusion
I've learned a lot making this tutorial. Remember that I'm a novice myself so some of the things I've done here may be inefficient or flat out incorrect. I hope that my work here can save other developers the headache of figuring out the basic dsteem commands for the Steem API.
My future tutorials will be focused on using this basic functionality to sidestep Node.JS. Instead of creating our own centralized servers we will force the Steem blockchain to be the server. All information will be stored directly on the blockchain in the form of comment text. I will provide more open source modules and tutorials to streamline this process. Witness me.
Thank you for your contribution.
After review your contribution we recommend the following points below:
In code it's very important to have comments explaining some lines. It helps the user who is reading your tutorial to better interpret the code.
In the requirements we recommend that you put basic knowledge of HTML and Javascript.
There is a tutorial very similar to yours, it's more intuitive and easy to learn but with steemjs. Link
Again -> include proof of work under the shape of a gist or your own github repository containing your code.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your suggestions! I've added the HTML and JavaScript requirements and a few comment lines.
The goal of this tutorial was to produce code so simple and self-explanatory that adding comments would only clutter the file. Could you be more specific about which lines are unclear/unexplained? Even the few comments that I did add feel extremely redundant. My tutorial goes into painstaking detail as to what each line does. The tutorial that you linked only has a single comment line.
Speaking of the tutorial that you linked, you make the claim that it is:
After reviewing this tutorial I could not disagree more. This tutorial is littered with many unnecessary complexities such as div tags, CCS, and jquery documents. The point of the tutorial is to interface with the Steem blockchain; not to make the interface look pretty. My code is much simpler and to the point for good reason.
Also, as you say, the tutorial linked is for SteemJS. This is basically a deprecated library. Even Steemit Inc, the creator of this library, doesn't even use it anymore and no references to it are made in the official tutorials section of the Steem API.
I don't understand why you would compare my dsteem tutorial to a deprecated and overly-complicated tutorial. I created this tutorial because I believe there is a wide chasm and completely unnecessary barrier to entry when it comes to using the dsteem library. It has nothing to do with SteemJS, even if the basic functionality is the same.
As far as linking my code to github: I'm working on that right now. Thank you for your time.
Thank you for your review, @portugalcoin!
So far this week you've reviewed 17 contributions. Keep up the good work!
This comment will change to "Hello World" if default settings are used
omg someone actually used it 10 days after the fact.
Thank you. Looking forward to more steemD tutorials specifically the steem dev site is absolutely horrible for any info even deducing wtf is going on at times as person has to with code, no real value tutorials just stupid get the posts nonsense, this has already gone beyond most things they have on that site in less time.
Good job on the tutorials. I have also written a small bot using node to vote on posts. I will make a tutorial on that.
Hey, @edicted!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!