PHP Tutorial #08 Array Methods ( Array_Replace, Array_Values, Array_Slice and Array_Intersect )
Repository
https://github.com/php/php-src
What Will I Learn?
I will learn the array methods ( Array_Replace, Array_Values, Array_Slice and Array_Intersect ) with examples.
- How to replace values using " array_replace " method.
- How to get values from an associative/indexed array using " array_values " method.
- What's the " array_slice " method and how to use it.
- The definition and uses of the " array_intersect " method.
Requirements
- Server support PHP , Xampp or Wamp for example
- An IDE like Sublime text.
- Browser (Chrome for example)
Difficulty
- Basic
Description
1– Array_Replace
The array_replace method replaces the values of array1 with values that have the same keys in each of the following arrays. If there is a key from the first array in the second array, its value is replaced by the corresponding key value of the second array. If the key is in the second array, and not in the first, it will be created in the first array. If a key exists only in the first array, it will stay as it is. If multiple replacement arrays are passed, they will be processed in order, and subsequent arrays overwrite the previous values.
The array_replace method is not recursive : The values in the first array will be replaced by any type in the second array. It has at least two arrays as parameters , the array in which the elements will be replaced. And the array from which the elements are extracted.
The method returns an array, or the NULL value, if an error occurs.
To use the array_replace method we need to pass the arrays as parameter
array_replace($array1, $array2);
I have an array of persons and I want to replace some persons using another array
$team = array("Person1" => "Alexendre", "Person2" => "Alex", "Person3" => "John", "Person4" => "Arden", "Person5" => "Mick");
$replacedTeam = array("Person1" => "Mickel", "Person5" => "Gabriel");
print_r(array_replace($team, $replacedTeam));
I have used " array_replace " and I passed the $team and $replacedTeam arrays as parameters , the method will replace the values of keys exist in the first array by the values of the second array and this is the result
2– Array_Values
The array_values function returns all values of the array elements and recalculates the array numerically.
It has the array as parameter and it returns an array of values.
To use the array_values method we need to pass an array as parameter
array_values($array);
I have an array of languages and I want to get just the values of this array
$languages = array("en" => "English", "fr" => "French", "sp" => "Spanish"); print_r(array_values($languages));
The array_values will return an array of values indexed from 0 to the last element
3- Array_Slice
The array_slice method returns a set of successive elements of the array as specified by the offset and length parameters. It has 4 parameters the array is the first parameter, the second parameter is the offset. If the offset coefficient is not negative, the relay will start from the beginning of the array. If offset is negative, the relay will start from the end of the array.
The third parameter is the length If the length coefficient is positive, the number of consecutive elements is equal. If the array is shorter than length, only the available array elements will be returned. If the length is negative, the sequence will stop at this number of elements descending from the end of the array. If you do not specify the value of this optional parameter, the sequence will be made up of all elements from offset to the end of the array.
The fourth parameter is the perverse key the array_slice will reorder and set the numeric array pointers by default. But we can change this behavior by setting the value of the reserve_keys parameter to TRUE. The method returns a portion of the array. If the offset coefficient is greater than the size of the array, the function returns an empty array.
To use the array_slice we need to pass the array , the start index , the length and the optional parameter which is the preverse key
array_slice($array,$start,$length,$preverse);
I have an array of friends and I want to use array_slice method to get a special elements in the array
$teamS = array( "Alexendre", "Alex", "John", "Arden", "Mick");
print_r($teamS);
print_r(array_slice($teamS, 1, 3));
I passed the $teamS array as parameter , the start index is 1 it will start from the second element and the length that will be is 3 , this is the result
4– Array_Intersect
The function returns all array1 values in all other arrays. Note that the function preserves the keys associated with the returned values. It has at least two arrays as parameters and it return an array.
To use the array_intersect method we need to pass at least to parameters
array_intersect($array1,$array2);
I have two arrays group1 and group2 each array contains number of persons, I want to get the persons exist in the first and second arrays
$group1 = array("Person1", "Person2", "Person3", "Person4", "Person5");
$group2 = array("Person1", "Person3", "person6");
print_r(array_intersect($group1, $group2));
The array_intersect will return an array contains the elements exist in group1 and group2, it will return person1 and person3 because they are in the two arrays , this is the result
Video Tutorial
Curriculum
- PHP Tutorial #01 Indexed Arrays, Associative Arrays and Multidimensional Arrays
- PHP Tutorial #02 Array Methods (Sort, Rsort, Ksort, Krsort , Array_Reverse And Shuffle)
- PHP Tutorial #03 Array Methods ( Search Methods , Addition Methods and the Remove Methods)
- PHP Tutorial #04 Array Methods (Array_Sum, Array_Rand, Array_Column and Array_Unique)
- PHP Tutorial #05 Array Methods (Array_Chunk, Array_Combine, Array_Count_Values and Array_Product)
- PHP Tutorial #06 Array Methods (Array_Fill_Keys,Array_Fill, Array_Flip and Array_Filter)
- PHP Tutorial #07 Array Methods ( Array_Keys, Array_Map, Array_Merge and Array_Pad )
Proof of Work Done
https://github.com/alexendre-maxim/PHP-Tutorial/blob/master/replace.php
Hi @alexandre-maxim,
Thank you for your video tutorial contribution.
I see that your overall presentation has improved since the last time I evaluated your video tutorial.
Here are some areas that you can pay attention to improve on your video presentations:
Since teaching plays a very important part in the tutorial, try to put yourself in the role of a lecturer and that you have a group of about 100 students in front of you. When you speak, you will need to be more deliberate as you pronounce each word. Often times, video presenters do not realize this and when they teach, they are 'chatting' with the audience. Chatting is not the same as 'teaching'.
If you pay more attention in the 'teaching' skill, your audio presentation will come out stronger. Your words will be more distinct. Right now, some of your words run into each other meaning that your learners don't catch every words that you say and are left with guessing. Especially if English is your second language, speaking more slowly and deliberately will make your overall presentation more clear.
In your video presentation, you can improve on the visual aspect. I see that you use on screen arrows and circling. The lines are not consistent and too thin. You need to practice in making better arrows pointing and also circling.
Let me know if you have questions on these feedback.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @rosatravels!
So far this week you've reviewed 1 contributions. Keep up the good work!
Thank you @rosatravels , I will try to speak slowly and with understandable words.
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
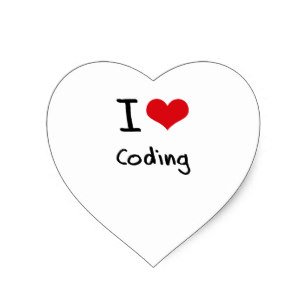
Reply !stop to disable the comment. Thanks!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
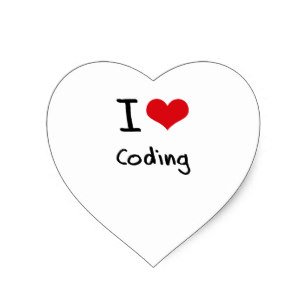
Reply !stop to disable the comment. Thanks!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
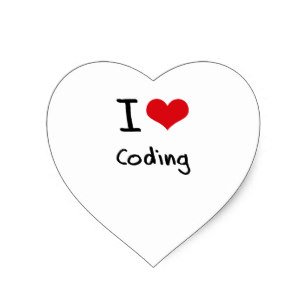
Reply !stop to disable the comment. Thanks!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
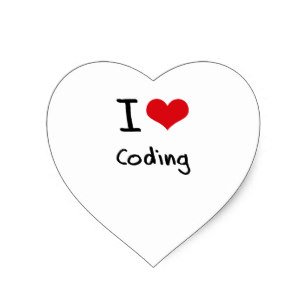
Reply !stop to disable the comment. Thanks!
Thank you
Hi @alexendre-maxim!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Thank you
Hey, @alexendre-maxim!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Thank you