PHP Tutorial #05 Array Methods (Array_Chunk, Array_Combine, Array_Count_Values and Array_Product)
Repository
https://github.com/php/php-src
What Will I Learn?
I will learn the array methods part 04 ( array_chunk , array_combine , array_count_values and array_product ).
- How to divide an array into small arrays using array_chunk method.
- How to combine between keys and values to generate an associative array using array_combine method.
- How to count values in array using array_count_values method.
- What's the array_product and how to use it.
Requirements
- Server support PHP , Xampp or Wamp for example
- An IDE like Sublime text.
- Browser (Chrome for example)
Difficulty
- Basic
Description
In this tutorial we will continue our series about the PHP programming language, we will talk about the arrays methods the fourth part (Array_Chunk, Array_Combine, Array_Count_Values and Array_Product ), let's start ..
1 - Array_Chunk :
The array_chunk method divides the array into smaller arrays each with a number of elements equal to the $size parameter value. The last small array may contain fewer elements than the $size parameter value.
It has 3 parameters the first is the " Array " , the second is " Size " which is the size of each partial array is cut from the original array and the third parameter is the " Perverse Key " by default the value is false it means the indexes in the new array or in the small array will start from 0 , if you change this value to true, the array will keep the keys in the resulting small arrays. This method returns a multidimensional array numerically, starting at zero.
Each dimension of the array contains a number of elements equal to the value of the $ size parameter. This method causes an error of type E_WARNING if the $size operand is smaller than 1 and the method will return NULL in that case.
There is two ways to use the array_chunk , this method will return a multidimensional array so you can create an array and get the result in this array , the second way is to print directly the array using " print_r " method
$result = array_chunk($array);
print_r(array_chunk($array));
I have an array and it contains 5 persons , I want to divide this array into small arrays each one will contain 2 persons and to do that we need to use the array_chunk method
$teamI = array( "Persone1", "Persone2","Persone3","Persone4","Persone5");
echo "<pre>";
print_r($teamI);
$groupI = array_chunk($teamI, 2);
print_r($groupI);
This is my code I will firstly print the $teamI array then I will print the result $groupI
If you look at the indexes , for each small array the keys starting from 0 , I want to keep the original keys for each value and to do that we need to change the third parameter to true and this is the result
2 - Array_Combine :
The method creates a new array by using the values of the array passed through the keys parameter as keys, and using the values of the array passed through the parameter values as values associated with these keys.
It has two parameters , the first is the keys which is an array of keys that this function uses. Any value that is not accepted as a key will be converted to a text string and the second parameter is the values which is another array contains the values that you will use.
The method returns a new array resulting from merging the key and value arrays, and returns FALSE if the number of elements in the two arrays is uneven.
The method causes an E_WARNING error if the number of elements in the value and key arrays is not equal.
To use the array_combine we have two ways , the normal way to create an array and the second way to print it directly
$result = array_combine($arrayKeys, $arrayValues);
print_r(array_combine($arrayKeys, $arrayValues));
I will create an associative array using the array_combine method , I will create two indexed arrays for the keys and values , this is the code
$languagesKeys = array("en","fr","es");
$languagesValues = array("English","French","Spanish");
$languages = array_combine($languagesKeys, $languagesValues);
print_r($languages);
The array_combine will combine between the keys and values and this is the result
3- Array_Count_Values :
The array_count_values function creates a new array that contains the values in the array passed by the array as keys, and the frequency of each value in that array as values associated with those keys.
The method returns a new array of values in the passed array, and each value is associated with how many times it is repeated in the array.
It will generate an E_WARNING error for each element in the array, not a string or an integer.
To use the array_count_values we pass the array as parameter
$result = array_count_values($array);
print_r(array_count_values($array));
I will count the number of each person in the array and for that I will use this method
$votes = array("persone1","persone2","persone1","persone3","persone5","persone3","persone2","persone1","persone2","persone2","persone3","persone4","persone5","persone1");
print_r($votes);
print_r(array_count_values($votes));
The method will count and return for as an array the keys are the persons and the values are the numbers
4 - Array_Product :
The array_product () method returns the value of multiplying the values of the array elements.
It has the array as parameter , and it twill return the product as an integer or float.
To use the array_product method we have two ways, the first is to pass an array as parameter , and the second to pass values
$result = array_product($array);
$result = array_product($value1,$value2,$value3);
I will create an array of numbers , I will use the array_product and this is the code
$number = array(2,4,3);
echo("The result is : " . array_product($number));
The array contains 3 values " 2, 4 , 3 " , the array product will multiply 2 by 4 and the result by 3
Video Tutorial
Curriculum
- PHP Tutorial #01 Indexed Arrays, Associative Arrays and Multidimensional Arrays
- PHP Tutorial #02 Array Methods (Sort, Rsort, Ksort, Krsort , Array_Reverse And Shuffle)
- PHP Tutorial #03 Array Methods ( Search Methods , Addition Methods and the Remove Methods)
- PHP Tutorial #04 Array Methods (Array_Sum, Array_Rand, Array_Column and Array_Unique)
Proof of Work Done
https://github.com/alexendre-maxim/PHP-Tutorial/blob/master/chank_combine.php
I thank you for your contribution. Here are my thoughts;
As I stated in my last review on your tutorials, there were lots of structure and grammar mistakes in your speech. I suggest you structuring your speech before recording it and practicing it until it is reliable. Having these mistakes makes your speech hard to understand. A (video) tutorial must be formal and easily understood. Still, it's no shame and I can easily see that you improved yourself.
In my last review on your tutorials, I stated that writing examples while recording is a bad habit. I'm grateful that you prepare your examples beforehand. But now, same thing happens with the charts. There are online chart programs like draw.io, I suggest using one of these and preparing the structure for the charts before recording. Then, you can easily paint over them to show what you mean without losing time and causing confusions. Here is an example I made for your first chart:
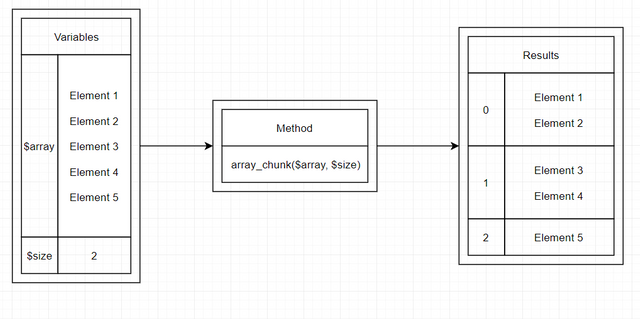
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you @yokunjon , in the next tutorial I will prepare the examples and I will train more to avoid mistakes
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
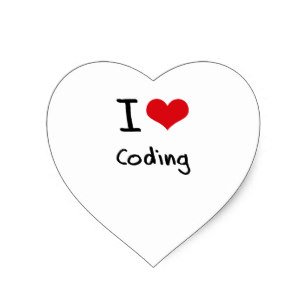
Reply !stop to disable the comment. Thanks!
Thank you
Hi @alexendre-maxim!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Thank you @steem-ua for your support
Nice work @alexendre-maxim.
Staff Pick
Thank you @buckydurddle , I am proud by your encouragement.
Hey, @alexendre-maxim!
Thanks for contributing on Utopian.
Congratulations! Your contribution was Staff Picked to receive a maximum vote for the video-tutorials category on Utopian for being of significant value to the project and the open source community.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Thank you