PHP Tutorial #03 Array Methods ( Search Methods , Addition Methods and the Remove Methods)
Repository
https://github.com/php/php-src
What Will I Learn?
I will learn the array methods part 2 (Array_Search, In_Array, Array_Key_Exists, Array_Unshift, Array_Push, Array_Shift and Array_Pop).
- How to search in array using the search methods
- How to add values to array using the addition methods
- How to remove from an array using the remove methods
Requirements
- Server support PHP , Xampp or Wamp for example
- An IDE like Sublime text.
- Browser (Chrome for example)
Difficulty
- Basic
Description
In this tutorial we will continue our series about PHP programming language, we will take 3 concepts , how to search in array , how to add values to an array and how to remove from an array using the array methods ( array_search, in array, array_key_exists , array_unshift , array_push,array_ pop and array_shift ), let's start ..
1 - Search Methods :
There are many ways to search a value in a particular array, in this tutorial we will use the " Array_Search and In_Array " for the indexed arrays and " Array_Key_Exists " for the associative arrays.
A - Array_Search Method :
The array_search () method searches for a specified value and returns the key to the first element that holds this value if it succeeds. This method in the "haystack" array looks for the value of the "needle".
The needle Is the value that we search and the Haystack Is the array that we will search in.
If the needle is a string, the comparison will be sensitive to the letters case, this method returns the element key to the value of the needle if it is found in the array, or returns FALSE if it's not found.
To use the array_search() method we must pass two parameters at least, the needle and the haystack
array_search(Needle, Array);
In my example I have created an indexed array and I will search if " Alex " exists in my array and this is my code
if(array_search("Alex", $friends)){
echo "The element exists! (Array_Search)";
}
The array_search will return true with the key if the element exists , so if the condition is true I will print by the echo method "The element exists!" and this is the result
B - In_Array Method :
The in_array () method checks for a value in an array, the same as array search it will return the key that holds the value if it succeeds or false if it fails.
Always we have 3 parameters , haystack, needle and the strict value " True or False ".
The Strict Parameter:
If we make the third parameter true, the method will check if the needle and the value found in the haystack are equals , if they are equals it will return the key , else it will return false.
To use the in_array method we pass three parameters " the needle, the haystack and the strict "
in_array(needle, haystack, strict);
I will continue with the previous example, I will check if " alex " exists in the friends array by the ' in_array ' method
echo "<h3>In_Array</h3>";
if(in_array("alex", $friends,true)){
echo "The element exists! (In_Array)";
} else {
echo "The element <span style='color:red;font-size:20px'>not</span> exists! (In_Array)";
}
The value that I passed is " alex " with small " a " and this is the result, it will execute the else content
C - Array_Key_Exists Method :
This method verifies that the key or index exists within the array. The method returns the value true if the key is present . And false if the key is not present in the array .
The array_key_exists will look for keys within the first dimension only. The method will not find nested keys in multidimensional arrays.
To use the array_key_exists method we pass the array and the key
array_key_exists(key, array);
I will use the " languages " array because it's an associative array , I will search for the key " en " and this is my code
echo "<h3>Array_Key_Exists</h3>";
if(array_key_exists("en", $languages)) {
echo "The element exists! (Array_Key_Exists)";
}
If the "en" key exists in our languages array the system will print " The element exists! " and this is the result
2 - The Add Methods :
You can add more values to your array and there are many methods to add , in this tutorial we will use " Unshift and Push methods".
A - Array Unshift Method :
This method adds one or more elements at the beggining of the array.
Note that the list of items enters the beginning of the array at once, so that the inserted elements remain in the same order. All the numeric array keys will be modified to start counting from scratch while the craft keys will not change.
To use the array_unshift we need to pass the array and the values
array_unshift(array, values);
I will use the friends array to add a new friend
echo "<h3>Unshift Method </h3>";
array_unshift($friends, "Mick");
echo "<h3>My Friends </h3>";
foreach ($friends as $friend) {
echo $friend . " is my friend <br>";
}
I have added a new friend named " Mick " and I will print the new array
B - Array Push Method :
This method adds one or more elements at the end of the array.
The array_push () method treats the array as a stack, drives the variables we passed to the end of the array, and increases its length by the number of variables we add.
To use the array_unshift we need to pass the array and the values
array_push(array, values);
I will add a new friend at the end of our friends using the array_push method
echo "<h3>Push Method </h3>";
array_push($friends, "Arden");
echo "<h3>My Friends </h3>";
foreach ($friends as $friend) {
echo $friend . " is my friend <br>";
}
I will add " Arden " at the end of the array , we can use this method to add values to the associative arrays also
3 - The Remove Methods :
You can remove elements from an array using the remove methods , we will learn the " array_shift and array_pop " methods.
A - Array Shift Method :
Array_shift () removes the first element of the array and returns its value, shortening the array by one element and displacing all elements towards the beginning of the array.
Modifies all the numerical keys of the array to start counting from scratch while the literal keys do not change.
To use this method we need to pass only the array as parameter
array_shift(array);
We have added " Mick " using the " Unshift " method and now we will remove " Mick " using the " Shift" Method
echo "<h3>Shift Method </h3>";
array_shift($friends);
echo "<h3>My Friends </h3>";
foreach ($friends as $friend) {
echo $friend . " is my friend <br>";
}
We will remove the first element of the " friends " array , " Mick " will be removed and this is the result
B - Array Pop Method :
The array_pop () method drives the last element of the array and returns its value, reducing the length of the array by one element. The method returns the value of the last element of the array.
If the array is empty ,the function returns the value " NULL ", it will give an E_WARNING error when used on a non-array value.
To use this method we need to pass only the array as parameter
array_pop(array);
We have added " Arden " using the array_push method and now we will remove it using the array_pop method
echo "<h3>Pop Method </h3>";
array_pop($friends);
echo "<h3>My Friends </h3>";
foreach ($friends as $friend) {
echo $friend . " is my friend <br>";
}
Arden is the last element of the array and the pop method will remove the last element, this is the result
Video Tutorial
Hi @alexandre-maxim
I see improvement in your video tutorials since the last time I reviewed your contribution. You are speaking more clearly and making your presentation easier to follow.
When it comes to tutorials, you need to have the mind set of a teacher when you teach the concepts. Showing people what you do is not enough in a tutorial. When it comes to teaching, it takes more than just explanation. You need to show your students the principles behind the concepts so that the learners can take away the skill and be able to do them on their own after the tutorial. Next time, try to work on the 'teaching' aspect. This is the hardest part for many people as they have not gone through the school of teaching. So you will need to learn this by practice.
Let me know if you have any questions.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @rosatravels!
So far this week you've reviewed 1 contributions. Keep up the good work!
Thank you @rosatravels , I will try to be different in my explanation .
woo, this was nice, I came thinking I know everything and I found that you can unshift, which is basic but I never ever looked at it, I made an algo to do it lol. Embarrassing. Will have to search for unshift and shifts brothers in other languages as well.
Hey @hispeedimagins
Here's a tip for your valuable feedback! @Utopian-io loves and incentivises informative comments.
Contributing on Utopian
Learn how to contribute on our website.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
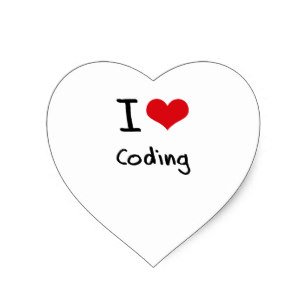
Reply !stop to disable the comment. Thanks!
Thank you
Hey, @alexendre-maxim!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!