[Unity Game Development Tutorial: 14 : Turing Game: 5] - Wrapping things up... theory... where could you go and conclusion of this series
Wrapping things up, delving into the future, philosophy of the mind, and more...
The last few tutorials in this situation would seem to indicate that people are more interested in theory of this idea than they are the actual code. The theory post did substantially better than my posts where I did actual code. The post yesterday took me the longest out of all of the posts due to the amount of code, testing, putting a demo out on the web hosting, etc. It so far is the worst I've done in awhile in terms of payouts on these tutorials and it is the one that I put the most effort into.
That is okay though that post, covered the basics and has a working demo. It was approached as an early example of how to build something like this.
THIS POST I want to focus on what you might consider doing next, and the crazy ways this can be taken. I am not going to write any further code on this topic, as that is not what seems to be of the most interest at this point. This will be another theoretical post and likely will be the last I do on Turing Game style posts unless people have specific questions that justify making more of these. (I will move on to other types of posts and my next tutorial section when I start it will likely be on another type of game)
The Demo, and links to the past
If you did not try out the demo from the last post. I am including it in this post as well.
Click on this link and wait for it to load in your browser if you want to try it out.
It will load a screen like the following. In the lower left corner of the screen it says enter text. Click there, type something and press enter.
IF you want to know how this was made and missed the previous tutorials on this:
[1] - Setting up the UI so we can attach code and begin the game
[2] - Getting input, output, and simple parsing working
[3] - Theoretical/Design Discussion - no code or development
[4] - Simulating conversation, now we're getting somewhere...
What might the next steps be?
This project somewhat mirrors my experiments in my youth. So I can recount a lot of things I did next from a theoretical standing, and show you how far I took it. Then I can share some ideas with you as far as where I was considering taking it next. I will also share some practical uses for this in terms of game design at the end, before I conclude this series.
When making one of these you need to implement rules, and play with it. Where does it seem odd? What could you do to fix this?
Source: giphy.com
Emotions
In my youth I decided to make a variable that essentially contained an integer for how much the computer liked you. Praise and saying nice things could shift the variable in the positive, and insulting or being mean or aggressive could lower the value of the variable.
I could then use this variable to tailor the responses to be more in line with what the emotions of the computer should be like if they were human.
I also played with emotions like boredom, sadness, happiness, etc. Boredom was a very fun one. If you implement a timer so that the Computer responds in an amount of time you could expect a person to type out the response that makes it more realistic. Another step is to have a counter for how long until the player responds. I took it far enough that I'd have the computer start making statements of its own if there was inactivity.
Source: giphy.com
These are all things to consider for making your simulation seem more real. All we had in the working demo above is something that used a similar approach to Eliza and hadn't started getting overhauled for more serious work.
Multiple Sentences
You could make the computer identify sentences and break them out into arrays of strings first where each string was a sentence. Then you could tokenize each sentence one at a time. You could first respond to each sentence individually using something like we already have made, or you could go for a little more realism and try to make it build something based upon the total input. This could get complex very quickly, but it could lead to more realism.
Simple Logic
My next steps after doing the above was to make a simple logic base system. If certain words occurred I would kick in the logic system.
X IS Y
X ARE Y
X HAS Y
X DOES Y
X DOES NOT Y
X DOES NOT HAVE Y
X IS NOT Y
X ARE NOT Y
X WANTS Y
X DOES NOT WANT Y
X NEEDS Y
X DOES NOT NEED Y
X IS PART OF Y
X IS NOT PART OF Y
The key for me is anytime a phrase like this occurred I would check X against a list of words I had saved. If I found X then I would now view it as a number. If I did not find X I would add it to the list and now it has a number. I would do the same thing with Y.
Source: giphy.com
So now we are essentially talking about the relationship between two numbers X and Y. I would not ASSUME any of those things above. I could convert each of those values above to a number. So I essentially had X RELATIONSHIP_NUMBER Y for each pairing. Some combinations of the above are mutually exclusive so they cancel each other out. In those cases if the AI is learning during the conversation you should ask questions to determine which trait should win out. You could even weight it so the more references they hear to a specific relationship the stronger they believe that relationship to be true. It would then be more difficult to try to counter that. Sounds kind of human like? How stubborn should the AI be?
So each word would have a definition which would consist of another number and a relationship number. So the definition of each word could be extended.
RelationShip Number might be something like 0=relationship unknown, 1=IS, 2=IS NOT, 3=HAS, 4=HAS NOT, 5=DOES, 6=DOES NOT, 7=WANTS, 8=WANTS NOT, 9=NEEDS, 10=NEEDS NOT, 11=PART OF, 12=NOT PART OF
The difference between NEEDS and WANTS was simply that NEEDS were things that are required for survival. WANTS are things that are wanted, but not required for survival.
Learning, Recursion, and examples
The first time I ever implemented something like the above I actually wrote it in a basic programming language. It was a variant that did not support recursion very well. You could fake it using GOSUB and ENDSUB type setups, but that had limitations. I came up with the idea of having the program only traverse three levels deep.
Here is an example:
Deva is a Human
A Human is a Mammal
A Mammal is an Animal
A Mammal Has Hair
A Reptile is an Animal
A Reptile does not have Hair
Source: giphy.com
So breaking that down into numbers...
Deva=1, Human=2, Mammal=3, Animal=4, Hair=5, Reptile=6
Let's theoretically define the words as:
Word[#]:definitionblock:definitionblock
We will define a definition block as:
logical#[word#]
So now we can take my example and build what that should look like:
Deva[1]:1[2]
Human[2]:1[3]
Mammal[3]:1[4]:3[5]
Animal[4]:3[5]
Hair[5]:
Reptile[6]:1[4]:4[5]
So if you were to look at this table the relationship of Hair to Deva (that's me) is 1(self),2(human),3(Mammal),4(hair). So it is 4 levels away.
Traversing each level is where the recursion would come in. I decided to limit the recursion to say 3 levels.
So asking the question "Does Deva have hair?" in the above scenario the computer would respond "I don't know." because Hair is one level too deep in the recursion.
So what I would do is have it so anytime you asked a question that was in range, but was deeper than level 1 if it found an answer at level 2 or 3 it would move that answer to level 1. This would shift the entire tree closer. Though this was more than a tree it quickly becomes an interconnected web.
Let's ask "Is Deva a Mammal?" which is 3 deep. It would respond "Yes" or in some affirmative way. Yet the system would have found this at a deeper level. So it will as an "Is mammal" definition value to Deva. So now that is a layer 1 reference and all definition values on Mammal are now shifted up appropriately.
This could simulate learning. It actually worked pretty well.
It did however, offer an opportunity with an experiment tied in with the previously mentions boredom.
Dreaming
I tapped into the boredom routine and made it so if sufficient time passed with no interaction from the player the computer would shift into a dream state until the player interacted again.
In this state it would pick random word X, random relationship R, and random word Y making sure X and Y were always different.
IS Deva a Reptile?
It would begin asking itself all manner of random questions. And they could end up being quite bizarre conversations. Sounds kind of like some of my dreams.
Source: giphy.com
Now that you know the structure above and how the recursion works, can you picture what this dreaming did?
Anytime it his answers that were not at level 1 it would shift those results to 1. The result was as it dreamed it formulated far deeper definitions of words than what the initial input provided it.
It could learn relationships you never told it. It actually worked and was pretty cool.
My ideas I never pursued
I had a lot of grand ideas based upon the experiments above. Each one became far more complex than the next.
My next goal was to have VERB, ADVERB, POSSESSIVE, PRONOUN, MALE, FEMALE, NEUTRAL, NOUN, ADJECTIVE, PERSON, PLACE, THING, PLURAL, etc setup as pre-defined traits, as well as some other grammatical useful types.
I planned on being able to teach it sentence structures so it could attempt to guess at the nature of words it did not know based upon position within sentences. I would also have it construct responses using words and sentence formats based upon the logic it had. When it didn't have enough information I could initially fall back on the techniques used in the tutorial 4 in this series until it had sufficient information to stand on it's own.
Source: giphy.com
If something like this worked then it could read text documents, web pages, etc and build up its knowledge that way.
Down the rabbit hole
The more you delve into this the more you begin to wonder about wisdom, intuition, common sense, etc. The logic, math, and science parts of things are easy to simulate. Some things are rather nebulous.
You also have trained your computer to deal with people who use proper spelling, grammar, and speak the same language. How do you train it when all of these things differ? It can get very complex, very fast.
Source: giphy.com
It can be quite fascinating...
Practical Gaming Applications
The parsing of text is used in many old school text adventure games. It was also used in MUDs and other text input based games. It is still useful for that.
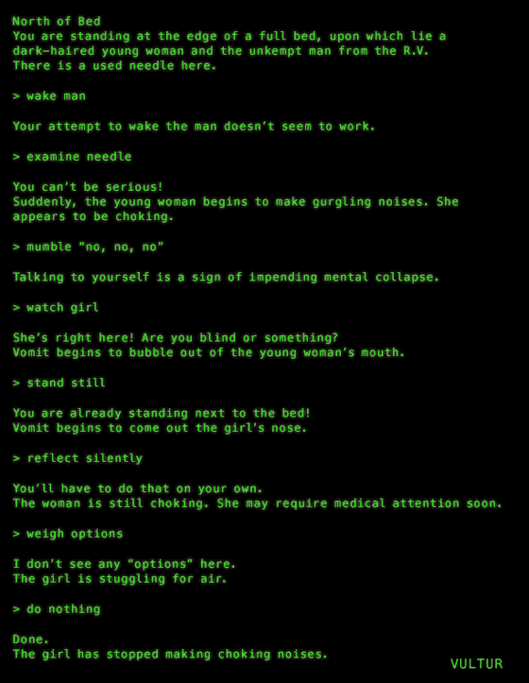
Source: giphy.com
You could take it further though. What if you wanted to make NPCs in a Role Playing or Adventure game that you actually spoke to rather than using canned responses? You could do that using a system like this.
I made a system for setting up different personalities within Bioware's Neverwinter Nights game that did something like this. I also had accent and drunk filters. If I wanted to add an accent I would simply run the response to be sent to the player through an accent filter to add some tweaks to the output before sending it to the player. The Drunk filter depending upon drunkeness would appropriately add tweaks to slur words... :) Exaggerate S, and take advantage of some SH sounds where appropriate.
Source: giphy.com
There is a use for this in gaming if you wish to do something like this. WARNING: This does alienate some players who are not good at typing, or conversing. It is thus generally not a good idea if you want to target the widest audience possible.
If you do use such a system it is a good idea to have it HIGHLIGHT keywords of interest in the NPC responses. This way the player knows what words they can use that the player might respond to. I believe the old Ultima series did this some beginning with Ultima IV.
Source: giphy.com
Conclusion
In this tutorial series we scratched the surface of one approach to building something like this. Making something that can truly pass the Turing Test is daunting. It is a fun challenge. You probably can see that such a program would be vastly more code than in this tutorial. It also would not be feasible to do a tutorial on attempting something at that degree. Instead, I hope I have opened the door for you and you now have some new ideas and tricks in your skillset.
I find that pursuing this profoundly impacted how I view the mind and a lot of philosophical ideas as well. It is also one reason I am not particularly concerned about us being replaced completely anytime soon. We could make an intelligence based purely on logic, math, and science fairly quickly. That could potentially supplant us, but we could not replace us with an equivalent in terms of wisdom, common sense, or intuition any time soon as far as I am able to tell. I do attempt to keep up with the field somewhat, but it's been awhile since I checked on the latest Turing Test participants.
Thank you for reading my tutorials. Your up votes are appreciated. It is how I gauge interest.
Steem on!
It's already been few weeks?! I missed your posts between, but upvoted them as I voted for this topic. And I agree with you; the theory parts were probably the most interesting ones, but it also was nice to see the code. For more consumer friendly topic, I guess the voxel sandbox rpg would be ideal? But I'm sure any subject you decide to post on and give full attention will be worth our time.
It's a toss up between cubic voxel setting, or writing a sprite system for use in games like roguelikes and such that does the sprites itself and is easy to add/update sprites, support lighting and normal maps, and such. Those are the two I am kicking around. I'll likely post on some other topics for a few days before diving back into game dev posts. I like to post on a lot of different things. If I don't then my posts do progressively worse as I release them too quick.
Both sound intriguing. I'll look forward to what you come up with.
I already made a mock up awhile ago of how people would intuitively think to approach a cubic voxel world in Unity in anticipation of someday doing such a tutorial. It gives me a chance to show you WHY you don't likely want to go that route. :)