[Unity Game Development Tutorial: 11 : Turing Game: 2] - Getting input, output, and simple parsing working
The Steemit community voted for me to do a Turing Test type game. This is the second installment in that topic. The first can be found by clicking here.
This tutorial continues where the previous tutorial left off and assumes you have a project started.
ALTERNATIVELY: You can download a google drive zipped copy of the project created for the last tutorial here.
Setting up basic input and output
In the previous tutorial we created all of the UI elements we are likely to need for this game. We left them at default Unity UI appearances, but you are welcome to swap out the sprites and textures and make yours look however you like.
In this section we will make it so you input in the text field, and it adds your output to the scrolling text area. It will also send whatever was input to a method we will use to break the sentence down and prepare it for usage in the games responses. This is known as parsing, and since we will react to specific occurrences of words it also sometimes has similarities to what is known as tokenizing.
Let us begin. We will assume you have done the previous tutorial or some of my other Unity Tutorials and therefore will be familiar with some steps. This will enable me to skip some steps.
TuringBrain.cs
Let's create a script called TuringBrain.cs and open it up in the editor. We are going to make it look like this initially.
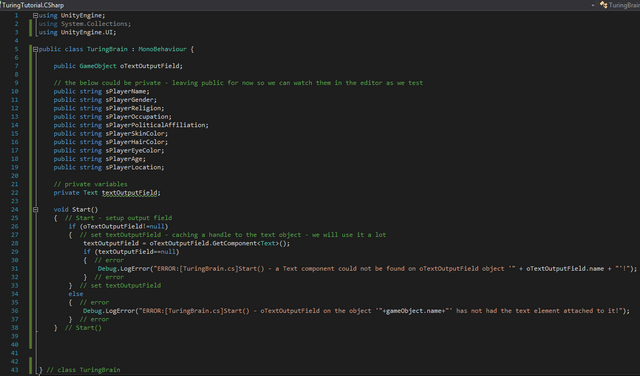
Other than the oTextOutputField public variable at the top, the rest are public variables simply because we plan to use them in the near future, and while they normally would be private for something like this we will leave them public so we can see how they change in the editor when we are testing them. This should be a normal trick you use in Unity. Feel free to set variables public so you expose them to the inspector in the editor. Once you no longer need to see them in the inspector set them back to private. This can be very useful for troubleshooting.
The next part is that we use the Start() event to cache handles to components on the Text Output area of the UI. We also supply some error checking so it gives errors if it is not setup properly.
Let's drag that script onto the Canvas object in the hierarchy.
Then drag the TextScrollArea from the hierarchy onto the slot O Text Output Field on the inspector for Canvas.
Let's now add a public method to the script that is empty at the moment. We simply need it there so we can properly attach it to the UI.
- Click on the InputField in the hierarchy.
- Click on the + icon in the On End Edit (String) in the inspector.
- Drag the Canvas object into the box below the Runtime Only drop down
- In the drop down to the right of the Runtime Only drop down select TuringBrain and then the TextHasBeenEntered method we created above.
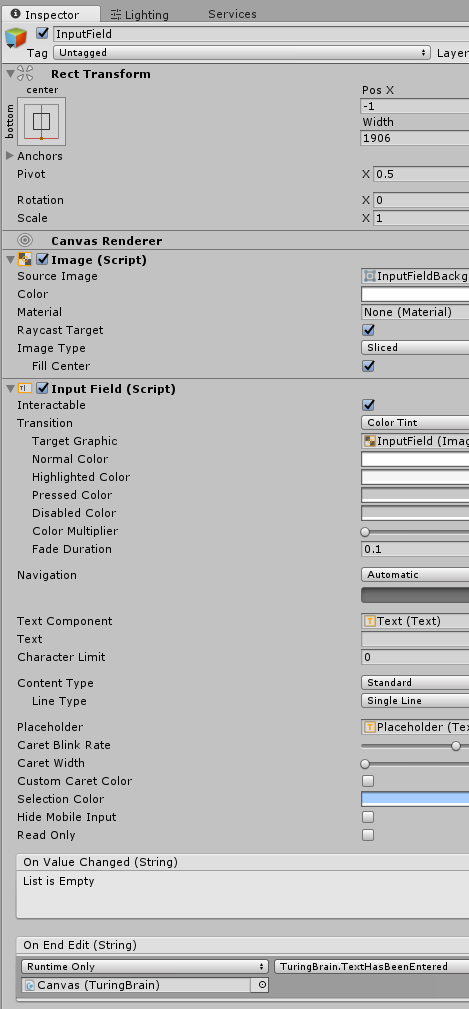
The UI now has a link to the code.
Accelerating the steps a bit... as you are learning new things it frees me to go faster.
Code going to talk about
- We add a new private called inputInputField and drag InputField from the hierarchy onto it.
- We add a new section to cache a handle to InputField within the Start() event complete with basic error checking.
- We create an empty HandleInput(string sInput) method that will handle using the input for something.
- We create an OutputText(string sOutput) method and set it up to append text to the end of the scrolling text area.
- We modify the TextHasBeenEntered() method that had existed from previous steps so that it deals with the text that was input by clearing the input field, sending a message to OutputText(), and calling HandleInput()

Go ahead and try it out
Here is my experiment.
Seems like a lot of work for simple input/output.
Yes, this is quite a lot more work than a simple IO program would be at a command line. Unity is a full fledged game engine. It is designed to handle complex 3D and 2D work. Now that we have this working we could quickly and dramatically change at any time we felt the need how it appears on the UI, what it is attached to, etc simply by dragging some things around, swapping some sprites or textures, etc. It is a bit of an awkward setup to get to this point, but once you are at this point it is quite powerful.
Parsing, Tokenizing
Now we need to break that input up into something we can use. This is the last sections we will be working on that are tedious. Once we have this section done we can move onto the interesting part. Things should begin to get fun and progressively theoretical and mind adventure like from that point on.
First Let's add two string arrays to the public variable section of variables we can monitor at run time.
This array will contain the words after we break them down. We want both upper and typed in case versions. We convert them at the beginning because you will reference these fields often so by doing this at the beginning we actually reduce complexity going further.
Second let's add a method for splitting the typed in text into words.
The delimiters indicate which characters should be treated as word breaks. What should determine where a word break ends, and begins. This is basic and we will become more complex in the future. There are some messy factors in this when typing in multiple sentences. We will deal with that in a future tutorial. For now we are focusing on getting the basics working.
Third we now modify the HandleInput() method from earlier to call split and then also create an upper case version of what split returns.
At this point your code should look like this.
Try it out and check out the arrays in the inspector as you try typing things. Here are the results when I type "I am Deva."
Ready for the Fun Stuff
This was the bulk of what I intended to do in this tutorial. I don't want to leave you completely dangling though. I will give you a little bit of a taste of what is to come.
Let us simply deal with me telling the computer what my name is by saying "I am X", or "I'm X". There are far more complexities than this to making a Turing Test worthy product, but you have to start somewhere.
First we will modify the HandleInput() method some more.
Second we will create a HandleIAm() method.
Now let's try it out.
Bottom Code Section: Easier to read version
Conclusion
Obviously this is far from perfect. This field has some very deep corners. We will not reach all of them, but we can take it much further than this. If you liked this tutorial and wish me to continue and take it further and further then please give me an up vote.
This tutorial offered you some basics so you could get something like Eliza working fairly quickly with just the information I have already provided.
Steem On!
EDIT: I created a purely theoretical post (no code) to act as the glue between this tutorial and the next coding tutorial. It will explain why we are doing some of what we end up doing in code. I also took a moment to link to all coding related tutorials I posted if you need help from any of those, as well as my glossary, and coding for beginners tutorials made by @charlie.wilson. That post is here.
Keep up the great work!
Tweeted and here's the short link for this post for anyone to use: http://steem.link/MKtbX