Learning Python with Steem - The Winfrey Bot
Important:
I'm just starting to learn Python and I'm not a developer.
Use the code below entirely at your own risk.
I'm slowly learning how to code with Python 3.
I took this bot apart and put it back together and - for the most part - understand how it works now:
It's very motivating to see your code doing something useful instantly.
Coding Python with Piston for curation, can be very rewarding; Mentally and monetarily.
As I learn new stuff from programming tutorials, I try to use them in my bot.
I'm sure I made some mistakes, but this is mainly an exercise for me.
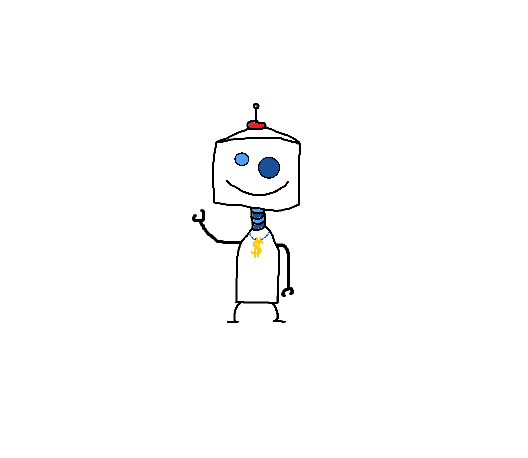
It will need 4 files in the same directory to work:
accounts.txt | wif.txt | authors.txt | upvote_history.txt |
---|---|---|---|
account1 | 5wifkeyreallylong | goodauthor1 | |
account2 | 5wifkeyevenlonger | goodauthor2 |
votebot.py:
import threading
import random
import time
import csv
from time import localtime
from steem.steem import Steem
from steem.steem import BroadcastingError
vote_delay = 1740
def feed():
authors= list_load('authors.txt')
upvote_history= list_load('upvotehistory.txt')
steem = Steem(wif=posting_key)
for comment in steem.stream_comments():
if comment.author in authors:
if len(comment.title) > 0:
if comment.identifier in upvote_history:
continue
upvote_history.append(comment.identifier)
list_save('upvotehistory.txt', upvote_history)
print(timestamp_builder(), comment.identifier, \
": thread started, vote in ", vote_delay, " seconds ...")
workerThread = threading.Thread(name=comment.identifier, \
target=worker, args=(comment,))
workerThread.start()
def worker(worker_comment):
time.sleep(vote_delay)
try:
for (k,v) in enumerate(account):
print(timestamp_builder(), worker_comment.identifier, " <--- voted ",v)
worker_steem = Steem(wif=posting_key[k])
upvote_comment = worker_steem.get_content(worker_comment.identifier)
upvote_comment.vote(100, v)
except BroadcastingError as e:
print(str(E))
def list_save(listfile, listvar):
with open(listfile, 'w') as writestuff:
writestuff.write('\n'.join(listvar))
writestuff.write('\n')
def list_load(listfile):
with open(listfile, 'r') as readstuff:
listvar= []
reader= csv.reader(readstuff)
for rows in reader:
v= rows[0]
listvar.append(v)
return listvar
def timestamp_builder():
lt = localtime()
timestamp = time.strftime("%d/%m/%Y-%H:%M:%S ", lt)
return timestamp
account= list_load('accounts.txt')
posting_key= list_load('wif.txt')
if __name__ == "__main__":
while True:
try:
feed()
except (KeyboardInterrupt, SystemExit):
print("Quit")
break
except Exception as e:
print("Exception ... Restart")
traceback.print_exc()
Here is an example of the printout:
10/01/2017-19:18:35 @pgarcgo/proyecto-cervantes-compensacion-y-reconocimiento-para-escritores-de-habla-hispana-vol-106 : thread started, vote in 1740 seconds ...
10/01/2017-19:47:35 @pgarcgo/proyecto-cervantes-compensacion-y-reconocimiento-para-escritores-de-habla-hispana-vol-106 <--- voted votebot
10/01/2017-19:47:38 @pgarcgo/proyecto-cervantes-compensacion-y-reconocimiento-para-escritores-de-habla-hispana-vol-106 <--- voted deutschbot
Additions:
- input/output functions to store accounts, wifs, authors, upvote_history in txt files
- timestamp function
- better handling for multiple accounts
Also, I changed when upvote_history
gets updated.
This way, the bot won't start mutiple threads if the author edits his post before the bot voted.
upvote_history
gets stored in a file now, so when the bot crashes and restarts, it won't try to upvote a post again.
This is still far from ideal.
It's still very basic and I don't think it's a good approach to curation in general.
I did it for the exercise and learned a lot.
Ideally, I would need a steem.stream_votes()
function, similar to steem.stream_comments()
Next steps will be databases and maybe how to use a proper config file.
If you add the cheetah/steemcleaners snippet from this bot, they will be happy :)
https://steemit.com/upvote-bot/@fyrstikken/updated-winfrey-upvote-bot-will-vote-for-everyone-who-post-on-steemit-with-a-2-upvote-except-posts-flagged-by-cheetah-or
oh, and also - comment what the different parts of the code is doing, easier for others to build on top of it :)
good work, I´ll give it a run soon.
That was just meant to record my recent progress in Python.
If I have something more interesting, I will comment the code more.
This is really amateurish , I know :)
I like it - keep playing with it :)
@felixxx you're so humble - if you call this amateurish - I call it magic.
You have an awesome brain and that bot looks cute!
I tried learning coding in Phyton - from po0 I guess that was the author's name but I got busy so I forgot about it and I don't remember the author's name even sighs.
Its fun to code specially when its coming out right but its not just fun - maybe cause am not a comp genius - so it makes my head hurt hahahaha
That's awesome man. Thank for sharing improvements with everyone :)
The information in your post is very useful, Felix!
Thank you for sharing with us.
Upvoted & Followed.
made a mistake :(
Very nice info
Can you tell in few simple words how threading works in python?:)
I just copied all that from here:
https://steemit.com/socialist-bot/@fyrstikken/the-anonymous-winfrey-bot-upvotes-for-everyone-download-here-easy-steps-for-n00bs
This is the threading bit:
That's it.
normaluser, please show my sp !
felixxx, your sp: 6452.223378970239 !
normaluser, please show my @elyaque -badges !
follower-badge:
http://i.imgur.com/LkkVhpJ.png
reputation-badge:
http://i.imgur.com/3AiPO9E.png
postcount-badge:
http://i.imgur.com/LgZhbd8.png
@normaluser, please show my @elyaque -badges !
@felixxx, your @elyaque -badges:
follower-badge:
http://i.imgur.com/LkkVhpJ.png
reputation-badge:
http://i.imgur.com/3AiPO9E.png
curation-badge:
http://i.imgur.com/qQHBITS.png
postcount-badge:
http://i.imgur.com/LgZhbd8.png
steempower-badge:
http://i.imgur.com/0hyC7hb.png
Find all @elyaque -badges here.
This is so fun and exciting. Here goes the really amateurish question.
This is done on your normal computer? Any operating system? No special hardware and software requirements?
Thank you for this post and for any reference you can give to a real newbie. Old man, but a complete newbie.
I code my scripts on a desktop pc and once I'm done move them to an old laptop, which runs 24/7 as a server.
Operating system: Ubuntu ( or another Linux )
The hardware requirements for the bot above are minimal; a raspberry pi would do.
Thanks so much. I´ll keep moving.
hey felix, sorry to bump, do you have any other scripts for steem-python library like rewardinator one, thanks again :)
I have some ... What exactly are you after ?
for example to upvote my fav authors based on category posted , upvote only newly created posts by my fav authors , something like that, i tried this https://steemit.com/howto/@felixxx/howto-steemit-bot-tutorial-for-newbies-1-votebot but it gave no post found error
Hmm ... Piston is pretty much obsolete by now.
Steem-Python should work ...
I don't use vote-bots, but they are easy to make. I just don't think they're good for Steemit ...
are there any scripts for steem-python for same?