[Java Tutorial #8] - How to create SP to Vests Converter Calculator Aplication using Netbeans
Repository
https://github.com/dmlloyd/openjdk
What Will I Learn?
- You will learn How to create GUI form for this aplication
- You will learn API to convert SP to VEST
- You will learn how to get data from user input and multiply it with the price from API URL
- You will learn how to display last data to the form
Requirements
- Basic knowledges about java programing
- Basic Knowledges about API
- Need to Install Netbeans for easy pratice
Difficulty
- Basic
Tutorial Contents
In this tutorial, I will try to explain how to make a calculator application to convert steem power to vest. The user inputs the amount of steam power and the system will issue amounts in the vests. Here is what the app looks like:
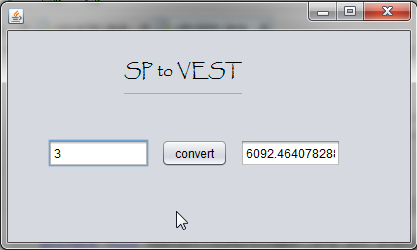
Let's start the tutorial :
Open Netbeans IDE software and create new project. Choose Java Aplication and click next. Then Rename the project and click finish.
Add new jFrameForm in your Source Package
Click on panel component and drag it to the form
Add Label, TextField and Button on Panel, just follow the components on picture bellow :
We already have a form, Now open
source
and start to edit the code.Create The method to get Data from URL. This method in
java.net.*
method. So before your start use this method import the package first
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.logging.Level;
import java.util.logging.Logger;
- Then create a method to read url from web
public String getApi(String url) throws Exception{
URL oracle=new URL(url);
HttpURLConnection httpcon = (HttpURLConnection) oracle.openConnection();
httpcon.addRequestProperty("User-Agent", "Mozilla/5.0");
BufferedReader in = new BufferedReader(new InputStreamReader(httpcon.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null){
return inputLine;
}
in.close();
return null;
}
Code | Explanation |
---|---|
getApi() | Method Name |
String url | The Parameter |
throws Exception | Some method to catch error |
URL oracle=new URL(url); | Decrlarating new URL |
HttpURLConnection httpcon = (HttpURLConnection) oracle.openConnection(); | class initiation to get URL connection. |
httpcon.addRequestProperty("User-Agent", "Mozilla/5.0"); | Add browser property, Here I add Mozilla 5.-0 |
BufferedReader in = new BufferedReader(new InputStreamReader(httpcon.getInputStream())); | method Read input from http connection. |
return inputLine; | return the output |
in.close(); | close input stream reader |
- Now create a method to count vests in Steem power
public void calc() throws Exception{
String Data = getApi("https://uploadbeta.com/api/steemit/converter/?cached");
JSONObject ObjData=new JSONObject(Data);
vest.setText(""+Double.parseDouble(sp.getText())*ObjData.getDouble("sp_to_vests"));
}
Code | Explanation |
---|---|
calc() | Method Name |
getApi | we call the previous method to get Data from URL. and the parameter is URL from https://helloacm.com/tools/steemit/ |
JSONObject ObjData=new JSONObject(Data); | convert the result from string to JSON Object. Here we need use java-json library. Dowload it here : http://www.java2s.com/Code/Jar/j/Downloadjavajsonjar.htm . Extract and add to project Libraries. Then import it to your file, then import org.json package |
vests.setText | setText method for vest convert result |
Double.parseDouble(sp.getText()) | get double value from user input |
ObjData.getDouble("sp_to_vests") | get sp price in vests from object data API |
""+ | The Variable that will be set as text in componen, because the variabel type is double we need combine with a string. So I use ""+. |
- Call the
calc()
method in convert button. Open the aplication design form and double click on button. then add this code.
private void convertActionPerformed(java.awt.event.ActionEvent evt) {
try {
calc();
} catch (Exception ex) {
Logger.getLogger(calculator.class.getName()).log(Level.SEVERE, null, ex);
}
}
save all code, and try to run the app
Finish, the aplication is running rightly. You can get the full code on my gist. The link is bellow
Curriculum
- [Java Tutorial] - How to create some java aplication to display Live SBD price from bittrex API using Netbeans
- [Java Tutorial #2] - How to create GUI aplication for displaying Live SBD price using Netbeans
- [Java Tutorial #3] - How to create GUI java aplication for displaying Steemit account data using Netbeans
- [Java Tutorial #4] - How to create Calculator java aplication to convert STEEM to IDR using Netbeans
- [Java Tutorial #5] - How to create GUI java aplication for checking The Estimated Reward in Last Week using Netbeans
- [Java Tutorial #6] - How to create GUI java aplication for checking STEEM POWER DETAIL using Netbeans
- [Java Tutorial #7] - How to create Converter Calculator Aplication for STEEM and SBD using Netbeans
Proof of Work Done
https://gist.github.com/team2dev/a5f030cd6f5a78b460ca248786da0937
@team2dev can you also publish java tourials for beginners which helps many newbie's.
yes, you can request me what tutorial you need and i will post it
Like Java containers and all
Thank you for your contribution.
What you did here is really basic. You simply called the API which is already readily available, and multiplied the number by the relevant JSON entry.
Similar content is easily available online, especially via the original creator of the API itself.
I would suggest to come up with more innovative, challenging, and not easily available ideas.
Also the word application is written with double "p", as you have it incorrect all over.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @team2dev
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!