[Java Tutorial] - How to create some java aplication to display Live SBD price from bittrex API using Netbeans
Repository
https://github.com/dmlloyd/openjdk
What Will I Learn?
- You will learn How to get data from URL API
- You will learn how to Display data in Java
- You will learn how to split data and dislay ony the important data
- You will learn about JSONObject
Requirements
- Basic knowledges about java programing
- Basic Knowledges about API
- Need to Install Netbeans for easy pratice
Difficulty
- Basic
Tutorial Contents
In this occasion, I will try to create a tutorial how to get data from bittrec API and display it in my JAVA aplication. For Sample, I wanna to display LIVE SBD price. You can try for other criptocurrency.
In Java Programming, To get data from some URL we can use net.URL package. By using some functions, We can grab all data from API url and display it in our Aplication. Lets start the tutorial :
Open Netbeans IDE software and create new project. Choose Java Aplication and click
next
Rename the project and click Finish
Open the java file on editor and start to code.
Import net-URL package
import java.net.URL;
import java.net.URLConnection;
import java.nio.charset.Charset;
These all package used to grab data from some URL in web. These package contains some class and some java method to open URL connection and to grab some data from The URL.
- import BufferedReader and IOException package. These package used to read input url and handle the error
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
- Create getData method. This method is to grab data from api URL. The Explanations under the code :
public static String getData(String apiURL) {
System.out.println("api URL:" + apiURL);
StringBuilder sb = new StringBuilder();
URLConnection urlConn = null;
InputStreamReader in = null;
try {
URL url = new URL(apiURL);
urlConn = url.openConnection();
if (urlConn != null)
urlConn.setReadTimeout(60 * 1000);
if (urlConn != null && urlConn.getInputStream() != null) {
in = new InputStreamReader(urlConn.getInputStream(),
Charset.defaultCharset());
BufferedReader bufferedReader = new BufferedReader(in);
if (bufferedReader != null) {
int cp;
while ((cp = bufferedReader.read()) != -1) {
sb.append((char) cp);
}
bufferedReader.close();
}
}
in.close();
} catch (IOException e) {
throw new RuntimeException("Exception while calling URL:"+ apiURL, e);
}
return sb.toString();
}
Code | Explanation |
---|---|
System.out.println("api URL:" + apiURL); | Print the API URL |
StringBuilder sb = new StringBuilder(); URLConnection urlConn = null; InputStreamReader in = null; | Instance Class Object. |
if (urlConn != null) urlConn.setReadTimeout(60 * 1000); | set time for timeout when the URL is not empty |
in = new InputStreamReader(urlConn.getInputStream(), Charset.defaultCharset()); | restore the url as input stream |
new BufferedReader(in); | Read the URL to grap or get Data |
while ((lengthData = bufferedReader.read()) != -1) { sb.append((char) lengthData);} | Displaying data in while looping. this looping start from the index 0 until the end. |
- Create the main class to display the data got from URL API. Call the getData class that we have created before. Then add some bittrex API url. you can visit : https://support.bittrex.com/hc/en-us/articles/115003723911-Developer-s-Guide-API for more URL
public static void main(String[] args) throws JSONException {
final JSONObject obj = new JSONObject(getData("https://bittrex.com/api/v1.1/public/getmarketsummary?market=btc-sbd"));
System.out.println(obj);
}
Becouse we use JSONObject here we need to add java-json Library. Dowload it here : http://www.java2s.com/Code/Jar/j/Downloadjavajsonjar.htm . Extract and add to project Libraries.
import the JSONObject package
import java.nio.charset.Charset;
import org.json.JSONException;
import org.json.JSONObject;
import org.json.JSONArray;
save the file and try to run the project
We already get data from URL as JSON. In case we don't need all data. We want just display market-name, timestamp and last-price. SO, now we need to split the JSON data.
final JSONArray result=obj.getJSONArray("result");
final int n = result.length();
for (int i = 0; i < n; ++i) {
final JSONObject person = result.getJSONObject(i);
System.out.println("Cryptocurrency :"+person.getString("MarketName"));
System.out.println("Real Time"+person.getString("TimeStamp"));
System.out.println("Last Price"+person.getDouble("Last"));
}
Code | Explanation |
---|---|
final JSONArray result=obj.getJSONArray("result"); | Get array value of result. |
final int n = result.length(); | get result lenght or get data count. |
for (int i = 0; i < n; ++i) | looping for here used to display data one by one. |
final JSONObject person = result.getJSONObject(i); | istance the class |
person.getString("MarketName") | get data from marketName. why we use getString method ? because the value is from string type. |
Save and try to run
Finally we Already split JSON data. What is the problem now. See on the result of lastprice, it display in Double type. If we need to convert it to decimal, what can we do ? we need to add
BigDecimal()
method like this:
System.out.println(BigDecimal.valueOf(person.getDouble("Last")));
- Because
BigDecimal()
is from java.math.BigDecimal package, so you need to import this package :
import java.math.BigDecimal;
Now, Try to run the project .
You can see now the Last Price is display in Decimal. Now try to display data in Sentence
System.out.println("The Last Price of "+person.getString("MarketName")+" is : "+BigDecimal.valueOf(person.getDouble("Last")));
System.out.println("Real Time :"+person.getString("TimeStamp"));
- FINISH. We already got data from URL API and display it as our plan. You can try to get dara from other URL API to make you more understand.
Curriculum
This is My first tutorial contributin about JAVA
Proof of Work Done
https://gist.github.com/team2dev/c466b39cd707e9486982c09090874432
Thank you for your contribution.
While I liked the content of your contribution, I would still like to extend few advices for your upcoming contributions:
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank a lot ser for your feedback and you great advice.
Hey @team2dev
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
اللہ ایک ہے جس کے پاس اس کا شریک نہیں ہے. اس کے پاس کوئی اولاد نہیں ہے. یہ محافظ اور برقرار رکھنے والا ہے. وہ ہر چیز پر طاقت رکھتے ہیں.
Join Coinbase and get $10 (€8) of free Bitcoin when you first buy or sell $100 (€86) of digital currency.
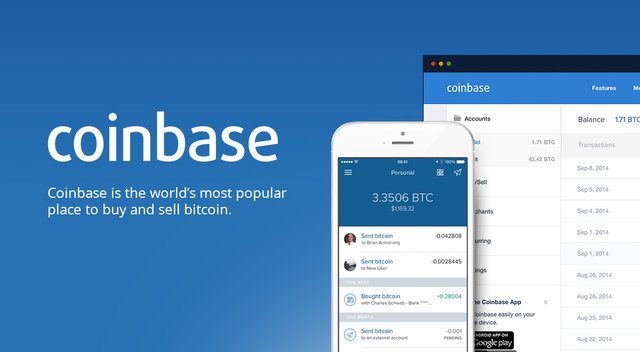
https://www.coinbase.com/join
Your contributtion is good enough, I want to give you some advice:
Try to take a closer screenshots. It is quite difficult to read some of your screen shots.
Don't forget to add resources to get more points in your contributions
Add more required items for the requirements section.
I hope this advices are helpful. Let me know if this is helpful.