[Java Tutorial #2] - How to create GUI aplication for displaying Live SBD price using Netbeans
Repository
https://github.com/dmlloyd/openjdk
What Will I Learn?
- You will learn How to get JSONdata from URL API
- You will learn how to split data and dislay ony the important data
- You will learn how to display result on GUI form
Requirements
- Basic knowledges about java programing
- Basic Knowledges about API
- Need to Install Netbeans for easy pratice
Difficulty
- Basic
Tutorial Contents
In the previous tutorial, I've explained how to retrieve data from API URLs using JAVA programing. And on this occasion, I will continue the previous tutorial that is how to display data into GUI form on java application
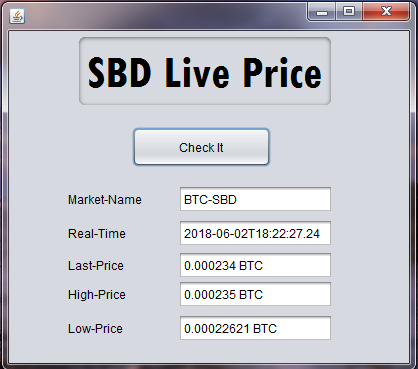
Let's Start :
Open Netbeans IDE software and create new project. Choose Java Aplication and click
next
. Then Rename the project and clickfinish
Add a jFrameForm in your Source Package
Open the java file on editor and start to code.
Rename the Form
Now Start to create a form. Just Follow the Components bellow :
number : 1. jLabel
number : 2. jLabel
number : 3. jButton
number : 4. jTextField
We already have a form that contain a button and 4 jTextField. When the button clicked the data will display in jTextfied. Now open
source
and start to edit the code.
Add getData method, This method i heve explained also in my previous tutorial. see here for more detail
public static String getData(String apiURL) {
StringBuilder sb = new StringBuilder();
URLConnection urlConn = null;
InputStreamReader in = null;
try {
URL url = new URL(apiURL);
urlConn = url.openConnection();
if (urlConn != null)
urlConn.setReadTimeout(60 * 1000);
if (urlConn != null && urlConn.getInputStream() != null) {
in = new InputStreamReader(urlConn.getInputStream(),Charset.defaultCharset());
BufferedReader bufferedReader = new BufferedReader(in);
if (bufferedReader != null) {
int lengthData;
while ((lengthData = bufferedReader.read()) != -1) {
sb.append((char) lengthData);
}
bufferedReader.close();
}
}
in.close();
} catch (IOException e) {
throw new RuntimeException("Exception while calling URL:"+ apiURL, e);
}
return sb.toString();
}
Code | Explanation |
---|---|
StringBuilder sb = new StringBuilder(); URLConnection urlConn = null; InputStreamReader in = null; | This code to Instance Class Object. |
if (urlConn != null) urlConn.setReadTimeout(60 * 1000); | set time for timeout when the URL is not empty |
in = new InputStreamReader(urlConn.getInputStream(), Charset.defaultCharset()); | restore the url as input stream |
new BufferedReader(in); | Read the URL to grap or get Data |
while ((lengthData = bufferedReader.read()) != -1) { sb.append((char) lengthData);} | Displaying data in while looping. this looping start from the index 0 until the end. |
- As I have explained in previous tutorial, to get data from url and to use BufferedReader we should import net-URL, BufferedReader and IOException package.
import java.net.URL;
import java.net.URLConnection;
import java.nio.charset.Charset;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
- Create a method to display data on the form
void display() throws JSONException{
final JSONObject obj = new JSONObject(getData("https://bittrex.com/api/v1.1/public/getmarketsummary?market=btc-sbd"));
final JSONArray result=obj.getJSONArray("result");
final int n = result.length();
for (int i = 0; i < n; ++i) {
final JSONObject person = result.getJSONObject(i);
marketName.setText(person.getString("MarketName"));
realTime.setText(person.getString("TimeStamp"));
lastPrice.setText(BigDecimal.valueOf(person.getDouble("Last")).toString()+" BTC");
highPrice.setText(BigDecimal.valueOf(person.getDouble("High")).toString()+" BTC");
lowPrice.setText(BigDecimal.valueOf(person.getDouble("Low")).toString()+" BTC");
}
}
Code | Explanation |
---|---|
display() | Method Name |
throws JSONException | This code to handle the error from JSON |
final JSONArray result=obj.getJSONArray("result"); | Get array value of result. |
marketName.setText(person.getString("MarketName")); | this is the main code of this tutorial, it is to display the result on jTextField. |
BigDecimal.valueOf(person.getDouble("High")) | Convert the Double to Decimal |
toString() | because in jTextField component just can use String Type, so here we need to convert it to string. |
- TO use JSONObject here we need to add java-json Library. Dowload it here : http://www.java2s.com/Code/Jar/j/Downloadjavajsonjar.htm . Extract and add to project Libraries. Then import it to your file.
import java.nio.charset.Charset;
import org.json.JSONException;
import org.json.JSONObject;
import org.json.JSONArray;
The last one you need to import
import java.math.BigDecimal;
for BigDecimal package.The last step is call the
display()
method in jButton. Open the design and doble click on button.
Add this code to call the
display()
method.
try {
display();
} catch (JSONException ex) {
Logger.getLogger(form.class.getName()).log(Level.SEVERE, null, ex);
}
The code above is for calling the
display()
method. We call this method in try and catch to handle the error.
- Finally, Save and try to run the project.
- Then click the
check it
button on the form. And you will see the data will display rightly.
Curriculum
Proof of Work Done
https://gist.github.com/team2dev/bcac8e443d3d69de7803cc503d55d32e
Thank you for your contribution.
While I liked the content of your contribution, I would still like to extend few advices for your upcoming contributions:
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank a lot bro, I will do my best for next
Hey @team2dev
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Nice tutorial,
I would like to suggest you to change the the word "Netbeans" from the tiltel to "Java" since you are using Java as the underlying technology to perform your operations.
Also please explain the code in more details or use code comments if you think explicit explanation is not needed. Always explicitly state a little details about the external libraries being used.
Good luck and Keep contributing to the community.
Its not tiltel its title
I am very interesting about this post. #almumu steemians new in this site peace from #indonesia
and make sure to join our Discord Server
and follow @steemkitchen for more curated