Managing particle physics model information in Python
Particle physicists currently explore the fundamental laws of Nature through very powerful machines, the LHC at CERN being one of them. The good accomplishment of this task crucially depends on our ability to implement, in appropriate simulation tools, any model physicists could dream of. This would indeed allow us to draw conclusive statements from theory-data comparisons.
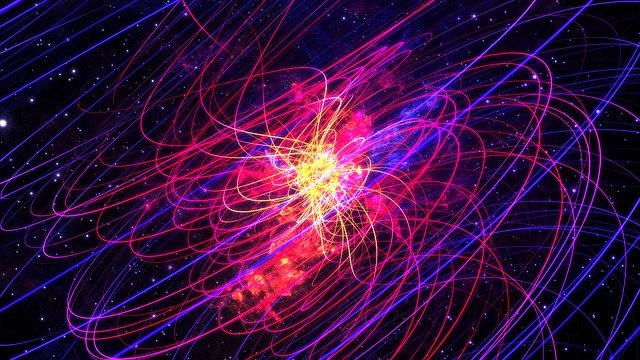
[image credits: GamOI (CC0)]
A week ago, I proposed to run a full particle physics research project on Steem in this context. The aim is to design a Python program allowing for inputting any particle physics model in the simulation tools.
Thanks to a joint effort from @steemstem and @utopian-io, we are here today, ready to start working.
The first part of this project consists in designing a data format to store the information that will have to be inputted by the users. As the amount of inputs is pretty large, I decided to organize the project in terms of one Utopian sub-task per type of inputs. The topic of the day consists in the treatment of the model parameters.
EXTERNAL AND INTERNAL PARAMETERS
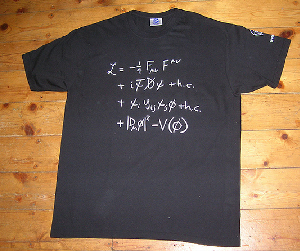
[image credits: ArXiv]
As explained in my previous post, the entire information on a particle physics model is included in an equation called a Lagrangian.
Such a Lagrangian is in generally compact, and for the Standard Model, it even fits on a t-shirt, as illustrated with the picture. Note that this t-shirt can be bought at the CERN shop.
Now let us go back to the Lagrangian. It contains various things, that include parameters. This is what we will talk about today.
In any typical particle physics model, we have two types of parameters, namely external and internal parameters.
- External parameters are real numbers for which the user must provide a numerical value.
- Internal parameters can be either real or complex, and are connected to other parameters via an analytical formula.
An illustrative declaration of external parameter is provided below in the good old FeynRules syntax (which we want to adapt for the needs of this project). The parameter that is chosen as an example is named aS
.
aS == {
ParameterType -> External,
Value -> 0.1184,
InteractionOrder -> {QCD, 2},
BlockName -> SMINPUTS,
OrderBlock -> 3
}
I guess the meaning of each attribute of this aS
parameter is almost straightforward. Please ignore the Mathematica syntax and mentally convert it into a future Python syntax.
A remark is in order concerning three attributes. The interaction order is an optional property. It indicates the nature of a parameter with respect to the fundamental interactions embedded in the model. Here, the aS
parameter has the same strength as the square (this is the 2) of the coupling strength of the strong interaction (this is the QCD part). Whilst QCD and QED are reserved keywords with very well defined meanings, the user is actually free to use anything.
All external parameters are then organized in groups (or blocks... yes, this is not invented, see here) and each entry in a block connects a counter (that consists in one integer) and a value.
It is now the time to move on with internal parameters. In the FeynRules syntax, the declaration of an internal parameter (named gs
for the sake of the example) would read
gs == {
ParameterType -> Internal,
ComplexParameter -> False,
InteractionOrder -> {QCD, 1},
Value -> Sqrt[4 Pi aS],
ParameterName -> G
}
Once again, please ignore the Mathematica syntax and mentally trade it with a Python class. I need to discuss one of the above lines in detail (the rest can be guessed). Some parameters are special in the sense that one must clearly be able to identify what they are physically (physics always strikes back). This is what the ParameterName option allows us to do in the above syntax. It tags gs
as a ‘G’ parameter that is well known and with well defined properties.
MATRIX PARAMETERS AND INDICES
Some specificities exist for parameters that are matrices. First of all, their indices must be specified. In addition, some properties can optionally be turned on (unitarity, hermiticity, orthogonality).
For instance, in the FeynRules syntax, the typical declaration of a matrix would read
CKM == {
ParameterType -> Internal,
Indices -> {Index[Generation], Index[Generation]},
Unitary ->True,
ComplexParameter -> True,
Value -> { … }
}
Any unindicated option (hermiticity, orthogonality) means that it is set to false (that is the default choice). Moreover, the value of the parameter, represented by the dots, is this time a list of values, one entry for each element of the matrix.
Moreover, for external matrices, one needs as usual to provide a block name. There is however no need to specify the counter. They can be dealt with internally. The counter consists this time in a 2-tuple corresponding to the line and column numbers of each element.
Very importantly, one must be able to generalize that to any tensor (any parameter carrying at least 2 indices).
Concerning the indices, they must also be declared. In this declaration, one must define their range and their unfolding properties. The latter is a bit hard to explain. In short, if set to true, it means that if the index appears summed in the Lagrangian, the summ must be explicitly expanded when the Lagrangian will be treated.
SUMMARY AND EXTRA INFORMATION
In this post, we really start this particle physics research project at SteemSTEM/Utopian.io.
I decided to focus on the input parameters that the user will have to provide and that the code will have to read, and process internally.
The tasks that have to be done for now are quite simple.
- The first step is to design a command line interface (CLI). Whilst users can in principle have their own Python program handling tasks, some may want to have a CLI to act on the various objects. We will, task after task, introduce the set of methods that this CLI should be able to cope with.
- One needs to define a class to handle the parameters of a particle physics model (i.e. the parameter class), that are provided as inputs by the user.
- One needs to design a bunch of functions allowing to test whether a parameter is real, is complex, is a matrix. In case of a matrix parameter, one also needs functions testing the unitary/hermitian/orthogonal nature of the matrices. Those functions should be methods of the class, as well as methods taking a parameter as an argument that could be played with in the CLI.
- One especially needs a function that returns the coupling order of a product of parameters (could be something like QCD2 QED3 if we have several coupling orders involved.
- BONUS: we may need a nice logo for this project! ^^
I am totally open for the strategy to follow for the input format. The simplest option is generally the best and is the one I will choose as a default. However, if you want to get an opinion before coding, leaving a comment to this post may be helpful.
I think that for now, we are all good. To tackle this project, I have created a fresh GitHub folder. The subdirectory structure is left open for now.
Please do not hesitate to come back to me for questions.
Thanks for the task request, @lemouth! I'm very looking forward to getting started with this (already doing some research) and curious to see if there will be others who are also willing to help out.
Everything about the task is pretty much clear to me, so I think you did a great job of explaining everything. I think it will be quite a lot of work, but people who are interested and invested in the project will definitely be able to take it on!
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thanks amos for the report. I am pretty exciting to see where this will bring us. Let's create a precedent! :)
Thank you for your review, @amosbastian!
So far this week you've reviewed 20 contributions. Keep up the good work!
OK, this seems interesting. Not sure how much time I'll have but I can give it my best shot.
As always it seems like most of the complexity is going to be about figuring out the concepts to be implemented.
Are all participants to develop their own implementation of the same thing or is it a collaborative project?
I was more thinking about a collaborative thing. Let me see how we can organize ourself better and I come back to you asap.
In any case, thanks for your interest!
Cool!
I forgot to ask. Does particle physicists that work using Python also use JupyterNotebook/JupyterLab?
I'm wondering if Javascript communities can also take parts someday by providing custom widgets/extensions for visualization or mini-dashboard.
Not to my knowledge.
We need more tools for doing actual calculations than visualizing things. From there, I am not too sure about what could javascript brings. This being said, this cold be useful for outreach.
Well, it looks straightforward. Who am I kidding? I have no idea the difficulty or ease of the project. But I'm pretty excited to see the end product.
Let's see whether it will work. I am pretty excited about it! :)
There's only one way to find out which is what is already in progress; that way is to try.
I will keep you updated! :)
Thank you :)
Hi @lemouth!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Congratulations @lemouth! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
Click on the badge to view your Board of Honor.
If you no longer want to receive notifications, reply to this comment with the word
STOP
Hey, @lemouth!
Thanks for contributing on Utopian.
We’re already looking forward to your next task request!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Thanks! But we will first finalize this TR before moving on with the next one ;)
Excellent @lemouth. Regards.
Thanks for passing by ^^
New to Steem, but interested in this project. I think this project needs a design of the datastructure. So some sort of Object Oriented (OO) design. Also an example function on instances of those classes will probabely clear things up. I now a bit about design patterns f.i. to generate instances of the classes. I think a preliminary (dummy) implementation can speed up the discussion. Also some unittests will help clear up the input and output for which the implementation will follow later. Can I help in this?
We have a discord group. Please add me as a friend on discord (lemouth#8260 ) and I will add you in.
@remind-me in next week
Hey @drsensor, I will notify you on November 12th 2018, 12:00:00 pm (UTC)
Later! ( read more... )
Hi @drsensor!
You asked me in this comment to create a reminder.
It seems the time has passed!