Check your followers with steem-python
Hi steemit friends.
I recently found the steem-python library and wanted to experiment a little bit with it. I wrote a simple script that lists all my followers and writes a simple .html file as a result.
The result
When executing the script, a .html file is generated with a timestamp in the current folder. So you could execute the script multiple times (e.g. each week) and compare the result files. The result file lists all the followers in a table with the following attributes: Account Name, Steem Power, Voting Power. The account names are directly linked, so you can click on the name and in a new tab the account opens on the steemit website.
The code
Basically it all start with the creation of a steem object:
s = Steem()
I introduced a dictionary to not load an account multiple times:
dict = {} # dictionary to tmp store accounts
def get_account_from_map(account):
a = dict.get(account, '')
if a == '':
a = s.get_account(account)
dict[account] = a
return a
I defined some helper methods to get the sp and vp in printable format (note: i added rounding)
def get_sp(account):
a = get_account_from_map(account)
return round(Converter().vests_to_sp(int(a['vesting_shares'].split('.')[0])))
def get_vp(account):
a = get_account_from_map(account)
return '{0:.2f}%'.format(int(a['voting_power'])/100)
And finally loop over the followers and write a .html file (note: i simplified the code a bit for better readability):
followers = s.get_followers('jjb777', '', 'blog', 1000)
file = open('file_name', 'w')
file.write('')
for follower in followers:
name = follower['follower']
file.write('<tr><td><a href="https://steemit.com/@{}">{}</a></td><td>{}</td><td>{}</td></tr>'.format(name, name, get_sp(name), get_vp(name)))
file.write('</table></body></html>')
file.close()
The full script is on my github account: followers_html.py
The preconditions
- To run this code you need python3 and steem-python installed
- Adapt the constants (ACCOUNT_NAME, FOLLOWER_LIMIT) before you run the script yourself
The Conclusion
what have I learned from all this?
- I made my first steps with python and steem-python
- Analysing the output of my script:
- I have only one follower with more than 10K SP, thanks @libertyteeth
- From my 223 followers there are 13 above 1K SP.
What's next?
I'm eager to try out more functionality of the steem-python library. Maybe I will write more posts about it.
If you have suggestions please leave a comment.
In the end it was all fun to me. If you like this post please upvote or leave a comment.
Thanks. J
Thanks for this. I am very impressed with all the helping hands here on steemit.
is it just me? but your script is veryyy slooow, it takes forever to download the stuff and save as html
Hey. Thanks for trying my script.
The time is used to fetch the follower accounts. Maybe there is faster implementations but it worked fine for me.
There is one limit I found while experimented with it. There is a hard limit of 1000 in the method of tge steem python library to get the follower accounts. If you have more you'll have to load all followers in batches of 1000 to get them all.
J
thats the reason why it stopped after 1000 :D good to know, I made another script. I used beautifulsoup and scraped the follower/following list from steemdb.com and saved as a CSV file. But I need help to sort the csv list, remove duplicates etc. properly with python and the names are in a chronological order on the website,that makes problems, ah its complicated :D I consider to post my script in the next days
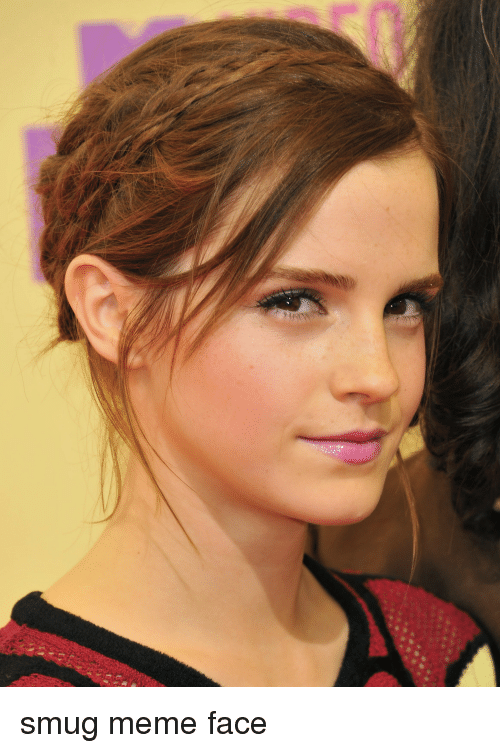
There's lots of ways to remove duplicates. U can write a script ;-)
Or use an editor that can do this. Or maybe excel can do this too.
J
actually I can then make this correction fast in libreOffice but I want for the end user to have everything already done by running once the script :D the problem is, if i save the follower list as an array, it could maybe break if the list is huge. any ideas? have an account on steem.chat?
Lol. What are you trying to do? If you put everything into a set/dict the duplicates may go away. There should not be memory problems unless you try to save millions of data sets. If you reach the limits you should have a look at streaming files and free temporary data sets. Using streaming for files uses buffers to limit the amount of data that is held into memory. You may want to free memory by explicitely freeing unused memory.
But I don't know how to do this in python. I'm sure there's lot's of info in the web. Python is well-used.
Jah, I made an account in steem.chat months ago but I don't use it.
J
Hi Mate, found your post a bit late.
Please have a look below at a piece of code that will solve the issue with the 1000 limit.
Thanks bro. Thats a good idea to process them all. J
Cool post dude. I have followed.
Follow me @codero
Thanks. Followed you. I'll check yar posts later on. I'm on discord if you want to discuss js or coding stuff. J
Holy smokes if this is the first attempt, can't wait to see what your future attempts will hold.
God bless.
Namaste
Yes what @chiefmappster said. This feels like something I'm going to want to look back at so I had to make some mark so that I can come back and reference. My future understanding very much appreciates this post,.Thank You for sharing!
You are speaking my language :)
God bless.
Namaste
Thanks. Maybe I'll do more posts with steem python experiments. Check my blog from time to time. J
This is great, now I've seen your post I'm inspired to try myself :)
Thank you. I just started python to learn data analysis, so I can use this as a good exercise, thanks.
This post has received a 13.51 % upvote from @buildawhale thanks to: @jjb777. Send 0.100 or more SBD to @buildawhale with a post link in the memo field to bid on the next vote.
To support our curation initiative, please vote on my owner, @themarkymark, as a Steem Witness
Well done. That deserves an upvote. :D
Cool Im self taught on Python so not too good at coding, but this could be fun to play around with
Thanks. Yap is fun to play around. The documentation is actually difficult. J