Learn Basic Python Programming EP.4. - Let's build a Calculator
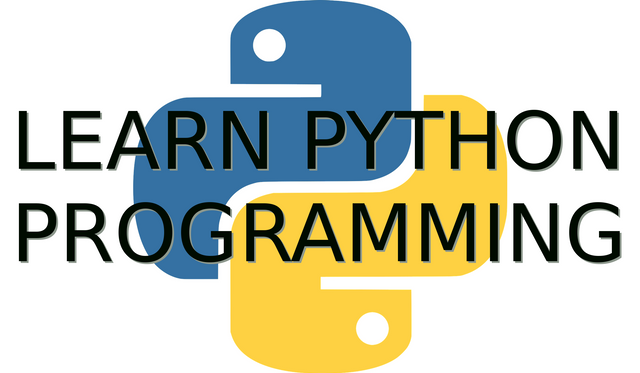
After you've read my initial 3 tutorials, which you can easily read and absorb in 1 hour or less, the most basic thing you can build now is a basic calculator script. You know Linux or Windows is already equipped with a nice GUI calculator, Gnome Calculator in Linux, but we don't need a fancy GUI, just a good old terminal and a keyboard and we can do any math operation we want.
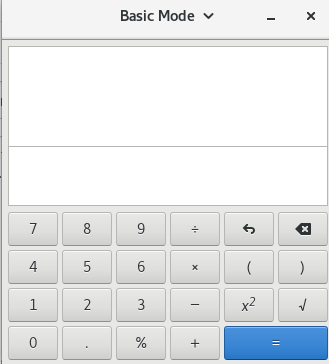
Make sure you read all 3 parts of th tutorial, you need all the knowledge from there:
- https://steemit.com/programming/@profitgenerator/learn-basic-python-programming-ep-1
- https://steemit.com/programming/@profitgenerator/learn-basic-python-programming-ep-2-variables-and-inputs
- https://steemit.com/programming/@profitgenerator/learn-basic-python-programming-ep-3-logic-and-loops
We will use python3
, and we will name the file calc.py
. We will also need to import the math
package in order to access more complex functions like logarithms and such.
Then we will let the user choose the operation, and select it first. Then we test which one of the operation is selected, and and the user will enter 2 numbers which will be used in the operation.
Well it looks like this:
Not all of it fits in 1 screenshot so here is the full script:
Basically we input an operator defined as above: + - / * log ^
, where ^ is the exponential operator and log is the logarithm.
Then we use the if/elif/else
structure to test which operator did the user select, then the user should input 2 numbers, and then we perform the operation on them. In case of the log, first we input the argument, then the base, in case of the exponential we input the exponentiation first we input the base then the exponent.
The exponent operator is **
if you didn't know. And we use the math.log
from the math library that is imported to perform the log operation.
Now let's add an else operator if the user adds an operator that is not recognized:
else:
print("Sorry this operator is not recognized")
But also we can add more operations, like algebraic or trigonometric stuff:
Watch out some functions have only 1 argument like Sine and Cosines, so we only need 1 variable there. Also add the else to the end in case the user inputs and unknown command, or mistypes it.
But you can also include tons of more functions there, the math
library has tons of functions, you can read about them here:
- https://docs.python.org/3.5/library/math.html (for python 3.5 which I use)
- https://docs.python.org/3.6/library/math.html (for python 3.6 the latest stable release)
So if you need hyperbolic sine function for some trigonometry project of yours, just use math.sinh(x)
or if you need the error function for some statistical project then use math.erf(x)
, and things like that.
Here is the full code I wrote here, but you can just add essentially any mathematical operation you want:
See you don't even need a fancy GUI calculator, you can just calculate stuff this way, quickly and efficiently!
This is how easily you can make your own calculator, and this is nothing, with Python you can do a lot lot more, more complex and more interesting projects:
- Electrum Bitcoin wallet was written in Python.
- The Joinmarket was written in Python, and helps enhancing the Privacy of Bitcoin users, it's basically a python script like this
So I am telling you, the sky is the limit with Python, you can create tons of good softwares that can help you in cryptocurrencies or in your day to day life.
Sources:
https://pixabay.com
Python is a trademark of the Python Software Foundation
awesome, thanks for this !
Very cool, I'll have to go back and look at your previous EP.
Buddy... what about GUI ,... how can we implement that?
You need a separate package for that I believe it's called TK:
Python by itself is terminal based.
I've never done GUI in Python, so I have no clue how to do it.
Ok buddy 👍
Tkinter is default but pyQt would be recommended. We can do some advanced stuff in future.
Also, a small suggestion. Could you please paste the code directly here instead of screenshots. Is there any issue?
Lets see how syntax works here:
Looks like syntax highlighting isnt available on steemit 😐
How to put multiple lines in a code on steemit, do you use the "``" marks or what is the markdown syntax for code?
" ``` "
Code goes here
" ``` "