Learn Basic Python Programming EP.3. - Logic & Loops
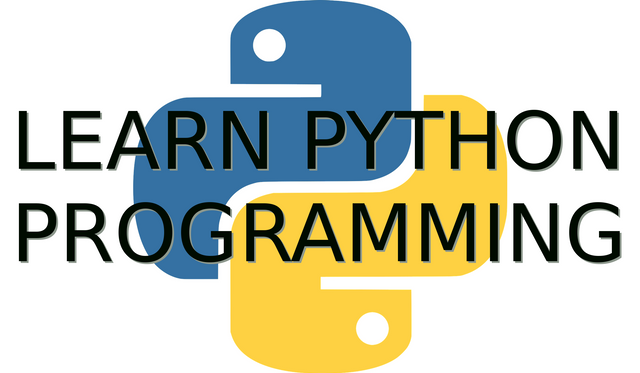
Hello in this episode we are going to learn about logical operations, and loops. But @ragequit reminded me that we should actually use Python3 since it replaced Python2.x, it has support while Py2 was abandoned I believe and it has much more features, bug fixes and such. So it's actually better to learn Py3 since its the current best version of the language.
Now there are a few changes in Py3 compared to Py2, first of all you don't call the script anymore with python test.py
, but with python3 test.py
, so the python3
function is what calls the Python3 language.
Then we have to rewrite some of the logic, since Python3 requires all functions's arguments to be inside ( )
brackets, so this means we can't write print "blablabla"
anymore, but we have to write print ("blablabla")
. And many functions wer also renamed like raw_input()
with input()
and such...
So this s how our calculator script looks like on Python3:
Actually you can put the description inside the input()
function if you want, so it looks like this:
These screenshots looks a little bit odd here on Steemit so let me add a line to it to separate the screenshot from the text here on Steemit, it would look easier to visualize.
Logical Operations
Now I am going to teach you about using logical operations, they are if
and else
for simplicity. If x operator y then do z task
, that is how it basically looks like. Or if there is an exception to the logical rule, then use else
to account for the exceptions.
So I am creating a file logic.py
, to not mix it up with the other file and let's see how these logical operations work:
First of all I have customized this a little bit so the commented --------
lines separate he screenshot better from the text on Steemit and the printed out --------
lines the screenshot of the terminal from the text on Steemit, so don't be scared about all those lines, it's just cosmetics.
Then what we did here is basically choose an evil number 666
, then enter 2 numbers from the keyboard, add them together, and if the sum is 666 then write out that it is, or if not, then write it that it isn't.
One thing that you need to keep in mind is that you must put a :
after the if/else
function to signal that the command after that is inside that logic. You also must indent every command inside an if/else
by at least 1 space to show that that is inside it. In C++ you basically use { }
brackets to show where a logic ends, but here we use indents, basically 1 space or a tab.
The logical operators are the following:
==
is equivalence=!
not equal>
larger<
smaller>=
larger or equal<=
smaller or equaland
logical andor
logical or
The math operators only work for numerical variables while the equal not equal can work for strings too.
If there are multiple logical points you want to touch you can use elif
short for else if, which you can specify other logical options, like 1 number from the chosen number in our example. And then finish off the logic with else
which contains every other option except those specified in the if
or elif
. Also this is part of the same logical structure so the if elif else
commands must have the same indentation levels, while their arguments should be indented 1 space to the right.
We also used the or
operator in the elif
structure which tests 2 logical possibilities, either this or that is true, but if 1 option is true, then it gives out that command, otherwise it just goes to the else
option. So in this case we test for 1 number away from the favorite number +/- 1
basically, and if that is true than it gives out this :
Loops
There are 2 kinds of loops, while
and for for
the basics, a while
loop runs until the argument is true, a for
loop runs until it's specified. In this case we will create another file loop.py
to test out these.
WARNING IF LOOPS ARE USED INCORRECTLY, THEY CAN RUN INFINITE TIMES, AND FREEZE OR CRASH YOUR COMPUTER. BE CAREFUL HOW YOU USE THEM, I AM NOT RESPONSIBLE IF YOUR COMPUTER CRASHES, OR ANY OTHER HARM HAPPENS TO YOU OR YOUR COMPUTER.
While Loop
So to use a while loop it has the same structure as other logical operators, you must use :
after it and indent everything inside it's argument. A while loop runs until the argument is true, or in other words > 0, and it stops when the argument reaches 0.
In this case the number is 5, we print out "Hello"
, then we subtract 1 from it, after the number hits 1, in prints out "Hello"
1 more time, then it subtracts 1 from it, and it becomes 0, then the loop stops.
The number-=1
means number=number-1
similarly number+=1
is number=number+1
, but don't use +
here because that would make the loop run forever since it never becomes 0 and it will probably crash your computer. It returns:
It printed out "Hello" 5 times just as predicted.
While loops are only for careful people because using them incorrectly can crash a computer, but also a for loop as well if the argument runs for infinity, but there the chances are smaller since you specify the range.
For Loop
The for
equivalent to the while
loop above is this:
Here we specify the range directly. But we also need another variable that will iterate itself, in this case x
that can also be used as an enumerator. Like this:
Which looks like this:
In Python all enumerations start from 0, and in this case goes 5 times, so the 5th number is the 4th indexed element.
Note: You use while
when you don't know the size of the range, and just want to test something down recursively, and you use for
when you want to enumerate something and you already know the size of the range.
That's it for this episode, I hope you have learned a lot of new things!
Sources:
- https://pixabay.com
- Python is a trademark of the Python Software Foundation
This is awesome! I'm very happy to see someone doing this here. Just two days ago I paid for some courses on Udemy.com to learn python and deep learning/data science with python. I will be keeping an eye on your future posts and thank you for sharing and educating.
I am not an expert on python in anyway, but I would consider myself like medium knowledgeable about it, I am happy to share basic knowledge here with anyone who would like to learn Python.
Sweet!
The way I like to think about the for loop in python is that it's actually the foreach from other languages. Since you can achieve with the while loop anything you could with the classical for loop why not shorten the foreach and call it a day!
I didnt quite understood what is the difference between foreach and for, I believe it can iterate other things too not just numbers.
Also keep in mind that I have like medium knowledge about python, I have not much experience with using classes and other objects , I have to learn more about those. But I will touch functions and other basic things in this tutorial.
Explaining to others is a great way of making sure you understand something, it's a good thing to do once you feel like you master something. It also helps you figure out what you don't understand that well and look over it again.
The idea is that Python doesn't have a foreach syntax like other languages, instead the for loop works like a foreach would. If you have no idea what I'm talking about don't stress it, it's just a tip for whoever is coming from another language that has the classic for loop
to remember that python's for is actually a foreach
I never thought I would see a Python tutorial from you. I have lots to say about python3. It's too much for a comment though.
I have been using it for a long time to calculate stuff, especially for trading. I thought I might share my knowledge here. After all this platfom is for sharing ideas and knowledge and I am happy by sharing my knowledge here.
Here is why I use Python 3.6
https://steemit.com/programming/@leprechaun/why-you-should-use-python-3-6
Python 3 ... Cool :-) just what we wanted.... you are really listening to the viewers :-) the content also is spot on... resteeming all of your python tutorials :-)