Learn Basic Python Programming EP.2. - Variables & Inputs
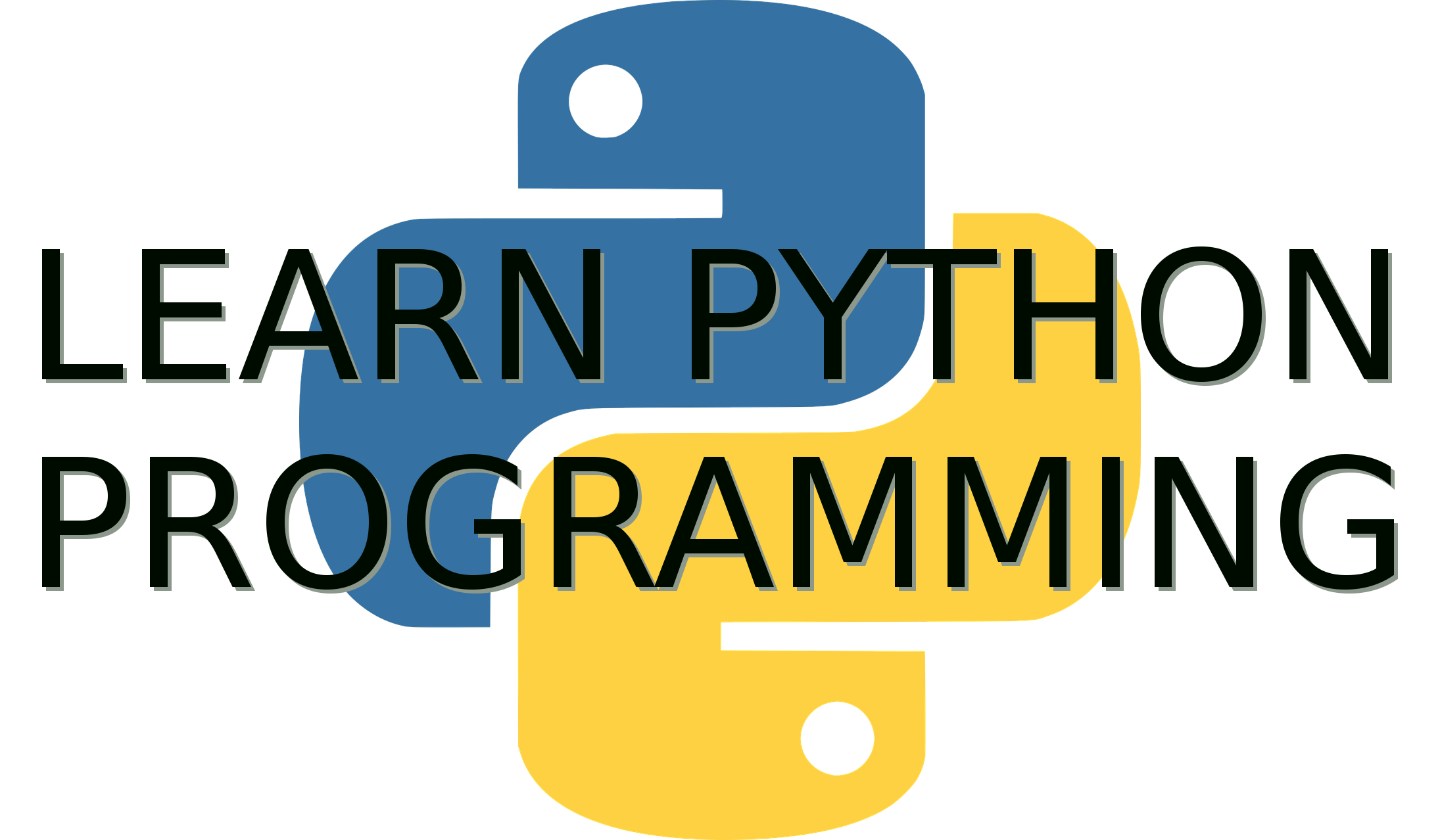
In the past episode we learned the absolute basics how to execute the script and how to print out (display) text in the terminal:
In this episode we are going to learn how to use variables and how to interact wit the script, by inputting things from the keyboard.
Variables
In python you don't need to declare variables (str,int,float,double) like in C++, the code itself automatically detects what kind of variable it is, so it's very easy to use for novices. Essentially you can transform or specify later on, but not always needed.
Let's go back to our test.py
file, and see how to use variables. Well basically you just write the variable name, an =
and the value of the variable. For numbers you just write the number , for a string variable, like a text, you just write it in the string wraps "
or '
we learned in the past episode.
Which will display:
So we have just substituted the argument of the print
with a variable, instead of writing print "text"
we just use the variable text_variable
to contain the data "text"
and we print it out this way.
Also note that a number can be a string
too however if we put the number in the ""
wraps it becomes a string variable, and only string operations can be done with it like concatenation, trimming ,separation into characters and such. While an integer can only be used mathematically, like addition, division ,etc...
It may display the same value, but it's a different kind of variable used for different purposes:
Displaying:
Math Operations
Well you just use the basic operators + * - /
just like in any other math software. Then give value to your variables that you want to calculate from, and perform the operation. It would work just fine, obviously you can't divide by 0 and things like that, but otherwise it should work fine.
Displaying:
It works just fine, except for division, it's because all math variables are by default integers I believe, and a division, unless it's a round number, will always give out fractions, which is a float variable with decimal points after it. In python we use the float()
function to turn the number into a float variable, but then we have to transform each variable into float, or we make the initial variable float by adding a .0
decimal to the number.
So either like this:
Or like this:
Both displaying the correct values this time:
Inputs
Now let's interact with the script, let's input the values of A and B directly from the keyboard from the terminal. For this we are going to use the raw_input()
function. Every time this is called, the terminal will be waiting for a text input. So you write something in the terminal, and you finalize it by hitting Enter
on the keyboard. So in this case the raw_input()
will be called 2 times separately for A and B, which means that you will have to enter a number, then hit enter, and then enter another number. The order is as specified in the script, in our case the first one is A. We also have to transform it into float()
since the raw_input()
is a string based function.
Of course B can't be 0, we can't divide by 0, and you can't enter a text, otherwise it will be an error. So just enter 2 numbers:
So I have entered 56 for A, hit enter and 14 for B and hit enter. Then it displayed the results of those calculations.
And hence this is how you created your very first calculator software, it's just this easy!
Essentially, let's make it more user friendly and add instructions inside it:
Note that we can't combine float variable with string, so we have to turn the float into string with the str()
function. And adding together 2 strings is called concatenation which is done with +
operator. It means that the 2nd string will be put after the end of the first one.
So that is how you have your first calculator ready to calculate for you.
Stay tuned for the next episode, I will be teaching you even more interesting stuff about programming!
Sources:
- https://pixabay.com
- Python is a trademark of the Python Software Foundation
You seem to be teaching Python 2.x. That's probably not a great idea since the backwards compatibility is broken since Python 3 and everyone is either in process of migrating to Python 3 or done doing so.
For anyone interested in great python documentation I suggest starting with David Beazley. His presentations are incredibly funny and informative, and his books are pure gold.
I can appreciate the amount of effort that went in this guide and I'm not trying to put you down or anything, keep up the work and best of luck!
Yes it's true, but it's easier to learn. I am also using mostly Python 3 these days, but for simplicity python 2 is also very useful.
Nice tut!
raw_input()
is replaced withinput()
in py3, right?That's right, I will rewrite the script to be compatible with py3 in the next episode, and teach people about logics and loops.
🆒
Actually I will transition to python 3, no need to teach people depreciated stuff!
I personally think it's for the best :) Can't wait to check it out, i'm subbed!
Oh and if I could make one last suggestion it would be to have the code selectable.
Good luck!
Beauty of python... No need to declare variables; just use it on the go ☺️👍
A double edged sword kind of beauty. I come from C++ world so I'm a little biased. lol. C++ was my first. Definitely easier for beginners.
Hum I have studied both... I think if you were exposed to python first, you will feel python is easy.. Likewise with cpp ☺️
Just to be clear I meant Python is easier for beginners (easier is a bit subjective though), but it is all the same really in the end once you get past the hurdle of the basics. Just maybe a different set of gotchas for each language. C will yell at your typos a little better versus having to debug at run time. It can get confusing switching around a lot. lol
Something like VB.Net might be even easier especially if you want to get a pretty GUI going as a beginner.
Yes indeed ☺️ I want to learn VB also