Easy Tutorial: Computer Programming for DUMMIES -- *100 followers edition!!* -- file i/o
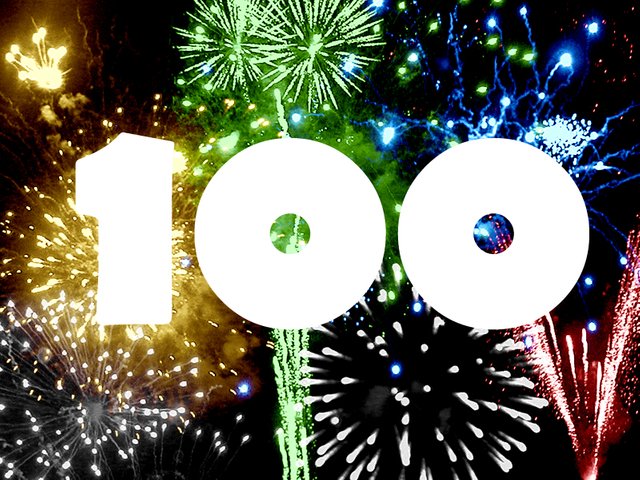
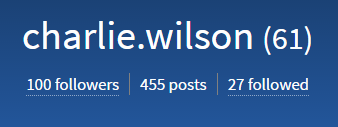
I made it! It took a lot of posts to get my first 100 followers, but it was worth it. In fact, it wasn't really work at all. I love steeming! This is a great website! I want to show my appreciation for every single one of my followers! I may not be THAT popular on Steemit, but I am doing pretty well. I have all 100 of you guys to thank for that. I know I'm being a little corny and all with the fireworks, but I feel like it is appropriate to celebrate my little bit of success so far (and hopefully more to come). I thought that a great way to do this is by incorporating my celebration into one of my more popular post series.
This is my 25th programming tutorial (of this series) in C++. This will be about file input and output. At the end of this tutorial, I will write a program that takes a file with the names of all of my followers, processes the content, then individually thanks every one of them!
If you want to check out my other tutorials in this series, here they are:
Part 11: Colored Text in your Terminal
Part 16: Binary, Bitwise Operators, and 2^n
Part 19: Object Oriented Programming 1 -- Data Structures
Part 20: Object Oriented Programming 2 -- Classes
Part 21: Object Oriented Programming 3 -- Polymorphism
Part 22: Command Line Arguments
Part 24: Programming in separate files
For this tutorial, I will be showing the method for the C language. This will also work in C++, but there is also another way that is slightly easier. I believe that it is important to know how to do things in C, even if it isn't the easiest language to work with. Leave a comment if you would like me to post the C++ method with the fstream library.
Opening a File
To open a file in C, we need to create a file pointer. To do this, simply type:
FILE *fp;
- this creates a file pointer called fp
After this, it needs to be associated with whatever file that is being opened. We can do that with a simple function, fopen().
The arguments for this function are:
- the file name
- an access type
Here is a list of access types and each of their purposes:
Image source
Examples:
fp = fopen("file.txt", "r");
- opens a file called "file.txt" for reading only
fp = fopen("file.txt", "w");
- opens a file called "file.txt" for writing only
fp = fopen("file.txt", "r+");
- opens a file called "file.txt" for reading and writing
Don't worry too much about all of the access types right now. Study the rest of them after you are familiar with the basics.
Reading from a File
Reading from a file is fairly simple. You can use file i/o functions such as fscanf() and fgets().
fscanf()
This is the file i/o version of scanf().
Syntax:
fscanf(file_pointer, format, variables);
- this works just like scanf(), except that it reads from a file
- the first parameter is a pointer to the file
- file_pointer --> a pointer to a file
- format --> the format fscanf() will be storing the data as (Ex: %s %d %f)
- variables --> the variables that the scanned data will be stored in
Here is a more specific example:
fscanf(fp, "%s %s %d", string1, string 2, integer);
- this scans the file that fp is pointing to
- fp --> a pointer to a file
- string1 --> a string that the first segment of scanned data is stored
- string2 --> a string that the second segment of scanned data is stored
- integer --> an integer that the third segment of scanned data will be stored in
fgets()
This function reads a line from a file and stores the contents into a string. It stops when either a certain number of characters are read, a newline is read, or if it reaches the end of the file.
Syntax:
fgets(string, n, fp);
- string --> a string that the scanned data is stored in
- n --> an integer that describes how many characters that are being scanned
- fp --> a pointer to a file
More specifically:
char string[50];
fgets(string, 50, fp);
- string --> a character array to store the contents that are scanned
- "n" in the last example was replaced with 50. If we put a higher number in fgets(), then we may get an error. string can only hold 50 characters.
- fp --> a pointer to a file
Writing to a File
Writing to a file is also fairly simple. You can use functions such as fprintf() to do this.
fprintf()
fprintf() is the file i/o version of printf().
Syntax:
fprintf(file_pointer, format, variables);
- this works just like printf(), except that it prints to a file
- file_pointer --> a pointer to a file
- format --> the format fprintf() will be writing the data to (Ex: %s %d %f)
- variables --> the variables that are being printed
Here is a more specific example:
char* string1 = "The";
char* string2 = "number";
int integer = 7;
fprintf(fp, "%s %s %d", string1, string 2, integer);
- this prints to "The number 7" to the file that fp is pointing to
- fp --> a pointer to a file
- string1 --> a string that holds "The"
- string2 --> a string that holds "Number"
- integer --> an integer that holds 7
Closing a File
Closing a file is easy. The function fclose() will close a file. All that it needs is the file pointer.
Example:
fclose(fp);
- fp --> a pointer to a file
- this closes the file that fp is pointing to
More File i/o Functions
There are many more file i/o functions for C. Here are some examples:
Programming Examples:
Here is a simple program I wrote to implement reading and writing to a file called text.txt:
#include<stdio.h>
int main()
{
FILE* fp = fopen("text.txt", "r+");
char buffer[50];
while(fgets(buffer, 50, fp) != NULL)
printf("%s", buffer);
printf("\n");
fprintf(fp, "\nMore text for the file");
fclose(fp);
return 0;
}
- create a file pointer called fp and open "text.txt" for reading and writing
- buffer --> character array to hold each line that is read
- the while loop will read one line at a time up to 50 characters
- fgets() returns NULL when it is at the end of the file
- print each line as it is read
- write "\nMore text for the file" to the file
- close the file and end the program
text.txt before running the program:
This file contains random text.
It only has two lines.
text.txt after running the program:
This file contains random text.
It only has two lines.
More text for the file
Output:
[cmw4026@omega test]$ gcc fileio.c
[cmw4026@omega test]$ ./a.out
This file contains random text.
It only has two lines.
[cmw4026@omega test]$
Finally, here is my program thanking all 100 of my followers!
Here is the format of the text file I named "followers.txt" copied and pasted right off of my Steemit page:
FOLLOWMUTE amat
FOLLOWMUTE nonameslefttouse
FOLLOWMUTE seafarer124
FOLLOWMUTE riffatniaz
FOLLOWMUTE alabamanarchy
UNFOLLOWMUTE highimpactflix
- It is a weird format, but I can work with it!
Here is my program:
#include<stdio.h>
#include <string.h>
int main()
{
FILE* fp = fopen("followers.txt", "r");
char name[50];
char temp[50];
fscanf(fp, "%s %s", name, name);
while(strcmp(name, temp) != 0)
{
strcpy(temp, name);
printf("Thank you for following me %s! I appreciate your support!\n", name);
fscanf(fp, "%s %s", name, name);
}
printf("\n");
fclose(fp);
return 0;
}
- open the file
- name --> a string to hold the name of each follower
- temp --> a temporary variable to compare values
- fscanf(fp, "%s %s", name, name);
- this reads "UNFOLLOW" OR "FOLLOW" first and stores it into name
- then it reads the follower's name and overwrites name with that
- the while loop
- executes while the last name read isn't the same as the current name read
- fscanf() does this weird thing where it will read the last line twice
- the while loop executes until a name is repeated, thus the end of the file
- print a thank you note for each follower
- executes while the last name read isn't the same as the current name read
- close the file and exit
Here is the output:
[cmw4026@omega test]$ gcc 100.c
[cmw4026@omega test]$ ./a.out
Thank you for following me amat! I appreciate your support!
Thank you for following me nonameslefttouse! I appreciate your support!
Thank you for following me seafarer124! I appreciate your support!
Thank you for following me riffatniaz! I appreciate your support!
Thank you for following me alabamanarchy! I appreciate your support!
Thank you for following me highimpactflix! I appreciate your support!
Thank you for following me jacobcwitmer! I appreciate your support!
Thank you for following me escapethefate! I appreciate your support!
Thank you for following me zodiac! I appreciate your support!
Thank you for following me sergey44! I appreciate your support!
Thank you for following me lajulius! I appreciate your support!
Thank you for following me heroic15397! I appreciate your support!
Thank you for following me n25052016! I appreciate your support!
Thank you for following me plentyoffish! I appreciate your support!
Thank you for following me goose! I appreciate your support!
Thank you for following me apolymask! I appreciate your support!
Thank you for following me riosparada! I appreciate your support!
Thank you for following me alextheyounguh! I appreciate your support!
Thank you for following me therealpaul! I appreciate your support!
Thank you for following me rxhector! I appreciate your support!
Thank you for following me tux! I appreciate your support!
Thank you for following me kirk-gosik! I appreciate your support!
Thank you for following me screenname! I appreciate your support!
Thank you for following me electrowarrior! I appreciate your support!
Thank you for following me martin-stuessy! I appreciate your support!
Thank you for following me wildmanhowling! I appreciate your support!
Thank you for following me steemswede! I appreciate your support!
Thank you for following me pictures! I appreciate your support!
Thank you for following me ptacatie! I appreciate your support!
Thank you for following me therajmahal! I appreciate your support!
Thank you for following me bitcoinparadise! I appreciate your support!
Thank you for following me wilmaballsdrop! I appreciate your support!
Thank you for following me burnin! I appreciate your support!
Thank you for following me dragonanarchist! I appreciate your support!
Thank you for following me grandpere! I appreciate your support!
Thank you for following me eagleesbd! I appreciate your support!
Thank you for following me liberosist! I appreciate your support!
Thank you for following me renz-27! I appreciate your support!
Thank you for following me katiasan1978! I appreciate your support!
Thank you for following me celsius100! I appreciate your support!
Thank you for following me nin0000! I appreciate your support!
Thank you for following me gikitiki! I appreciate your support!
Thank you for following me ottodv! I appreciate your support!
Thank you for following me tink! I appreciate your support!
Thank you for following me kevinwong! I appreciate your support!
Thank you for following me virtualgrowth! I appreciate your support!
Thank you for following me vegascomic! I appreciate your support!
Thank you for following me stealthtrader! I appreciate your support!
Thank you for following me freejayforever! I appreciate your support!
Thank you for following me cryptoctopus! I appreciate your support!
Thank you for following me asmolokalo! I appreciate your support!
Thank you for following me iggy! I appreciate your support!
Thank you for following me steempowerwhale! I appreciate your support!
Thank you for following me rafaela! I appreciate your support!
Thank you for following me edtorrez! I appreciate your support!
Thank you for following me maxigan! I appreciate your support!
Thank you for following me techslut! I appreciate your support!
Thank you for following me seljalex77! I appreciate your support!
Thank you for following me alphabeta! I appreciate your support!
Thank you for following me steemitpolonia! I appreciate your support!
Thank you for following me kcwagenseller! I appreciate your support!
Thank you for following me ned! I appreciate your support!
Thank you for following me getonthetrain! I appreciate your support!
Thank you for following me appliantologist! I appreciate your support!
Thank you for following me luzcypher! I appreciate your support!
Thank you for following me rissakoirtyohann! I appreciate your support!
Thank you for following me ibringawareness! I appreciate your support!
Thank you for following me nathwright876! I appreciate your support!
Thank you for following me echoesinthemind! I appreciate your support!
Thank you for following me f1111111! I appreciate your support!
Thank you for following me normdeguerre! I appreciate your support!
Thank you for following me sammie! I appreciate your support!
Thank you for following me alexpatin! I appreciate your support!
Thank you for following me ausbitbank! I appreciate your support!
Thank you for following me alexgr! I appreciate your support!
Thank you for following me pilgrimtraveler! I appreciate your support!
Thank you for following me ajaub1962! I appreciate your support!
Thank you for following me radioactivities! I appreciate your support!
Thank you for following me haster! I appreciate your support!
Thank you for following me thedevil! I appreciate your support!
Thank you for following me listentojon! I appreciate your support!
Thank you for following me ats-david! I appreciate your support!
Thank you for following me otisbrown! I appreciate your support!
Thank you for following me therdm000! I appreciate your support!
Thank you for following me mandibil! I appreciate your support!
Thank you for following me tommycordero! I appreciate your support!
Thank you for following me thecryptofiend! I appreciate your support!
Thank you for following me justkd! I appreciate your support!
Thank you for following me timbo! I appreciate your support!
Thank you for following me reddust! I appreciate your support!
Thank you for following me bontonstory! I appreciate your support!
Thank you for following me sbuh! I appreciate your support!
Thank you for following me thoughts-in-time! I appreciate your support!
Thank you for following me dwinblood! I appreciate your support!
Thank you for following me xyanthon! I appreciate your support!
Thank you for following me always1success! I appreciate your support!
Thank you for following me stagg! I appreciate your support!
Thank you for following me dangis! I appreciate your support!
Thank you for following me naquoya! I appreciate your support!
Thank you for following me derekareith! I appreciate your support!
[cmw4026@omega test]$
I really mean it! Thanks guys!
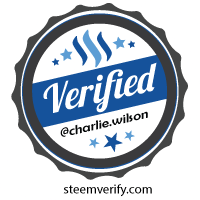
Congrats on 100 followers
Congratulations!!
Thanks!
Great post! I found it quite clear and understandable! Will check out the rest
Thanks! They start out super basic.
Congratulations!!!
Thank you!