Easy Tutorial: Computer Programming for DUMMIES -- header files
In this tutorial, I will be showing you how to implement header files into your code in C or C++. If you want to check out the rest of my tutorials in this series, here they are:
Part 11: Colored Text in your Terminal
Part 16: Binary, Bitwise Operators, and 2^n
Part 19: Object Oriented Programming 1 -- Data Structures
Part 20: Object Oriented Programming 2 -- Classes
Part 21: Object Oriented Programming 3 -- Polymorphism
Part 22: Command Line Arguments
Why use header files?
When working on large projects, it is smart to break up your code into multiple files. This is much easier to read and interpret than stuffing all of it into one file.
Including a header file
Including a header file into your code is similar to including a system file. The only difference is that you use double quotes instead of chevrons. Here is the syntax:
#include "header.h"
- This includes a header file called header.h
Ensuring that the header isn't included more than once
If the header file accidentally gets included twice, all of the variables and functions will be declared twice. This will present an error. There is an easy way to fix this.
Place this at the beginning of the header file:
#ifndef HEADER_H
#define HEADER_H
- "HEADER_H" can be replaced with any name you want
Place this at the end of the header file:
#endif
What these 3 lines of code mean, is that if "HEADER_H" is already defined, ignore the following code. This is called a wrapper.
Here is a simple program I wrote to implement a program with a header file:
main.cpp -- the file with main()
#include <iostream>
#include "header.h"
using namespace std;
int main()
{
Rectangle r;
r.height = 5;
r.width = 4;
cout << "Area of rectangle: " << r.height * r.width << endl;
return 0;
}
- creates a rectangle object
- defines the dimensions of the rectangle
- prints the computed area of the rectangle
header.h -- the header file
#ifndef HEADER_H
#define HEADER_H
class Rectangle
{
public:
int height;
int width;
};
#endif
- declares the class Rectangle
- int height --> member to hold the height of the rectangle
- int width --> member to hold the width of the rectangle
Here is the output of the program:
[cmw4026@omega test]$ g++ main.cpp header.h
[cmw4026@omega test]$ ./a.out
Area of rectangle: 20
[cmw4026@omega test]$
- note that I compiled the program with both files
I hope this was helpful! Leave any suggestions in the comments!
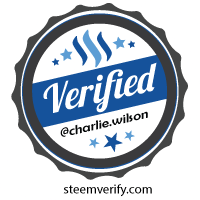
Keep up the good work. I resteemed this. I also referenced you in my latest tutorial post along with some other people.
Thanks a lot for the support! I'll return the favor as much as I can.