Easy Tutorial: Computer Programming for DUMMIES -- command line arguments
In this tutorial, I will show you what command line arguments are and how to implement them in a C or C++ program. If you want to check out my previous tutorials in this series, here they are:
Part 11: Colored Text in your Terminal
Part 16: Binary, Bitwise Operators, and 2^n
Part 19: Object Oriented Programming 1 -- Data Structures
Part 20: Object Oriented Programming 2 -- Classes
Part 21: Object Oriented Programming 3 -- Polymorphism
What are command line arguments?
Command line arguments are arguments that you can give to certain programs before they run. They are placed after the command to run an executable. For Linux, the command to run an executable is "./"
Here is an example, running an executable called a.out with two command line arguments:
./a.out 4 6
- the arguments are 4 and 6
Implementing this in a C program
So how do we tell our program that it should be expecting command line arguments? We just have to include a few arguments when main() is declared.
Here is what it looks like:
int main(int argc, char* argv[])
{
return 0;
}
- argc and argv are standard names for these arguments
- argc --> an integer that holds the number of command line arguments
- in our example, argc would equal 3
1: ./a.out
2: 4
3: 6
- in our example, argc would equal 3
- argv --> a string array that holds the arguments in the form of a string
- argv[0] = "./a.out"
- argv[1] = "4"
- argv[2] = "6"
Why is it important to have argc? We need to know how many arguments there are, because if we call argv[3] (or a larger value) in our example, we would get an error.
Here is a program I wrote to to implement command line arguments:
#include<stdio.h>
int main(int argc, char* argv[])
{
int x;
for(x = 0; x < argc; x++)
printf("Argument %d is: %s\n", x, argv[x]);
return 0;
}
Here is the output:
[cmw4026@omega test]$ gcc arguments.c
[cmw4026@omega test]$ ./a.out 5 2 banana 69 more arguments
Argument 0 is: ./a.out
Argument 1 is: 5
Argument 2 is: 2
Argument 3 is: banana
Argument 4 is: 69
Argument 5 is: more
Argument 6 is: arguments
[cmw4026@omega test]$
Here is another program I wrote. This shows you how to safely make sure a program does not execute unless it has a certain amount of arguments. First, it makes sure that only two arguments were included (besides the command to run the executable), then it adds them together and prints the value.
#include<stdio.h>
int main(int argc, char* argv[])
{
if(argc < 3 || argc > 3)
{
printf("usage: ./a.out number1 number2\n");
return 0;
}
int num1 = atoi(argv[1]);
int num2 = atoi(argv[2]);
printf("The two arguments added together equals: %d", num1 + num2);
printf("\n");
return 0;
}
Here is some sample output:
[cmw4026@omega test]$ gcc arguments2.c
[cmw4026@omega test]$ ./a.out
usage: ./a.out number1 number2
[cmw4026@omega test]$ ./a.out 7
usage: ./a.out number1 number2
[cmw4026@omega test]$ ./a.out 7 5
The two arguments added together equals: 12
[cmw4026@omega test]$ ./a.out 50 25
The two arguments added together equals: 75
[cmw4026@omega test]$ ./a.out 50 25 100
usage: ./a.out number1 number2
[cmw4026@omega test]$
- If the wrong amount of command line arguments was given, a usage statement was displayed.
I hope that was helpful! Leave any suggestions in the comments.
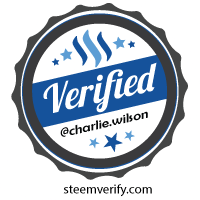