The Connection Between Music and Code
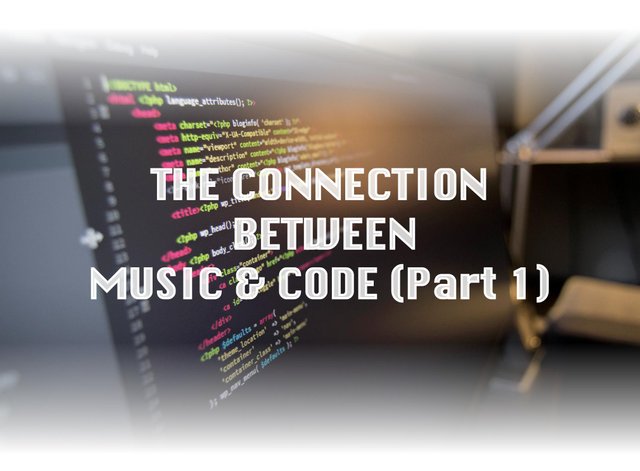
In the Music and Math series, I assume that you have an instrument nearby to play chords or notes associated with a song.
What if you don't have any instruments? I will use this series to explore concepts that allow us to approach music from a programming perspective and even make bots that automatically generate music (stretch goal).
The general approach will be to create a runway of theoretical concepts and attempt to get code to catch up to our imaginations.
A Guitar String As A Function
If we take the E string of a guitar, it has predetermined set of inputs and outputs, which makes it perfect to map out as a function. The open fret (0) gives us the E note. The first fret gives us the F note, and the second fret is F sharp. This continues all the way up the E string fret.
E string Fret | Note |
---|---|
0 | E |
1 | F |
2 | Gb |
3 | G |
4 | Ab |
5 | A |
6 | Bb |
7 | B |
8 | C |
9 | Db |
10 | D |
11 | Eb |
The function E contains all the notes that can be played, and all scales as well. E major scale is E(0,2,4,5,7,9,11). Bb major scale is E(6,8,10,11,1,3,5). As I write these indices there is a subtle pattern to the numbering. In music composition we call the jumps between notes, whole steps or half steps.
For this music programming post, we can say the whole step is adding 2 to the index and a half step is adding one. Major scales are composed with these note jumps (W = Whole Step, H = Half Step): W, W, H, W, W, W, H.
Similarly, in our program, scales can be made by incrementing the index like this: +2, +2, +1, +2, +2, +2, +1.
Single Guitar String Chords
The C chord (C, E, G), with our function can be referred to as E(8), E(0), and E(3).
The Heart And Soul song chord progression can be notated like this.
C chord: E(8), E(0), and E(3)
Am chord: E(5), E(8), and E(0)
F chord: E(1), E(5), and E(8)
G chord: E(3), E(7), and E(10)
Every Guitar String Referenced From The E string
You can use the E string to also create other strings as long as you provide the correct starting point.
I use the sentence "Even A Diamond Gets Better Edges" to remember my guitar strings that are E, A, D, G, B, E.
With our E string function we can map out the other 4 strings this way:
A string: E(5) to E(11) and E(0) to E(4)
D string: E(10) to E(11) and E(0) to E(9)
G string: E(3) to E(11) and E(0) to E(2)
B string: E(7) to E(11) and E(0) to E(6)
Beyond the Major Scale
At this point in the post, a lot of the concepts like strings and scales seemed strange and even unnecessary. In code, you can do whatever the hell you want!
I am going to take a step back and explain my excitement over scales. Scales are the toolbox for any composer to make music. When you walk up to a piano for the first time and sweep your hand to hit every key, you are playing the chromatic scale. If you hit only black keys, you are playing the pentatonic scale.
Each scale on it's own has a predictable ability to generate some response out of humans.
If these scales are the toolbox, then chords are the tools with which composers build songs. Some songs are written with only one chord. Some only have four chords. An old friend of mine made fun of Nirvana (not friends anymore) and said they only used 4 chords in their songs. Not only was this false, but only true in the parts of the songs he heard.
Take Smells like Teen Spirit, for example. The chorus has 4 chords, but the section where Kurt Cobain says "Hey, yay" has 1 additional chord (F major) my former friend didn't hear, because he didn't listen all the way through the song.
If the scale of E minor (E, F#,G,A,B,C,D) is the toolbox for the Smells Like Teen Spirit song then the chords E minor, A minor, G major and C major are the foundations, studs, floors and nails of the song. Those chords are pretty much everything accept the soul and decoration living inside the song.
The melody comes from the scale, but weaves through the chords to connect with the listener. This effect is so powerful, I can see Cobain in the Smells Like Teen Spirit video and his blond hair covering his face as he sings. The melody is strong enough to lock in memories and experiences than be recalled years later.
Here is the melody for some of "Teen Spirit".
B D E A E D C B I'm worse at what I do best C B A G A B A G F And for this gift, I feel blessed B D E A D E D C B Our little group has always been C B A G A B A G F And always will until the end
I went into this little side rant, because there is a great challenge when you take code and music to a much higher level. When you go from composing songs to composing musicians you need a starting point.
You need to start deconstruction at some source of knowledge.
Why only stick to the old conventions of making scales?
I could make random scales. Or make scales based on constants like pi and e. Make scales based on weather forecasts.
Let's proceed with making a PI scale for PI to 11 digits (3.14159265358).
Well it is pretty easy.
Just toss in every desirable digit of PI into the E string function: E(3,1,4,1,5,9,2,6,5,3,5,8).
What if we wanted to make a scale based today's temperature?
Let's assume the following temperature range:
Hour | Temperature |
---|---|
6 | 70° |
9 | 72° |
12 | 74° |
15 | 76° |
18 | 78° |
21 | 76° |
24 | 74° |
I would love it if every day was like this perfect day in the 70s. In fact, I will call this the Perfect Day scale. To reign in this temperatures into numbers, we'll have to normalize the numbers a bit. One way to turn these values into usable inputs is to choose a base temperature and rewrite the values based on the difference between the actual temperature and the base temperature.
With a base temperature of 70°, we can say the value for noon will be 6 (76 minus 70). With this trick, we can proceed to make our Perfect Day scale: E(0,2,4,6,8,6,4).
I have tried using Javascript in steemit posts, but I haven't had any success. It would be nice to have a way to embed code here (in some sort of safe sandbox) and have you execute it on your own without any downsides.
Processing Examples
I've decided to go with Processing.
Warning! If there is a software release before alpha... like pre-alpha... this software will fall in that bucket. It works, but the cases to prevent crashes haven't been flushed out. If you end up with a crash, just force quit the software and restart.
Here is my task list for this post:
- Create a function that plays a tone based on a number input. The number will be the fret number of the E string of a guitar.
- Play a PI scale.
- Make a song based on temperature data.
E String Function
Some of the first roadblocks I face is that scientific pitch notation has each note pegged at a specific frequency. I wanted to just multiply one frequency by a constant to easily derive the target frequency.
Well I knew that if I doubled E2 I would get it's octave, which is E3. The 5th of the E scale is A2, which is 1.5 times E2. And each half step is 1.05 times higher than the previous note. My equation will take The frequency of E2 times the fret number and 1.05.
Visit this paste for the code. If you ran that in Processing, you would have a program that plays a tone for every number you type. The space bar clears the screen.
(couldn't get the code to embed here with pastebin so I submitted a bug.)
Here is a video of the code in action.
The Temperate Musician
Writing software to automatically grab temperatures for a certain zip code and translate that to music is a stretch for me. I have the E string function working, and getting this temperate musician up and running requires getting temperatures for a specific location and playing those temperatures as music.
It is late here, so I will save this work for a future post.
I hope you enjoyed this.
Which programming language would you use to make your code instrument?
Follow me to see more variations on this type of post.
Music & Math series:
Now, I have to admit, especially since I have been into that subject for so many years, can't wait to hear more developed version of this. Thank you for the solid info so far, dedication and your musical acumen. Namaste :)
I tried processing once, and I know a bit of Javascript. In my next post, I am going to focus more on the UI and export video directly from Processing.
Thanks for this comment. I do love music, and I want to push my knowledge further.