Implementing !info Command | Steem Discord Bot Python #6
Welcome back, developers.
Greetings to all Steem enthusiasts! Today, we will learn how to get the username from the discord command and show the information for this username using the SDS API.
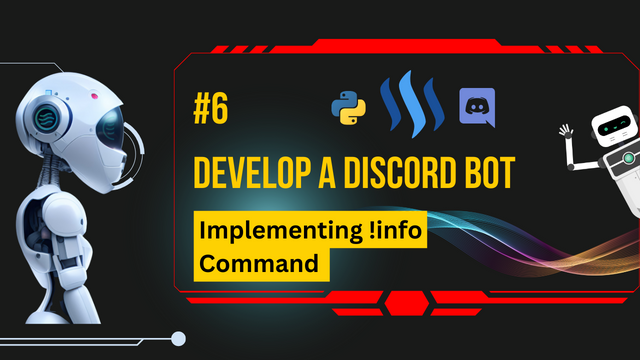
Summary |
---|
We established the necessary environment for developing a Python-based Discord bot during our first lecture. In our second lecture we create a discord bot token, configure the bot and generate the invite link. We used the invite link and added the bot to our server. In our third lecture, we will bring the Community-Bot online in our local environment.
In the 4th lecture, we successfully developed our initial bot command, !info. We also learned how to create functions and retrieve data using the requests library in 5th lecture. Today we will fetch data for any user and show in the discord.
Procedure |
---|
- First, we need to extract the username from the Discord command. We will allow two properties in the command. The first one is the command itself, and the second one is the parameter, which in our case will be the username.
We will check if the length of the props is greater than or equal to 2. We will use the len() built-in function in Python to determine the length of an array or string. Additionally, we will convert the username to lowercase and remove the "@" symbol to prevent any issues if a user mistakenly uses uppercase letters or adds "@" at the beginning of their username.
if len(props) >= 2:
username = str(props[1]).lower().replace('@', '')
await steemfun.get_account_ext(username)
- We obtained the user profile data using the SDS API. I have developed a dedicated function for everyone to utilize. This function will convert the SDS API response into a simple JSON object and array.
add this function to the steemfun.py file
def map_sds_response(response):
""" Returns the mapped rows and columns of given SDS response
:param response: SDS response
:type response: json, dict
"""
result = response.get('result')
if not result:
result = response
cols = result.get('cols')
rows = result.get('rows')
if not cols:
if not rows:
return result
else:
return rows
keys = list(cols.keys())
redefined_data = []
for row in rows:
mapped_obj = {}
loop = 0
for value in row:
mapped_obj[keys[loop]] = value
loop += 1
# noinspection PyBroadException
try:
if mapped_obj.get('author_reputation'):
mapped_obj['author_reputation'] = round(mapped_obj['author_reputation'], 2)
if mapped_obj.get('observer_reputation'):
mapped_obj['observer_reputation'] = round(mapped_obj['observer_reputation'], 2)
if mapped_obj.get('reputation'):
mapped_obj['reputation'] = round(mapped_obj['reputation'], 2)
except Exception:
pass
redefined_data.append(mapped_obj)
return redefined_data
- Now return the response by converting it for getAccountExt as shown here.
async def get_account_ext(self, username):
if username is None:
return
else:
api = self.utils.sds_base + f"/accounts_api/getAccountExt/{username}"
response = requests.get(api).json()
return map_sds_response(response)
- We can use any property provided by the SDS API. We need to use try except to handle any unexpected error and we will also add the typing() to set the bot in typing state.
# !info command
if command == utils.commands[0]:
if len(props) >= 2:
username = str(props[1]).lower().replace('@', '')
await message.channel.typing()
try:
account_data = await steemfun.get_account_ext(username)
print(1122, account_data['name'])
if account_data is not None:
await message.reply(f'username: {account_data.get("name")}\n'
f'Steem: {account_data.get("balance_steem")}\n'
f'SBD: {account_data.get("balance_sbd")}\n'
f'VP: {account_data.get("upvote_mana_percent")}\n'
f'RC: {account_data.get("rc_mana_percent")}\n')
except Exception as e:
await message.channel.send("Error " + str(e))
- Let's run the bot and check if the bot is sending the information properly or not.
It's working like a charm. See you in the next lecture.
Github |
---|
Steem Discord Bot Series |
---|
Lecture #1: Develop a Discord bot for your Steem Community
Lecture #2: How to Add a Bot to Your Discord Server
Lecture #3: Bring the Steem Discord Bot Online
Lecture #4: First Bot Command
lecture #5: Retrieving Steemit Account Information using SDS
SteemPro Official |
---|
Download SteemPro Mobile
https://play.google.com/store/apps/details?id=com.steempro.mobileVisit here.
https://www.steempro.comSteemPro Discord
Official Discord Server
Cc: @blacks
Cc: @rme
Cc: @hungry-griffin
Cc: @steemchiller
Cc: @steemcurator01
Cc: @pennsif
Cc: @future.witness
Cc: @stephenkendal
Cc: @justyy
Best Regards @faisalamin
Upvoted! Thank you for supporting witness @jswit.