스팀 앱 개발기 #7 - ProfileFragment 그리고 WalletFragment에 데이터 바인딩 적용
지난 포스트에서는 태그별 내용을 보여줄 TagsFragment에 데이터 바인딩 적용하는 과정을 보여 드렸는데요. 이번에는 사용자 프로필과 포스트를 보여줄 ProfileFragment 그리고 지갑 내용 보여줄 WalletFragment에 데이터 바인딩 적용하는 과정을 보여 드리겠습니다.
지금은 세 개의 프래그먼트들의 화면들이 단순히 텍스트 뷰 하나만 가지고 있습니다. 그래서 두 프래그먼트들의 작업 과정은 어제 했던 것과 동일합니다. 데이터 바인딩 적용 전후로 코드가 어떻게 바뀌는지를 보는 것에 의미가 있겠습니다.
ProfileFragment에 데이터 바인딩 적용 과정
fragment_profile.xml 파일 변경 전
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_profile"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:textAlignment="center"
android:textSize="20sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
fragment_profile.xml 파일 변경
<?xml version="1.0" encoding="utf-8"?>
<layout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
<variable
name="viewModel"
type="lee.dorian.steem_ui.ui.profile.ProfileViewModel" />
</data>
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_profile"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:textAlignment="center"
android:text="@{viewModel.text}"
android:textSize="20sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
</layout>
ProfileFragment 클래스 변경 전
class ProfileFragment : Fragment() {
private var _binding: FragmentProfileBinding? = null
// This property is only valid between onCreateView and onDestroyView.
private val binding get() = _binding!!
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
val profileViewModel =
ViewModelProvider(this).get(ProfileViewModel::class.java)
_binding = FragmentProfileBinding.inflate(inflater, container, false)
val root: View = binding.root
val textView: TextView = binding.textProfile
profileViewModel.text.observe(viewLifecycleOwner) {
textView.text = it
}
return root
}
override fun onDestroyView() {
super.onDestroyView()
_binding = null
}
}
ProfileFragment 클래스 변경
class ProfileFragment : Fragment() {
private var _binding: FragmentProfileBinding? = null
// This property is only valid between onCreateView and onDestroyView.
private val binding get() = _binding!!
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
val profileViewModel =
ViewModelProvider(this).get(ProfileViewModel::class.java)
_binding = DataBindingUtil.inflate(this.layoutInflater, R.layout.fragment_profile, null, false)
binding.viewModel = profileViewModel
return binding.root
}
override fun onDestroyView() {
super.onDestroyView()
_binding = null
}
}
WalletFragment에 데이터 바인딩 적용 과정
변경 전 파일 내용은 ProfileFragment와 동일하므로 생략합니다.
fragment_wallet.xml 파일 변경
<?xml version="1.0" encoding="utf-8"?>
<layout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<data>
<variable
name="viewModel"
type="lee.dorian.steem_ui.ui.wallet.WalletViewModel" />
</data>
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/text_wallet"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:text="@{viewModel.text}"
android:textAlignment="center"
android:textSize="20sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
</layout>
WalletFragment 클래스 변경
class WalletFragment : Fragment() {
private var _binding: FragmentWalletBinding? = null
// This property is only valid between onCreateView and
// onDestroyView.
private val binding get() = _binding!!
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
val walletViewModel =
ViewModelProvider(this).get(WalletViewModel::class.java)
_binding = DataBindingUtil.inflate(this.layoutInflater, R.layout.fragment_wallet, null, false)
binding.viewModel = walletViewModel
return binding.root
}
override fun onDestroyView() {
super.onDestroyView()
_binding = null
}
}
[광고] STEEM 개발자 커뮤니티에 참여 하시면, 다양한 혜택을 받을 수 있습니다.
Upvoted! Thank you for supporting witness @jswit.
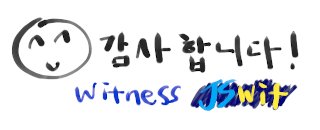