DOM manipulation with D3.js (Compare with Vanilla JS)
In this tutorial, I am goinng to cover DOM manipulation with D3. This is the continuation of my D3 tutorial. Read my previous tutorial on Introduction to D3.js to setup the project.
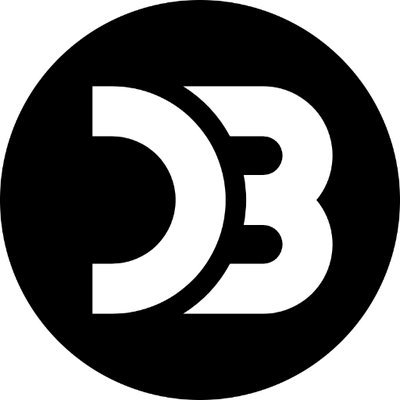
Source from Twitter
Prerequisite
- Basic understanding of JavaScript
- Basic understanding DOM manipulation with JavaScript / jQuery
DOM Manipulation with D3.js
D3.js is a powerful JavaScript Library for Data-Driven Documents. The usage mainly for Data Visualization with DOM manipulation of HTML, CSS and SVG.
Since D3 are able to do DOM manipulation, I will do comparison of D3 and Vanilla JavaScript side by side to see how D3 syntax works.
Demo of this tutorial at Codepen
(select
or selectAll
) and querySelector
In js, we will use document.getElementById
or document.querySelector
to select a specific DOM element.
d3 | js |
---|---|
let element = d3.select('.element'); | let element = document.querySelector('.element'); |
let elements = d3.select('p'); | let elements = document.querySelector('p'); |
classed
and className
When we want to add class to DOM element.
d3 | js |
---|---|
d3.select('#el').classed('test', true); | document.getElementById('el').className += 'test'; |
style
When we want to add inline styling to DOM element.
d3 | js |
---|---|
d3.select('.container').style('background', 'yellow'); | document.querySelector('.container').style.cssText = 'background: yellow'; |
html
and innerHTML
When we want to add html tag and text into DOM element.
d3 | js |
---|---|
d3.select('.container').text('Hello World'); | document.querySelector('.container').innerText = 'Hello World'; |
select
with callback (in D3)
This feature only available to D3 instead of Vanilla JS, this allow us to show dynamic styling. In this case, idx
is the order of the element. If it is odd, it will be grey, if it is even, it will be white.
d3.selectAll('li').style('background-color', function(_, idx) {
return idx % 2 === 0 ? 'grey' : 'white';
});
Event Listener with D3
and JS
d3 | js |
---|---|
d3.select('button').on('click', function() {}); | document.querySelector('button').addEventListener("click", function() {}) |
remove
d3 | js |
---|---|
d3.select('.paragraph').remove(); | document.querySelector('.paragraph').parentNode.removeChild(document.querySelector('.paragraph')) |
Making a simple app with D3 instead of jQuery
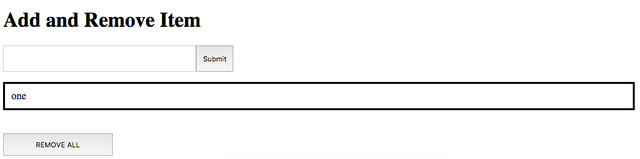
Demo at Codepen
in html
<h1>Add and Remove Item</h1>
<form style="display: flex;">
<input type="text" style="font-size: 30px;" />
<button type="submit" style="padding: 10px;margin: 0;">Submit</button>
</form>
<div class="result"></div>
<button class="remove">REMOVE ALL</button>
in js
with D3
// IF USING NPM: (uncomment next line)
// import * as d3 from 'd3';
// Event listener
d3.select('form').on('submit', function() {
d3.event.preventDefault();
let input = d3.select('input');
if (input.property('value')) {
d3
.select('.result')
.append('p')
.classed('paragraph', true)
.style('border', '3px solid black')
.style('padding', '10px')
.text(input.property('value'));
input.property('value', '');
}
});
// remove
d3
.select('.remove')
.style('padding', '10px 50px')
.style('margin-top', '20px')
.on('click', function() {
d3.selectAll('.paragraph').remove();
});
In the Submit Event Listener
,
The form listen to submit
event.
On submit
event, d3.event.preventDefault()
prevent the default form submit behaviour, which is a full page refresh for submitting the form.
Then, we check that input
has value with d3.select('input').property('value')
If there is value in input, then we append a p
tag with the input into result
class div.
In the Click Event Listener
,
Once the button is click, the paragraph class is cleared.
Before I learn D3.js, I thought D3.js was just a library for Data Visualization. I was amaze by the way the DOM manipulation done by D3.js, and in this tutorial, I showed you this library can do more than just that. Just take a look at bl.ocks.org, there are so many amazing project are done in D3.js .
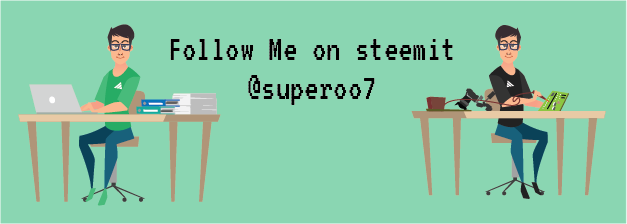
Posted on Utopian.io - Rewarding Open Source Contributors
posting is great hopefully steemit triumphant
thanks
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @superoo7 I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
it is a very interesting comparison. please check out my javascript tutorial too. thanks!
Get Paid For Your Comments 💰 🤑 https://steemit.com/steemit/@a-a-a/get-paid-for-your-comments