Deep Dive into Object and Function with TypeScript and JavaScript
In this post, I am going to deep dive into Modern JavaScript and TypeScript way of dealing with Object and Function. Bonus: Generics in TypeScript.
Previous Topic:
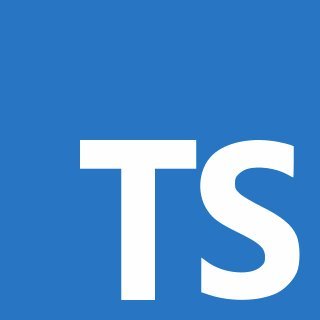
Source
Object literal
In JavaScript/TypeScript
Object is a quite common data type in JavaScript and TypeScript. In this section, I will talk about some of the feature that TypeScript had, but not all JavaScript platform has.
- normal object
let obj = {
round: true,
name: "circle",
edge: 0
};
- object in ES6
Object in ES6 offer prototype inside the object, string interpolation and more...
let world = "Earth";
let animal = {
__proto__: AnimalObject.prototype,
species: "Dog",
name: "Bob",
world,
[`${world}`]: true,
};
- simple object destructure
In ES6, we can destructure object with this method.
let someone = {
name: "Bob",
age: 3
};
const {name, age} = someone;
console.log(`${name} is ${age} years old`); // Bob is 3 years old
- deep nested object destructure
If the object is deeply nested, it still can be done.
let someone = {
name: "Bob",
age: 30,
skills: {
web_dev: {
html: {experience: "4 years"},
css: {experience: "1 year"},
javascript: {experience: "1 year"}
}
}
};
let {
name,
age,
skills: {web_dev: skill}
} = someone;
output:
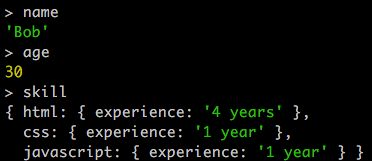
- Rest and Spread Properties
This is a bit like pattern matching in any functional language (e.g. Elixir), where we seek what the data looks like on our right, and we try to extract out on the left.
This is available to JS just like how spread operator in arrays. These features are available to TypeScript.
Still in proposal stage 3. I tested with node v8.9.2
Rest (extract out)
let colors = { r: 0, g: 120, b: 100 };
let { r, ...others } = colors;
console.log(others); // { g: 120, b: 100 }
Spread (merge in)
let rgb = { r: 0, g: 120, b: 100 };
let rgba = { ...rgb, a: 0.2}
console.log(rgba); // { r: 0, g: 120, b: 100, a: 0.2 }
This is one of my favourite feature in the proposal and TypeScript.
Imagine this case of scenario, you had to add a new a
into rgb. The old way of doing it:
let rgb = { r: 0, g: 120, b: 100 };
let rgba = rgb;
rgba.a = 0.2
The code will become more messier when there is more properties!.
- Getter and Setter
In OOP language, this is the common approach to access private data to achieve Encapsulation.
Getter
- return value of the property
Setter
-set the value of the property
var employee = {
first: "Boris",
last: "Sergeev",
get fullName() {
return this.first + " " + this.last;
},
set fullName(value) {
var parts = value.toString().split(" ");
this.first = parts[0] || "";
this.last = parts[1] || "";
},
email: "[email protected]"
};
console.log(employee.fullName); //Boris Sergeev
employee.fullName = "Alex Makarenko";
console.log(employee.first);//Alex
console.log(employee.last);//Makarenko
console.log(employee.fullName);//Alex Makarenko
Functions Types
In TypeScript, when we want to define how a function shape looks like.
let register: (firstname: string, lastname: string) => User; // declare function type
register = (firstname, lastname) => (new User()); // a function value
Interface to define function types
interface newUser {
(firstname: string, lastname: string): User
}
let register: newUser = (firstname, lastname) => (new User());
Optional Parameters
And TypeScript, assumes every argument in the function is required. However, you can use optional to state that that argument is optional.
The syntax is just like SwiftLang with ?
.
function createAnimal(
eyes: number,
wings?: number,
tail?: boolean
) {...}
Default Parameters Value
Same as ES6, there is default value for TypeScript.
function createAnimal(
eyes: number,
wings?: number,
tail?: boolean,
alive: boolean = true
) {...}
Rest Parameters
Rest
is what I have shown just now.
function addition(base: number, ...others: number[]) {...};
addition(1,2,3,4,5);
addition(1,2,3);
Generics in TypeScript
- allow us to reuse code accross many types, interfaces and functions.
function makeFive<T>(x: T): T[] {
return [ x, x, x, x, x ];
}
let num: number[] = makeFive(3);
let foods: string[] = makeFive("FOOD");
- Array also can be express this way
let cards = Array<[Suit, CardNumber]>(52);
- Promise also
let data: Promise<Response> = fetch("https://google.com");
That's the end of the description on Object and Function with Modern JavaScript and TypeScript. TypeScript already have more features than JavaScript, and JavaScript team (TC39) are slowly implement some features from TypeScript!
Posted on Utopian.io - Rewarding Open Source Contributors
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by superoo7 from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, someguy123, neoxian, followbtcnews/crimsonclad, and netuoso. The goal is to help Steemit grow by supporting Minnows and creating a social network. Please find us in the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP. Be sure to leave at least 50SP undelegated on your account.
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @superoo7 I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Get your post resteemed over 90000+ followers and get upto $19+ value Upvote. Your post will skyrocket and give you maximum exposer.
See our all pakages at: