TypeScript Data Types
TypeScript offer a lot more types than typical JavaScript. From people who experience with C or Java, TypeScript offers Tuples and Enums.
Learn more about TypeScript at my first post about Getting started with TypeScript
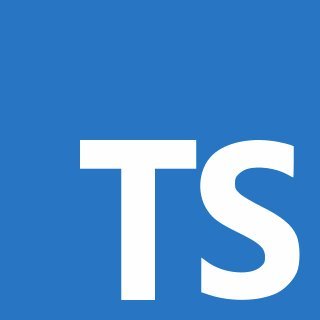
Source
Before we start
This post is about TypeScript types. So, you need an editor, workflow or just a playground to test out the code.
Method 1: Go to typescript playground
This playground can let you get started with TypeScript quickly. In the left is the code in TypeScript, and on the right is the compiled JavaScript
Method 2: use Code Runner Plugin in Visual Studio Code
This plugin required 2 global npm package which is ts-node
and typescript
.
to install:
npm install -g ts-node
oryarn global add ts-node
npm install -g typescript
oryarn global add typescript
if you are not familiar with npm or yarn, check out my tutorial
Press the play button on VS Code to test out your code without any tsc
workflow setup.
Method 3: Use tsc
Install typescript globally with npm.
npm install --g typescript
tsc --version
Compiled a .ts
file, which will generate a js file
tsc index.ts
Test your .js
file wither with node or browser
node index.js
<script src="./index.js"></script>
Basic Type (in TS)
The basic type in TypeScript:
string
number
boolean
let newString: string = "Hello World!";
let newNumber: number = 12;
let newBoolean: boolean = true;
let val: boolean | number = 12;
val = true;
Object
Object Shape (Excess Property) (in TS)
This will raise the flag.
let myObj: {a: string, b:string, c:string};
myObj = {
a: "hello",
b: "test",
c: "everyone",
d: "fail"
};
The error was:
error TS2322: Type '{ a: string; b: string; c: string; d: string; }' is not assignable to type '{ a: string; b: string; c: string; }'.
Object literal may only specify known properties, and 'd' does not exist in type '{ a: string; b: string; c: string; }'.
To fix this by using as
myObj = {
a: "hello",
b: "test",
c: "everyone",
d: "fail"
} as {a: string, b:string, c:string};
Interface
Interface is like a blueprint for the shape of your variable. It allows us to make a structure and refer to it by name.
interface House {
windows: number,
doors: number,
location: string
};
let myHouse: House = {
windows: 4,
doors 3,
location: "123 new street"
};
Interface will not compile into JavaScript
Change from an interface to another
interface User {
email: string;
password: string;
}
interface VerifiedUser {
email: string;
password: string;
isOnline: boolean;
}
let randomUser: User = {
email: "[email protected]",
password: "password"
}
function modUser(user: User) : VerifiedUser {
let changeUser = user as VerifiedUser;
changeUser.isOnline = true;
return changeUser;
}
const verUser = modUser(randomUser);
console.log(verUser);
Type any
- Allow any data type
- Normally use on mutable data
- Back to Javascript, dynamic language style
- Quick migration strategy from JS to TS for existing code base
let value: any = true;
value = 4;
value = "Hello";
Type never
- Nothing can be assign to
never
- When we use it, is normally along side for error detection
Class
Before I start about classes in TypeScript, I will explain a bit on how classes work in JavaScript.
JavaScript style of Class
Before I start about class in JS, you can test out these codes at babel website. On the left is the latest feature, on the right is the compiled version
- New added feature.
- Javascript new feature in ES6.
- Javascript used prototype for making Class.
- Like any OOP language, there is constructor.
class Animal {
constructor(species) {
this.species = species;
}
}
let dog = new Animal("Dog");
console.log(dog);
The output is Animal { species: 'Dog' }
- methods in the class.
static
method. (If you are familiar to OOP language like Java this are a little bit different)
static
is a function attach to the constructor (to the class itself), does not require any instance to be created.
This is a new feature, require babel transform to work
I tested this with node v8.9.2
class Animal {
constructor(species) {
this.species = species;
}
toPrint() {
return `Animal: ${this.species}`;
}
static createCat() {
return new Animal("Cat");
}
}
let kat = Animal.createCat();
console.log(kat);
console.log(kat.toPrint());
The output is :
Animal { species: 'Cat' }
Animal: Cat
The
static
only available to class Animal, which mean if you trycat.createCat()
, it will be an error.
- Public and Instance field
public field
- does not require instance
Instance field
- putting a property on an instance
This is the new feature in stage 2 where syntax might change in future.
Require babel to work
class Animal {
static _id = 0;
living = true;
constructor(species) {
this.species = species;
this.id = Animal._id++;
}
}
let cat = new Animal("Cat");
let dog = new Animal("Dog");
console.log(cat);
console.log(dog);
The output:
Animal { living: true, species: 'Cat', id: 0 }
Animal { living: true, species: 'Dog', id: 1 }
- Inheritance with
extends
extends
use to inherit from a class
super
use to call method from parent class.
class Animal {
constructor(species) {
this.species = species;
}
}
class Dog extends Animal {
constructor(name, species) {
super(species);
this.name = name;
}
}
let doggy = new Dog("Bob", "Dog");
console.log(doggy);
The output: Dog { species: 'Dog', name: 'Bob' }
- Mixins
Mixins are like templates for classes
const Animal = (sound) => class {
sound() {
return `${sound}`;
}
}
class Dog extends Animal("Bark") {
constructor(species) {
super();
this.species = species;
}
}
let dog = new Dog("dog");
dog.sound();
The output: 'Bark'
.
Typescript Style of Class
Going back to class in typescript
class Animal {
species: string;
legs: number;
eyes: number;
constructor(species: string,
legs: number,
eyes: number) {
this.species = species;
this.legs = legs;
this.eyes = eyes;
sound() {
return 'ouw!';
}
}
let dog = new Animal('dog', 4, 2);
Enum (in TS)
Enumerables can be quite common to be found in Java, C, C++ and Swift. TypeScript also offer this features.
- Enum used to define a type of new data type that consist of ordered member.
- Typescript will use a 'hacky' way to create enum.
- On the left is TypeScript, and on the right is the compiled JavaScript.
TypeScript | JavaScript |
---|---|
|
|
Arrays (in TS)
- Almost the same as JavaScript.
- Can declare type.
let arr: number[] = [1, 2, 3]
Tuples (in TS)
- A fixed length of arrays.
- Useful for destructuring.
- Order is important.
let tup: [string, number] = ["abc", 12];
Type Alias
- Useful for alternative to interface.
type BMI: [number, number, number];
let person: BMI = [80, 1.73, 26.73];
About Me
I am Lai Weng Han (Johnson), you can find me on Twitter.
Posted on Utopian.io - Rewarding Open Source Contributors
Wow! This is awesome, Tnx for bringing out this piece of information, am going tobtry this out right away.
I wish you all the best STEEMMING in 2018.
Kudos @superoo7.
Thanks for finding it useful. More series to come~
Hope to read more.... Smiles
Aiit.
There is some issue reading on steemit.com , I would suggest go to utopian.io for better experience (just a table)
https://utopian.io/utopian-io/@superoo7/typescript-data-types
Wow, thanks for sharing!
hope you find it useful
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thanks moderator
Hey @superoo7 I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x