Part 20: Plotting Account's Total Generated Post Rewards Since Creation
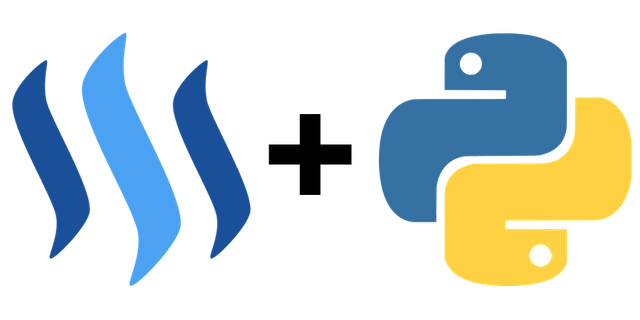
This tutorial is part of a series where different aspects of programming with steem-python
are explained. Links to the other tutorials can be found in the curriculum section below. This part will focus on calculating the total rewards an account has generated with blog posts since its creation!
What will I learn
- How to create a dictionary with dates as keys
- How to save blog post rewards to a dictionary
- How to sum generated blog post rewards
- How to use
pyplot
to create a graph
Requirements
- Python3.6
steem-python
Difficulty
- Intermediate
Tutorial
If you find it easier to follow along this way you can get the entire code for this tutorial from here, where I have split everything up into the relevant sections.
Creating a dictionary of dates
First things first, we need to create a dictionary of dates from the account's creation until now, where each day is a key. As you might recall an account has a "created" attribute, so getting the account creation date and turning it into a datetime
object is easy. Once we have this we can subtract it from today's date to get the total amount of days passed, like so
account = "steempytutorials"
created = parse(Account(account)["created"]).date()
today = datetime.today().date()
days = today - created
>>> 32 days, 0:00:00
Once we have this we can simply iterate over all dates between the account's creation and today by adding the amount of days using timedelta
to the account's creation date. This looks like this
dates = {}
for day in range(days.days + 1):
# Convert date to a string so it can be used as key
dates[str(created + timedelta(days=day))] = 0
which results in our dictionary looking like this
{
"2017-12-30": 0,
...,
"2018-01-31": 0
}
Iterating over all blog posts
We now have our dictionary, so we can start getting each blog post's total generated rewards. To do this we can reuse the code we used in part 18, as seen below
while post_limit < Account(account)["post_count"]:
print(post_limit, post_limit + 500)
for post in steem.get_blog(account, post_limit, 500):
post = Post(post["comment"])
if post.is_main_post() and post["author"] == account:
# COUNT TOTAL REWARD AND ADD TO DICTIONARY
post_limit += 500
We want to replace the comment with some code that gets the post's total generated reward. If a post is less than 7 days old, then this reward will still be pending, but otherwise it has already been paid out to the author, curators and beneficaries (if applicable). So what we will do is simply check if an account still has pending rewards and use that as the payout, and if that's not the case, then we will add the author's payout with the curator's payout together and use that instead. Once we have this we obviously want to add this to our dictionary, so we also need to get the day the post was made. To do all this we can use the following code
# Convert post creation date to string
post_date = str(post["created"].date())
# If pending payout then use this
payout = Amount(post["pending_payout_value"]).amount
# Otherwise sum author reward and curator reward
if payout == 0:
payout = (Amount(post["total_payout_value"]).amount +
Amount(post["curator_payout_value"]).amount)
# Add this to the relevant key
dates[post_date] += payout
Printing our dictionary once again gives us the following updated dictionary
{
"2017-12-30": 0,
"2017-12-31": 0,
...,
"2018-01-30": 37.868,
"2018-01-31": 3.843
}
Plotting the graph
Before we can plot this using pyplot
there are a few things we need to do. First of all, to get the x
values we need to convert the keys of the dictionary back to datetime
objects and append them to a list. To do this we can use some list comprehension
x = [datetime.strptime(date, "%Y-%m-%d").date() for date in dates.keys()]
For the y
values we want the total sum of rewards up until that point in time. To do this we can iterate over the dictionary's values and for each index sum the relevant slice.
y = [sum(list(dates.values())[0:i]) for i in range(len(dates.values()))]
This might look a bit complicated at first glance. Imagine it like this if it's a bit too difficult to understand
y = [sum(Post rewards day 1), sum(Post rewards day 1, Post rewards day 2), ..., sum(Post rewards day 1, ..., Post rewards last day)]
Alright, so now we have the x
and y
values all that's left is to plot them! But before we do that, let's also find out when the account generated its first reward (so we can show the date when this happened). We can simply calculate the index of when this happened and use this to show it as an xtick
like so.
def first_reward():
for i, reward in enumerate(y):
if reward > 0:
return i
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
# Set the xticks
plt.gca().xaxis.set_major_formatter(mdates.DateFormatter("%Y-%m-%d"))
plt.gca().xaxis.set_major_locator(mdates.DayLocator())
plt.xticks([x[0], x[first_reward() - 1], x[-1]], visible=True,
rotation="horizontal")
Once we have that, all that's left is actually plotting the graph, which is made very easy with pyplot
plt.plot(x, y)
# Always label your axes!
plt.xlabel("{} to {} for @{}".format(created, today, account))
plt.ylabel("Sum of post rewards generated")
# Add a nice grid show the plot
plt.grid()
plt.show()
which results in the following graph for @steempytutorials.
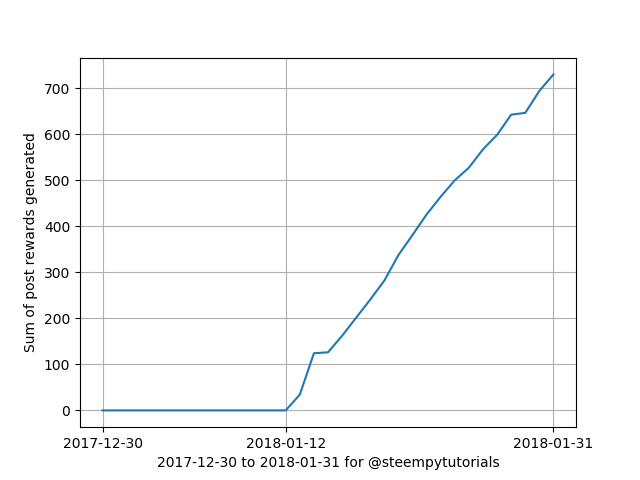
Note: this is not what the author has earned themselves, but what their posts have generated in total (a lot of other factors come in to play if you wanted to calculate this). Also there is a bug if you have more than 500 posts (or it could be intended behaviour), since get_blog()
seems to repeat the most recent 500 posts despite setting a different entry_id
.
Curriculum
Set up:
- Part 0: How To Install Steem-python, The Official Steem Library For Python
- Part 1: How To Configure The Steempy CLI Wallet And Upvote An Article With Steem-Python
Filtering
- Part 2: How To Stream And Filter The Blockchain Using Steem-Python
- Part 6: How To Automatically Reply To Mentions Using Steem-Python
Voting
- Part 3: Creating A Dynamic Autovoter That Runs 24/7
- Part 4: How To Follow A Voting Trail Using Steem-Python
- Part 8: How To Create Your Own Upvote Bot Using Steem-Python
Posting
- Part 5: Post An Article Directly To The Steem Blockchain And Automatically Buy Upvotes From Upvote Bots
- Part 7: How To Schedule Posts And Manually Upvote Posts For A Variable Voting Weight With Steem-Python
Constructing
Rewards
- Part 9: How To Calculate A Post's Total Rewards Using Steem-Python
- Part 12: How To Estimate Curation Rewards Using Steem-Python
- Part 14: How To Estimate All Rewards In Last N Days Using Steem-Python
Transfers
- Part 11: How To Build A List Of Transfers And Broadcast These In One Transaction With Steem-Python
- Part 13: Upvote Posts In Batches Based On Current Voting Power With Steem-Python
Analysis
- Part 15: How To Check If An Account Is Following Back And Retrieve Mutual Followers/Following Between Two Accounts
- Part 16: How To Analyse A User's Vote History In A Specific Time Period Using Steem-Python
- Part 18: How To Analyse An Account's Resteemers Using Steem-Python
- Part 19: Analysing The Steem Blockchain From A Custom Block Number For A Custom Block Count
The code for this tutorial can be found on GitHub!
This tutorial was written by @amosbastian in conjunction with @juliank.
Posted on Utopian.io - Rewarding Open Source Contributors
You seem to read my mind with these tutorials! Thank you so much for putting them together :)
(@themarkymark and I were discussing how to work out the ROI of our efforts on Steemit - this will help a great deal!)
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @steempytutorials I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x