🤖 TUTORIAL - Bots In The Background - Building bots With steem-js #7
This tutorial is part of a series on ‘How To Build Bot’s Using Steem-JS Open Source Library’ it aims to demonstrate practical applications for bots and demystify the code used to create them.
- part 1- auto-liking bot
- part 2 - Curation bot
- part 3 - Auto follow bot
- part 4 - Paid upvote bot
- part 5 - @ Mention Notifier bot
- part 6 - Auto Reward Claimer bot
The completed bot code is here - I know some people find it easier to see everything in one block and follow along that way. ⬅️
Outline 📝
In this tutorial, we’re looking at how to make Steem JS bot that run tasks at certain times of the day. Scheduling bot tasks is particularly useful for repetitive tasks such as claiming rewards, batch voting after power recharges or diversifying tokens. Almost all popular bots on the Steem network will be running in the background within continuously or scheduled to active at certain times.
Requirements
- A plain text editor (I use atom.io )
- Your private posting key - This is found in your wallet on the permissions tab on steemit.com, click ‘show private key’ (remember to keep this safe). This is what allows the bot to vote for you
Difficulty
This tutorial is intended to be beginner friendly. (I appreciate feedback on the teaching level).
We’re looking at the command-line, if you’ve installed node and used the command line before it may be a touch too easy for you.
Learning Goals
- familiarise yourself with previous Bots we’ve created in this tutorial series
- Run a bot in the background with Node.js
- Schedule bot task for the future
Step 1 - Setup 💻
In the previous six tutorials, our bots have been running in the browser. Browser bots are a great way to learn but the time has arrived for us to look at using the command-line to stop and start our bots. The command line allows our bot to run in the background on our computer or a remote server. We’re going to use Node.js. Node.js is a platform that allows you to run Javascript (typically a browser-based language) on its own outside of the browser.
Download and install Node.js if you don’t already have it installed. The download page offers installers for major operating systems. Once installed we use Node.js from the command-line. If you’ve never used the command-line it can seem a little daunting but it shouldn’t. the command line is just like using a computer with an app/program except you have to learn the individual commands and type them manually (appose to clicking buttons in an app that do things)
On Mac you’ll open the Terminal app and on Windows you’ll open the Command Prompt program.
You can test Node is install and running correctly by typing node -v
which will output the version you have installed. If you see the output you have node installed and we can continue.
Step 2 - Run A Bot With Node.js 🤖
Download the starter code here this is the code fom tut #6
We’ll start with the Bot code we made in tutorial #6. The bot we made was a HTML file that ran in the browser so the first step is to remove all the html, leaving us with just the javascript. Save the file under a new name bot.js
notice the .js extension for a javascript file.
// Tutorial 07 - Schedule Steem-Js Tasks
const ACCOUNT_NAME = ''
const ACCOUNT_KEY = ''
const INTERVAL = 60 * 60 * 1000
steem.api.setOptions({ url: 'https://api.steemit.com' });
setInterval(claimBalance, INTERVAL)
function claimBalance(){
steem.api.getAccounts([ACCOUNT_NAME], function(err, result){
console.log(err, result)
let sbdReward = result[0].reward_sbd_balance
let steemReward = result[0].reward_steem_balance
let steemPowerInVests = result[0].reward_vesting_balance
steem.broadcast.claimRewardBalance(ACCOUNT_KEY, ACCOUNT_NAME, steemReward, sbdReward, steemPowerInVests, function(err, result) {
console.log(err, result);
});
})
}
You’re code should look like above, make sure to fill in the information for NAME and KEY.
In our previous browser bots, we linked to a remotely hosted version of the Seem-js library. In this bot we’ll want to include it as a package. Node has a package manager built in that lets you download and use external code super easily.
Open Terminal or Command Prompt again.
The cd
command allows you to change directory, we want to change to the directory where we saved our bot.js file. For example I save mine in the documents folder then projects folders then Steem folder. On macOS ~/
represents the users home directory.
cd ~/Documents/Projects/Steem
One we’re in the correct folder we can download the external code by using the command npm install name-of-code
but first we need to initialise a project with npm init
this registers the name and author along with other settings for our project.
Run the command and follow the instructions.
npm init
With our project initialised install Steem through the package manager.
npm install steem
You’ll now have a new file package.json
that contains the project information and a new folder node_modules
that contains any third party code we install.
We need to add a line at the top of our bot.js file to include the steem.js library.
const steem = require('steem’);
Run our bot using Node.js with the node
command and the file we want to run. Try it out!
node bot.js
If you filled in your account name you should see the output of the steem.api.getAccounts()
function.
Step 3 - Using Forever
Aside from not needing the browser window open, we’ve not done much different, infant now we’re just left with the terminal open instead.
Forever is a node package that lets you run Node.js code in the background ‘forever’, if for any reason you code breaks it will restart it. It also means we can close the terminal and leave it running, typically you would use this in a server but it will work on a local computer too.
Install forever through npm. The -g
flag stands for global and allows it’s use anywhere on our system (as apposed to just this project we’re working on)
npm install forever -g
Now run the bot with forever.
forever start bot.js
Just like that the bot running in the background!
Step 4 - Scheduling Bot Tasks
Currently, our bot code runs continuously at the set interval, useful but other types of projects may want a more specific schedule.
Node Schedule is a package is a package set up for time-based scheduled aka running a task every day at 10 am or perhaps every Wednesday at 6pm. depending on what type of bot you’re making this can be super useful.
download & Install
npm install node-schedule
include in the bot code
const schedule = require('node-schedule')
To schedule the bot to run every day at 6 pm we would use this. The second number represents the hour of the day.
schedule.scheduleJob('* 18 * * *', function(){
claimBalance()
});
Node schedule uses the CRON job formatting. See more details here
To test it’s working try scheduling a task a couple minutes into the future and watching what happens with node bot.js
.
Now we’ve looked at scheduling and background tasks it starts to open up the opportunities to make more creative applications with bots.
I hope you find this interesting and useful. If you’re looking into building bots let me know or ask any questions below ✌️
Other Posts in This Series
- Part 1 - Beginner friendly - Build your first Steem bot in Javascript - 30minutes
- Part 2 - Beginner friendly - Building bots With steem-js #2
- PArt 3 - Copy Follow Bot - Building Bots With steem-js #3
- Part 4 - Paid Voting Bot - Building Bots With steem-js #4
- Part 5 - Mention Notifier Bot - Building Bots With steem-js #5
- Part 6 - 🤖 TUTORIAL - Auto Reward Claimer - Building bots With steem-js #6
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Super fast! Thanks for reviewing the tutorial 👊
hey @fuzeh, noticed Utopian 🤖 came around while I was asleep. Looks like it commented but no votes, never had that happen before. Will it vote later or has it bugged?
Looking at steemd it seems I got the comment and no vote, right after me this tutorial got a vote but no comment.
Thank you fot this tutorial.This was what I was looking for...
FD.
No problem, great to hear I can help. Let me know if there is anything extra I can help with.
Hey Sam...
I noticed that my dice bot would crash ever few hours after running it in the browser, so I wanted to set it up using this tutorial.
I removed the HTML and script codes and added:
const steem = require('steem’);
I renamed the html file to bot.js and installed Node.js and the Steem package... so my directory is looking like I think it should...
When I get to the last part of Step 2 in this tutorial, I get this message:
In Atom, the Steem portion of the code and the account key suddenly went red... (the yellow is mine to mask the account details):
Do I have a problem there?
Thanks heaps!
hey dude it looks like you have different types of quote around
'steem’
. Notice the first quote is'
(a vertical straight line) and the second quote is’
(comma moved to the top of the line). Sometimes called smart/dumb qoutes othertimes referenced as quotes vs speech marks. This could easily be copied from my code. I use IA Writer to write my posts and sometimes it autocorrects the quotes/speech mark to the fancier ones, I correct them when I remeber but I could easily have missed this one.Duuuuuuuude!
That was amazing. That was exactly the problem... and I'm pretty sure I would have never figured that out myself... my inexperienced eyes wouldn't have realized the difference.
So... I've now set up my dice rolling bot to fun forever... but I'm super interested in setting it up to run on a server. How would you feel about setting up a tutorial on running bots on a free server? I set up a AWS account, and may have accidently signed up for a Lightsail server, but I haven't had much luck deciphering what to do next to run my script.
Thanks for all your help thus far... I've got quite a few bots I'm excited about setting up, so these tutorials really have been so crazy helpful to me.
haha no worries, it's so easy to miss things especially when you've been staring at the same chunk of code for a while. In general whenever you see a change in the syntax highlighting you don't expect something is wrong, can feel like you're losing your mind if you can't see it though.
Totally keen to do a "run on a server" tutorial. I'm always hesitant to direct people to AWS because it's pretty far from friendly. I'll see if there's a nice way to do it these days and if not I'll see what the best options are. 👍
I'm assuming you need your dice roll bat to run 24/7 ?
Hahahaha, I've definitely had moments where I've stared at code for ages... left it for a bit, and then found my error within a second of opening it again.
Yeah, AWS is really confusing.. I just tried it because it came up as free, but I think it's also trying to get me to pay $5 a month... so yeah, it's a bit odd..... but if there's a simpler low cost 24/7 option then that would be amazing.
I know I'm asking a lot for a tutorial specifically for what I'm interested in, but I hope it'll tie into your bot series nicely for others too. I really do appreciate how amazingly responsive you've been... you're pretty much the best.
test
Awesome tuts
thank you
Hey @sambillingham I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Exactly what I am looking for. Thanks!
Is there a reason you prefer forever over pm2?
no not at all just have more experience using forever. I should use pm2 more and learn about it.
had my fun with all the other tutorials but I am stuck with this one... I have no idea what I am doing wrong ...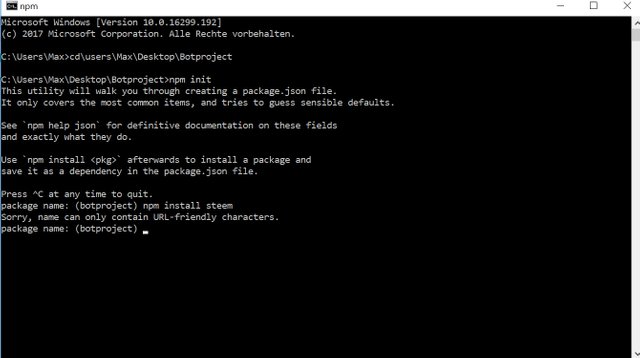