Steem-JS Tutorials #1 - Basics + Validating Wif Key
What Will I Learn?
- validating account wif active/posting key
- Steem-JS basics (voting, commenting, posting, receiving account information)
Requirements
- Node.JS, Download
- Steem Package(how to install at the tutorial)
Difficulty
- Basic
Tutorial Contents
In this tutorial, I learn how to validate a Wif(active/posting) key and how to receive account X information by his username.
Curriculum
The Tutorial
first of all, we need a basic server, the link for my tutorial Here
we need to install steem
package to access the steem blockchain and work with him.
go to your command line and type npm install steem --save
,
this command will install steem package to your project folder.
at the start of the page add this line
const steem = require('steem');
this line will include steem package to the script so we can work with it.
Account Information
let's make a variable with my / other username const user = "lonelywolf";
you don't need to make variable, it's just comfortable for the future.
now let's get my account information.
steem.api.getAccounts([user], function(err, res){console.log(res[0]);}
this is the simple function to get account information,
you need to use [ ]
to get the accounts if you don't use it the function will not work.
you can use it for multiple accounts, just add them.
steem.api.getAccounts([user, "atukh09"], function(err, res){console.log(res[0]);}
result (my account):
[ { id: 495898,
name: 'lonelywolf',
owner: { weight_threshold: 1, account_auths: [], key_auths: [Array] },
active: { weight_threshold: 1, account_auths: [], key_auths: [Array] },
posting:
{ weight_threshold: 1,
account_auths: [Array],
key_auths: [Array] },
memo_key: 'STM8F9tizjUYb5ThY36vLcBiKqUg5wKqVvrfRTjWVX8i1HPXvEPds',
json_metadata: '{"profile":{"name":"TheLonelyWolF","profile_image":"image removed","about":"Youtuber, Steemian, Gamer & developer","cover_image":"image removed","website":"https://steemit.com/@lonelywolf"},"config":{"min_bid_sbd":0.005,"min_bid_steem":0.005,"bid_window":2.4,"comments":false,"refunds":true,"accepts_steem":true,"pre_vote_group_url":"","funding_url":"","api_url":"","max_post_age":"3.5","is_disabled":true}}',
proxy: '',
last_owner_update: '2018-02-07T17:29:18',
last_account_update: '2018-04-04T15:11:54',
created: '2017-12-14T15:13:15',
mined: false,
recovery_account: 'steem',
last_account_recovery: '1970-01-01T00:00:00',
reset_account: 'null',
comment_count: 0,
lifetime_vote_count: 0,
post_count: 445,
can_vote: true,
voting_power: 9314,
last_vote_time: '2018-04-04T18:19:24',
balance: '1.317 STEEM',
savings_balance: '0.000 STEEM',
sbd_balance: '0.003 SBD',
sbd_seconds: '849930375',
sbd_seconds_last_update: '2018-04-04T19:31:18',
sbd_last_interest_payment: '2018-03-21T18:46:27',
savings_sbd_balance: '0.000 SBD',
savings_sbd_seconds: '0',
savings_sbd_seconds_last_update: '1970-01-01T00:00:00',
savings_sbd_last_interest_payment: '1970-01-01T00:00:00',
savings_withdraw_requests: 0,
reward_sbd_balance: '0.000 SBD',
reward_steem_balance: '0.000 STEEM',
reward_vesting_balance: '0.000000 VESTS',
reward_vesting_steem: '0.000 STEEM',
vesting_shares: '266703.545190 VESTS',
delegated_vesting_shares: '245671.180288 VESTS',
received_vesting_shares: '97935.859631 VESTS',
vesting_withdraw_rate: '0.000000 VESTS',
next_vesting_withdrawal: '1969-12-31T23:59:59',
withdrawn: 0,
to_withdraw: 0,
withdraw_routes: 0,
curation_rewards: 1667,
posting_rewards: 164574,
proxied_vsf_votes: [ 0, 0, 0, 0 ],
witnesses_voted_for: 5,
last_post: '2018-04-04T18:14:57',
last_root_post: '2018-04-04T18:14:57',
average_bandwidth: '75124617094',
lifetime_bandwidth: '1196389000000',
last_bandwidth_update: '2018-04-04T19:36:33',
average_market_bandwidth: '76806912525',
lifetime_market_bandwidth: '705900000000',
last_market_bandwidth_update: '2018-04-04T19:36:33',
vesting_balance: '0.000 STEEM',
reputation: '1960197300594',
transfer_history: [],
market_history: [],
post_history: [],
vote_history: [],
other_history: [],
witness_votes:
[ 'arcange',
'imacryptorick',
'lonelywolf',
'steempty',
'utopian-io' ],
tags_usage: [],
guest_bloggers: [] } ]
to get each user at the res
use number, for example, if I want atukh09
user I will use
console.log(res[1]);
and for my account, I will use 0
.
to get specific variables you need to know JSON, so for example I want my posting public key.
console.log(res[0].posting.key_auths[0][0]);
easy as that.
Voting
first, we need to set some variables.
you need to set those:
wif
= posting key,
voter
= voter username,
author
= voted username,
permlink
= the permlink of the post, for example, this is the original URL - https://steemit.com/steem/@lonelywolf/steem-js-steem-node-js-what-i-m-doing-and-what-i-want-to-do
we need this - steem-js-steem-node-js-what-i-m-doing-and-what-i-want-to-do
,
weight
= the voting weight, weight works as integer so 10.00% = 1000, 100.00% = 10000 etc.
we need all of those variables for the voting function, when we're done we should get something like that.
const wif = "XXXXXXXXXXXXXXXXXXXXXXXXX", voter = "lonelywolf", author = "lonelywolf", permlink = "steem-js-steem-node-js-what-i-m-doing-and-what-i-want-to-do", weight = 1000;
now let's broadcast the vote,
steem.broadcast.vote(wif, voter, author, permlink, weight, function(err, result) { console.log(err, result); });
this command takes all of the variables and broadcast them to the blockchain and get send the upvote.
if you got an error it can cause because of a low voting weight -
just change to at least 10% vote, 1000 at an integer.
if you have done all correctly it should work fine!
and you will get this -
congrats! you made a vote through steem-js.
Posting
make a few variables for the post,
wif
= posting key,
maintag
= the first(main) tag of the post,
user
= the post author,
permlink
= the post url, example: macro-entri-28
,
title
= the post title,
body
= the post body(content).
now let's broadcast the post,
steem.broadcast.comment(wif, '' /* Leave parent author empty*/, maintag, user, permlink, title, body, { tags: ['test'], app: 'steemjs/examples' }, function(err, result) { console.log(err, result); });
note
: at the tags, you do not make a variable because it's a JSON, so put your tags at tags:['test']
replace test with the tags, example -
tags:['photography','tutorial']
and if all correctly, you will see a new post on the account!
Comment
it's similar to post just with few more variables.
add this variables -
parentAuthor
= the account who created the post you comment on
parentPermlink
= the permlink of the post you commenting on
and use the same code with the variables
steem.broadcast.comment(wif, parentAuthor, parentPermlink, maintag, user, permlink, title, body, { tags: ['test'], app: 'steemjs/examples' }, function(err, result) { console.log(err, result); });
that's all! really simple.
Validating Wif Key
make 2 variables,
one of the wif key and second for the username.
we need those to get the public key and validate the private key.
wif
= active private key, you can find it at Wallet > Permissions
at your steemit wallet
user
= your username
first, we need the user public key.
steem.api.getAccounts([user], function(err, res){ const pubWif = res[0].active.key_auths[0][0]; });
note
: you can use it for posting key as well just change active
to posting
.
first, we want to check if the "wif" is valid.
if(steem.auth.isWif(wif)){}
this will check if the wif we entered is real steem wif key.
now we want to validate the private key with the public key.
const Valid = steem.auth.wifIsValid(wif, pubWif);
the function will give a boolean (false, true), so we store it into a variable.
now if it's true to do "X" if it's false do "Y"
if(Valid){ console.log("true"); }else{ console.log("false"); }
and now you're done, you can do anything you want, vote with it, comment, post, anything you just want.
this is all for this tutorial, wait for the next one and learn more about Steem-JS!
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @portugalcoin, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
Thank you!
You got a 28.62% upvote from @slimwhale courtesy of @lonelywolf!
Great post! You've earned a 13.17% upvote from @dolphinbot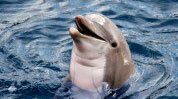
@lonelywolf thought you deserved 4.64% of our upvote for this post.
I'm here to tell you congratulations and to let everyone know there's a livestreaming service available for Steem. You can go live and start earning rewards via the Steem blockchain today at dlive.io!
Hey @lonelywolf I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x