Demonstrate Simple Pendulum with Android Studio
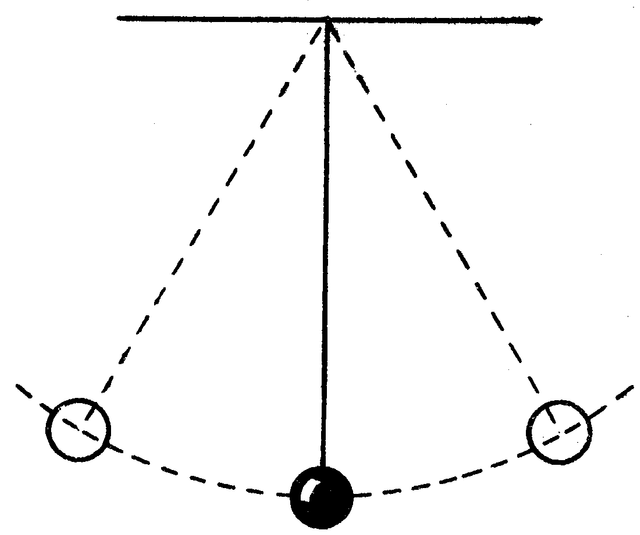
Repository
https://github.com/enyason/Simple_Pendulum_Demonstration
What Will I Learn?
- How to Implement simple pendulum with android studio
- How to create custom views
- How to do simple animation with the canvas class
Requirements
- System Requirements : Java JDK, Android Studio
- OS Support for Java : Windows, mac OS, Linux
- Required Knowledge : A fair knowledge of Java and use of Android Studio
Resources for this Tutorial
Canvas Android Documentation : https://developer.android.com/reference/android/graphics/Canvas
Java Docs : http://www.oracle.com/technetwork/java/javase/documentation/api-jsp-136079.html
Simple Pendulum : https://en.wikipedia.org/wiki/Pendulum
Difficulty
- Basic
Tutorial Duration 20- 30 Minutes
Tutorial Content
In this tutorial, we are going to demonstrate the simple pendulum using android studio. The gif below demonstrate a typical simple pendulum.
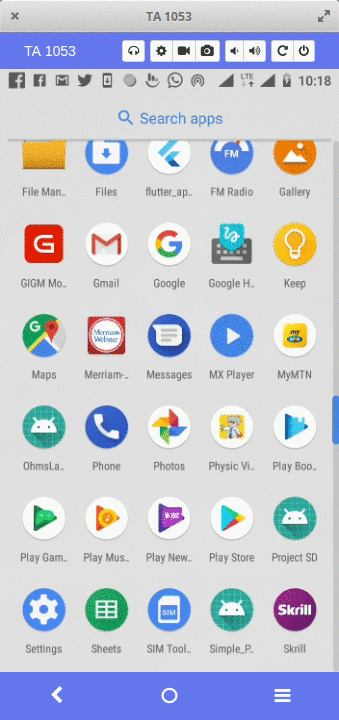
What is a Pendulum?
A pendulum is a weight suspended from a pivot so that it can swing freely. For more information on simple pendulum, visit https://en.wikipedia.org/wiki/Pendulum
STEP 1 : Create a new Android Studio Project
Open android studio an create a new project and add an empty activity to it
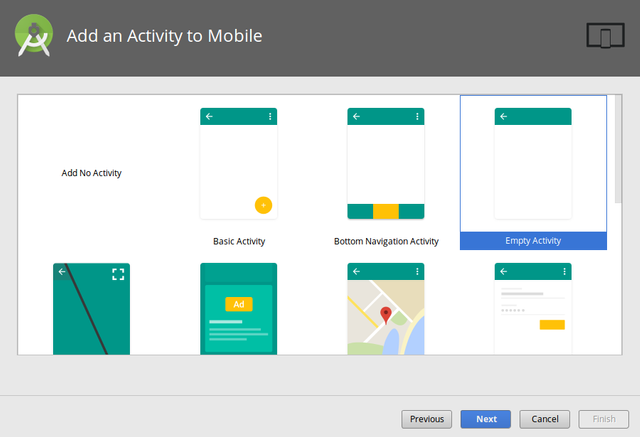
STEP 2 : Create a Custom View for the Pendulum Implementation
To achieve this , we are going to extend the view class of the android framework
- Extend View Class
Here we extend the view class then we implement it's constructor. See image below
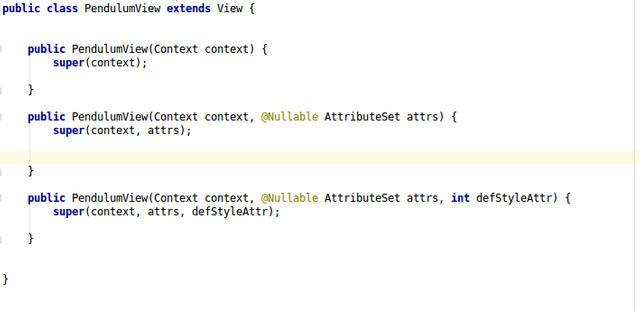
public class PendulumView extends View {
public PendulumView(Context context) {
super(context);
init();
}
public PendulumView(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
init();
}
public PendulumView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
}
STEP 3 : Override the onDraw Method of the class
All our implemtation will go to the onDraw method
Implement the rectangular box that serves as the holder
... pathHolder.moveTo(xCenter,100); pathHolder.lineTo(300,100); pathHolder.moveTo(300,100); pathHolder.lineTo(300,50); pathHolder.moveTo(300,50); pathHolder.lineTo(420,50); pathHolder.moveTo(420,50); pathHolder.lineTo(420,100); pathHolder.moveTo(420,100); pathHolder.lineTo(xCenter,100); ...
This codes blocks basically draws a rectangular path based on the x and y points we specified. The result is seen in the image below
Implement the Thread
... //build path for the thread pathThread.moveTo(xCenter, 100); pathThread.lineTo((float) thread_x, 350); ...
This code block draws the line for the thread, and its length is updated on motion.
Implement the circle ball
canvas.drawCircle(circle_x, 370, 30, paintCircle);
This line on code draws the circle to screen and updates its position, causing the ball to move when
invalidate()
is called on the ondraw method. see image below
STEP 4 : Handle Ball Direction
if (circle_x >= boundryRight) { x_dir -= 5;}
if (circle_x <= boundryLeft) { x_dir += 5;}
circle_x = circle_x + x_dir;
thread_x = thread_x + x_dir;
In this step, we handle the ball direction by checking comparing its position with the specified boundary
STEP 5 : Include PendulumView as a view in the activity_main_layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.nexdev.enyason.simple_pendulum_demonstration.MainActivity">
<com.nexdev.enyason.simple_pendulum_demonstration.PendulumView
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</RelativeLayout>
Code explanation
All we did here is to include the PendulumView as a view in the layout file. Below is the output of what we have done in this step
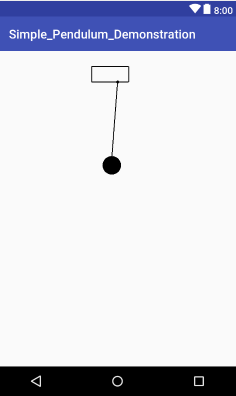
With all these steps taken, we should have something like this
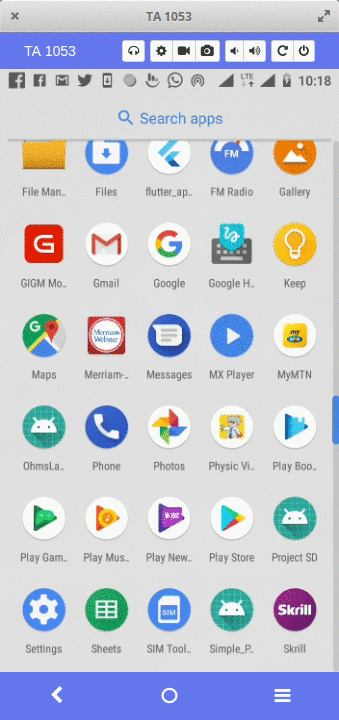
Curriculum
Proof of Work The complete source code can be found on github
Thank you for your contribution @ideba.
After analyzing your contribution we suggest the following:
In title it's important to have key words about the technology that will explain. We suggest you place this keyword in your Java JDK tutorial.
Code sections are better displayed using the code markup, instead of placing them as screenshots.
Good tutorial to learn how to create objects and give movement to objects in Java JDK / Android Studio.
We are waiting for more of your tutorials. Thank you again for your work!
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 4 contributions. Keep up the good work!
thanks for your review at @portugalcoin . I'll improve on my contribution
Hey, @ideba!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hi @ideba!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server