Advance Ruby Development Episode-1
Welcome to the First Tutorial of Advance Ruby Development
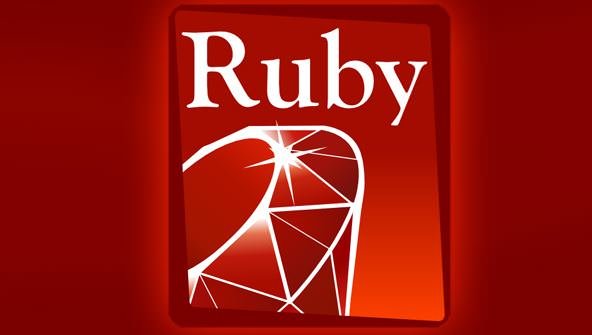
What Will I Learn?
- You will learn how to develop Applications using Ruby
- You will learn about IRB shell in Ruby programming
- You will learn about various class methods and their usage
- Your will learn about reading and writing through class methods
Requirements
System Requirements:
- Ruby Installer for building apps
- SciTE for code editing
- Command Prompt or Terminal
OS Support:
- Windows 7/8/10
- macOS
- Linux
Difficulty
- Intermediate
Required Understanding
- You just need a little bit of knowledge basic Ruby Syntax
- A fair understanding of Programming
- A thirst for learning and developing something new
Description
- In this Advance Ruby Development course, I will teach you the advanced concepts of the Ruby programming language. Ruby was designed to be more powerful that Perl, and more object-oriented than Python, and has gained in popularity due to its power and ease of use. By the end of this course you will surely develop some powerful and concrete applications. Also in the end of this course we will be developing a Restaurant finder app.
What is Ruby?
Ruby is an object-oriented, general purpose programming language designed by Yukihiro Matsumoto in the 1990s. Intended for improved productivity and entertaining, it creates a great starter language for first time programmers because of its simplicity, readability and concentrate on fun. Commonly used with Rails applications, that is a very popular language that is always in popularity. Organizations such as Twitter and Kickstarter utilize Ruby.
With Ruby, almost everything is an object. Every bit of information as well as codes can be provided their very own attributes and actions. Object-oriented programming calls attributes by the title instance variables and also actions tend to be known as methods. Ruby’s genuine object-oriented approach is quite commonly demonstrated with a bit of code that is applicable an action to a number.
7.times { print "I *like* Open Source Projects" }
As an example, addition is conducted with the plus (+)
operator. However, if you would instead use the readable word plus
, you can add this type of method to Ruby’s builtin Numeric class.
class Numeric
def plus(x)
self.+(x)
end
end
y = 4.plus 3
which results in the value of y=7
What is Interactive Ruby Shell (IRB) ?
Interactive Ruby Shell (IRB ) is actually a REPL with regard to coding in the Ruby Language. Typically the abbreviation irb originates from the point that the filename extension for Ruby is .rb, even though interactive Ruby files don’t have an extension involving .irb.
The program will be launched from the command line and enables the execution of Ruby commands with immediate response, testing in real-time. This features command record, line modifying capabilities, and job control, and it is capable of communicate directly being a shell script on the internet and also connect to a live server.
irb [ options ] [ programfile ] [ argument... ]
Example:
irb(main):001:0> num = 4
=> 4
irb(main):002:0> def fact(num)
irb(main):003:1> if num <= 1
irb(main):004:2> 1
irb(main):005:2> else
irb(main):006:2* num * fact(num - 1)
irb(main):007:2> end
irb(main):008:1> end
=> :fact
irb(main):009:0> fact(num)
=> 24
Methods In Ruby Programming
Ruby uses methods to use a block of codes. In other programming languages some other techniques are used like functions and procedures. Now we will have al look at methods in ruby and then we proceed to the next level.
Let have a look at this example
def course
puts "This course is about Ruby Programming "
end
def sub
puts 7-3
end
# Underscore can be used between words, like variable names in this case
def longest_word
words = ['pineapple', 'orange', 'mango', 'watermelon']
longest_word = words.inject do |memo,word|
memo.length > word.length ? memo : word
end
puts longest_word
end
# Useful for tests and Booleans values
def greater_seven?
value = 7
puts value > 7 ? 'More than 7' : 'Less than 7'
end
In the above example I define four methods which are course,sub,longest_word and greater_seven
Behaviour:
course
: It simply displays a message on the screen which is in this case is “This course is about Ruby Programming ”
sub
:This method simply perform the subtraction of two number which is passed as arguments which are 7 and 3
longest_word
: This method does a little interesting job here,it returns me the longest word out of an array of four words by using the inject
function which accumulates and returns the longest length word by increment the memo each time.
greater_seven?
: This methods basically testing which number is greater between the two and returns the Boolean value. It uses ?
at its end
Reading and Writing Operations:
Ruby has methods for Reading and Writing known as Reader and Writer. Other languages have getters and setter for this reading/writing in programs. These methods will allow us to read data from a program or write data to it. Later these operations will help us in making our restaurant finder app.
Example:
class Person
def set_noise(noise)
@noise = noise
end
def make_noise
@noise
end
end
person_one = Person.new
person_one.set_noise("Laugh!")
puts person_one.make_noise
person_two = Person.new
person_two.set_noise("Groan!")
puts person_two.make_noise
Now this is a very common feature of object-oriented programming languages, sometimes in other languages, they're called getter and setter methods. Either one is fine. You can use either name, typically in Ruby, we're going to call them reader and writer methods. So, here is the code which is a perfect example to explain, what we're looking for is a pairing of something that will set a value for us and something that will get a value for us, and that's exactly what we already have with our two methods
The first one is a setter method or a writer method. So we're setting the value of noise equal to a value, and this is the classic format for what a setter method would look like.
set_noise
equal to a value. It sets it for us. Then we have a get_noise
. It's a getter method. The idea is that we are getting that value back. One of these methods sets it, one of them gets it.
Now the important thing about these two methods is that they give us access control over these instance variables. So for example:
class Person
def set_noise(noise)
@noise = noise
end
person_one = Person.new
person_one.set_noise("Laugh!")
puts person_one.make_noise
person_two = Person.new
person_two.set_noise("Groan!")
puts person_two.make_noise
if we were to take away get_noise
, now we have no way to get that value back. Of course, we could write another method that would do it for us, but our getter method is gone
class Person
def make_noise
@noise
end
end
person_one = Person.new
person_one.set_noise("Laugh!")
puts person_one.make_noise
person_two = Person.new
person_two.set_noise("Groan!")
puts person_two.make_noise
Same thing here, if we were to take away set_noise
, now we have no way to set it by calling a method. we need a setter method if we want to be able to do it directly.
Like set_noise
and get_noise
and using underscore writing approach is very common in a lot of programming languages. In Ruby though, we can make use of that syntactic sugar that we looked at earlier, and so we can take get _out and simply ask for noise. So it asks Person to do the method noise, which returns the instance variable, noise. It's a very commonsense approach.
class Person
def noise=(noise)
@noise = noise
end
def noise
@noise
end
end
person_one = Person.new
person_one.noise = "Laugh!"
puts person_one.noise
person_two = Person.new
person_two.noise = "Groan!"
puts person_two.noise
So nowperson_one.noise
and person_two.noise
just returns that to us, where the syntactic sugar comes in is that we can do the same thing with set, we can take away set and just say noise=(noise).
Summary
In this tutorial of Advance Ruby Development we learn about the methods, also now we have a better understanding of reading and writing using methods in Ruby. In the next tutorial we will learn about modules and development of our Restaurant Finder App.
Your contribution cannot be approved because it does not follow the Utopian Rules.
Need help? Write a ticket on https://support.utopian.io.
Chat with us on Discord.
[utopian-moderator]
Congratulations @exploringworld! You received a personal award!
Click here to view your Board
Congratulations @exploringworld! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Vote for @Steemitboard as a witness to get one more award and increased upvotes!
Amazing post! I love it. Hey UPVOTE my post: https://steemit.com/life/@cryptopaparazzi/chapter-one-let-there-be-the-man-and-there-was-a-man-let-there-be-a-woman-and-there-was-sex and FOLLOW ME and I ll do the same :)