[Tutorial] Bootstrap 4 From Scratch with @cryptogecko #3 - Bootstrap Starter Pack Using Node.js
Repository
https://github.com/twbs/bootstrap.git
What Will I Learn?
- You will learn to set up the starter pack to help you build bootstrap themes.
- You will learn how to write gulp code to automate simple development tasks.
Requirements
- Desktop/PC
- Node.js
- A text-editor
- Git Bash if you're on windows, or terminal if you're on Linux or Mac
Difficulty
Basic - We will be covering every aspect of developing elegant and beautiful bootstrap themes from scratch, so you don't need to have any experience.
Tutorial Contents
You can read the introduction of this series here: Bootstrap 4 From Scratch: Introduction. We will be building 5 projects using this starter pack in the future tutorials.
If you do not know what bootstrap is, you can read more about it here: Bootstrap 4 From Scratch: Bootstrap
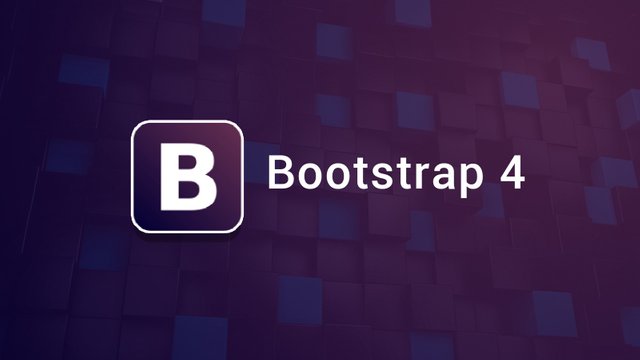
Glossary
Git - Git is a version-control system for tracking changes in computer files and coordinating work on those files among multiple people. It is primarily used for source-code management in software development, but it can be used to keep track of changes in any set of files.
Gulp - Gulp is a toolkit for automating painful or time-consuming tasks in your development workflow, so you can stop messing around and build something.
Node.js - Node.js is an open-source, cross-platform JavaScript run-time environment built on Chrome's V8 JavaScript engine that executes JavaScript code outside of a browser.
Sass - Sass (Syntactically awesome style sheets) is a style sheet language
Let us first set up our initial environment for bootstrap development. To have a more professional workflow throughout this tutorial series we will be using SASS which is a CSS pre-processor, we will be working with GULP to compile the SASS. So, in order to do this, we will be installing Node.js.
Node.js comes with NPM (Node Package Manager), and it is used to install all the required packages to run our website, including bootstrap.
I have also installed the Visual Studio Code text editor on my desktop. Now, I would suggest using it. But, it is fine if you use any other editor.
I am also be using GIT BASH, which is a better command line for windows. It is available for Linux an Mac as well, but the terminal provided for these operating systems should be just fine, you do not need really need to install GIT.
This entire tutorial series is going to be cross-platform, which means you don't need to have a particular operating system to follow along.
All right so let's get started, we will build our bootstrap 4 starter pack that we will be using throughout this tutorial series.
Before we start building our starter pack we have to create a new folder for our pack. So let us first create a folder anywhere on our PC. You can name it anything you like, I will be naming it bs4starterpack. After the folder has been created, you need to open the folder and right click anywhere to access the options, and then you have to open GIT BASH in this folder. Linux and Mac users can use the terminal to follow along, it will work just fine.
The first thing we are going to do is to generate a package.json file, which is like a manifest file, and it is going to hold all the dependencies. We can generate the package.json file using "npm init" command in the terminal. To use the npm command you should have node.js installed on your PC.
This command will ask you a couple questions, if you do not want to see them, you can just type npm init -yes. The yes flag forces the command to generate the package.json file with default values.
Now, we have to install the dependencies for our project, let's type
npm install bootstrap font-awesome jquery popper.js --save
and run it by pressing the enter button. These are the dependencies we will be needing in our project. The save flag saves the data about our dependencies in the package.json file.
Now, let's install our development dependencies, which include gulp, gulp-sass, which is a plugin to compile our SASS, and then browser-sync, which will allow us to have a development server that autoloads. So for that, we are going to type
npm install gulp gulp-sass browser-sync --save-dev
because these are our dev dependencies and only needed for the development purpose. So, these dependencies will also be added to our package.json file but as dev dependencies.
After you have installed all of the requires dependencies, your file should look something like this, accept few things:
{
"name": "bs4starterpack",
"version": "1.0.0",
"description": "Bootstrap Starter Pack to create elegant and beautiful themes",
"main": "index.js",
"scripts": {
"start": "gulp"
},
"repository": {
"type": "git",
"url": "git+https://github.com/ravigohel/bs4starterpack.git"
},
"keywords": [
"bootstrap",
"gulp",
"sass",
"themes"
],
"author": "example@gmail.com",
"license": "MIT",
"bugs": {
"url": "https://github.com/ravigohel/bs4starterpack/issues"
},
"homepage": "https://github.com/ravigohel/bs4starterpack#readme",
"dependencies": {
"bootstrap": "^4.1.3",
"font-awesome": "^4.7.0",
"jquery": "^3.3.1",
"popper.js": "^1.14.4"
},
"devDependencies": {
"browser-sync": "^2.26.3",
"gulp": "^3.9.1",
"gulp-sass": "^4.0.2"
}
}
Now, the last thing we are going to install is the gulp command line interface, and we want to install it globally so that we can access it from anywhere. So we will type "npm install -g gulp-cli", the g flag tells the npm to install it globally.
So these are the dependencies we need for our projects. So what we need to do now is to create a new source folder in our work folder. We will name it "src", and this is where all of our stuff will go, for example, our HTML files, our CSS and sass files. Inside the src folder create a new folder and name it "SCSS", and this is where our sass files will go. SASS is just a CSS pre-compiler that allows us to use things like variables in our CSS, we can nest styles, and the way we are building this, it's going to take our SASS file and just compile it automatically to a regular CSS file to include it in our HTML.
So let us now start working on the gulp file. As I said earlier that gulp is a task runner, and it can be used to do literally hundreds or maybe thousands of different things. We are going to use it for us to compile SASS, we are going to use it to move certain files from the Node Modules folder, like our Bootstrap, CSS files to our source folder. We are also going to use it to run our development server with browser-sync.
So, let's create a new file in our work folder, and name it gulpfile.js, and copy the below code in this file. Don't worry the code is very easy to understand, as the comments written above the code is self-explanatory.
const gulp = require('gulp');
const browserSync = require('browser-sync').create();
const sass = require('gulp-sass');
// Compile SASS & Inject Into Browser
gulp.task('sass', function(){
return gulp.src(['node_modules/bootstrap/scss/bootstrap.scss', 'src/scss/*.scss'])
.pipe(sass())
.pipe(gulp.dest("src/css"))
.pipe(browserSync.stream());
});
// Move JS Files to src/js
gulp.task('js', function(){
return gulp.src(['node_modules/bootstrap/dist/js/bootstrap.min.js', 'node_modules/jquery/dist/jquery.min.js', 'node_modules/popper.js/dist/umd/popper.min.js'])
.pipe(gulp.dest("src/js"))
.pipe(browserSync.stream());
});
// Watch SASS & Server
gulp.task('serve', ['sass'], function(){
browserSync.init({
server: "./src"
});
gulp.watch(['node_modules/bootstrap/scss/bootstrap.scss', 'src/scss/*.scss'], ['sass']);
gulp.watch("src/*.html").on('change', browserSync.reload);
});
// Move Fonts Folder to src/fonts
gulp.task('fonts', function(){
return gulp.src('node_modules/font-awesome/fonts/*')
.pipe(gulp.dest("src/fonts"));
});
// Move Fonts Awesome CSS to src/css
gulp.task('fa', function(){
return gulp.src('node_modules/font-awesome/css/font-awesome.min.css')
.pipe(gulp.dest("src/css"));
});
//Run all the tasks
gulp.task('default', ['js', 'serve', 'fa', 'fonts']);
In the first three lines of code, we are just bringing in the dependencies we installed earlier, because we will be using them here to automate some tasks.
// Compile SASS & Inject Into Browser
gulp.task('sass', function(){
return gulp.src(['node_modules/bootstrap/scss/bootstrap.scss', 'src/scss/*.scss'])
.pipe(sass())
.pipe(gulp.dest("src/css"))
.pipe(browserSync.stream());
});
The first task is compiling the sass files and injecting them into the browser. The way we define the gulp task is to simply use gulp.task command then we name it and then we are using a function that runs. So in the function, we are returning a telling it the source of all the sass files we want it to compile. The pipe plugin compiles the sass files into regular css files and moves the compiled css files to the destination we provided, in this case "src/css". And, the browserSync pluging synchronizes any change made to the sass file, and shows them into our browser.
The same thins is done with the javascript files, we are first telling the gulp task to bring the files and move them to a specific location. We are bringing the jquery, popper and bootstrap javascript files to our "src/js" folder.
Next we are watching any changes made to the sass files or the html files in our src folder. If any change is made to these files, the browser will reload, and then we can see the changes made in the browser.
Next two tasks are bringing in the fonts files to our "src/fonts" folder, and font awesome css files are brought into "src/css" folder.
The last task is calling all the tasks at once and running it. So that when we run gulp, it will run all the default tasks we need. We are passing a parameter of an array of the different tasks we want to run. If you've followed along, you should have installed gulp-cli globally, and now we should be able to run "gulp" in our command line. What it does is, it will go through all the tasks and it will complete them using the instructions given in the ''gulpfile.js" and it will open a development server on port 3000 in our browser.
Now, create an index.html file in the "src" folder, this is where we will be importing the css and js files, and this file will become our homepage in our future projects.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Bootstrap 4 Starter Pack</title>
<link rel="stylesheet" href="css/font-awesome.min.css">
<link rel="stylesheet" href="css/bootstrap.css">
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1>Bootstrap 4 Starter Pack</h1>
<p>Lorem ipsum dolor, sit amet consectetur adipisicing elit. Dignissimos, tempora ipsa! Eveniet fuga perspiciatis rem hic cumque asperiores autem inventore nobis! Reiciendis, rerum praesentium temporibus totam dolore magni tempore qui.</p>
<script src="js/jquery.min.js"></script>
<script src="js/popper.min.js"></script>
<script src="js/bootstrap.min.js"></script>
</body>
</html>
Now, let's run gulp command in our terminal, and you will see that the gulpfile has created a css, js and fonts folder for us to work with.
Now, I just want to prepare this starter pack for our first project. What we're going to do is go to our bs4starterpack and you should get rid of the node_modules folder with the package-lock.json file. You should also remove the js, css, and fonts folders from the src folder, beacuse when we run "npm install" command, these filse will be brought into these folders again. So, you don't need to worry about them. Now, you can save this folder somewhere on your desktop.
Thank you for your contribution. Below is our feedback:
npm install gulp gulp-sass browser-sync --save-dev
Looking forward to future more innovative tutorials by you.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
hello @mcfarhat,
I have made the changes you suggested here. I will make sure that the future tutorials are much more innovative and easy to understand. Thank you so much for reviewing this. :)
I wanted to make one thing clear that, this is an open source project. Where I am trying to create a bunch of templates using the bootstrap that can be used by our community. Cheers!
This comment was made from https://merasteem.com
Thank you for your review, @mcfarhat!
So far this week you've reviewed 1 contributions. Keep up the good work!
!sbi status
Hi @cryptogecko!
Did you know Steem Basic Income has a Quality Policy?
!bookkeeping magicdice
!bookkeeping magicdice
Hi @cryptogecko!
magicdice
Received:
Spent:
Total:
!sbi status
!bookkeeping drugwars
Hi @cryptogecko!
drugwars
Received:
Spent:
Total:
First transfer was before 4.18 days.
!bookkeeping magicdice
Hi @cryptogecko!
magicdice
Received:
Spent:
Total:
Hi @cryptogecko!
Did you know Steem Basic Income has a Quality Policy?
!sbi status
Hi @cryptogecko!
Structure of your total SBI vote value:
To reduce blockchain clutter, you can also check your status in our Discord server!
https://discord.gg/VpghTRz
!sbi status
Hi @felipejoys!
Structure of your total SBI vote value:
To reduce blockchain clutter, you can also check your status in our Discord server!
https://discord.gg/VpghTRz
!sbi status
Hi @cryptogecko!
Structure of your total SBI vote value:
Did you know that your SBI level shows in your Steempeak wallet?
Hi @cryptogecko!
magicdice
Received:
Spent:
Total:
Hi @cryptogecko!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server