[Unity Game Development Tutorial: 6] - Coding Lesson 2 - Some of those things you learn over time
There are some techniques and things that are useful to know when coding in Unity. This lesson will focus on those specific instances.
If you like this tutorial and would like to see more of them please vote. Also, if you have requests on things you'd like me to delve into please let me know.
Previous tutorials that had any coding elements:
[Unity Game Development Tutorial: 2] - The basics - An Intro to Unity - Layout, Non-Coder, Coder
[Unity Game Development Tutorial: 4] - Coding Education Speed Boost - Coding Lesson 1 - also my naming conventions
This lesson assumes you are familiar with C# and that the previous tutorials above made sense to you.
Relationship between objects, child, parent
In Unity in the hierarchial view everything is a GameObject even if it is empty. One thing all Game Objects have is a Transform. The Transform contains the position, rotation, and scale of an object.
When you nest objects in the asset view you can keep adding objects below other objects. When there is an object above another object in the hierarchial view that is considered a parent. Here are some examples:
In that view I named the object RootLevel so you would see this object is at the root level in the scene. It has no parent. It does have an object named SecondLevel below it. SecondLevel is considered a Child of RootLevel. RootLevel is considered the Parent of SecondLevel. This can be continued as this example shows:
It is important to know how to find children, and how to instantiate something and make it a child or parent of another object. I will cover that here.
How does an object define a parent
Parents are actually referenced by their Transform. When you are finding objects the Find routine returns a transform.
Every object has a place to store the Transform of their parent as gameObject.transform.parent.
This means if you instantiate an object and then set object.transform.parent=ObjectToTreatAsParent.transform you will turn the object into a child of ObjectToTreatAsParent.
Here is some example code:
Important thing about children
Once an object has a parent the values stored in the transform of that object are in relationship to the parent. So if the locations are 0,0,0 for position and 0,0,0 for rotation then it will be at where the parent is considered to be and with no additional rotation. You can tweak these values. A sword in the hand of a character could be positioned so it corresponds to the hand location, and the rotation turned so it looks good.
How to find children
So how can a parent find the children connected to it.
I will first show you how to find a specific child by it's name. You do this by using the FindChild() method off of the transform of the parent. FindChild() will return a transform. Many tutorials recommend you use:
GameObject oObjectFound=oParent.transform.FindChild("CoolWeapon").gameObject;
This would in theory set oObjectFound to be the item called CoolWeapon that is a child of oParent.
What happens when there is no CoolWeapon? It is not happy at all and will throw an error. I have a slightly different method that has some error checking in it that I would like to share with you here:
In this technique we use FindChild and capture the transform. We can then test for the transform being NULL meaning it didn't find anything before trying to grab the gameObject from that transform.
The popular method without this error checking blows up because it assumes the object will be found. When it is not found for some reason you end up trying to get Unity to give you a GameObject attached to a null transform and it does not like it. This was something I discovered by trial and error and I've seen most find child examples not test for this condition.
How to find all children
There are quite a few ways documented around the internet for finding child objects. It seems the most efficient is to use the built in features of the transform itself. I am going to write a little method here that will return an array of child objects in the form of an array of GameObjects. This could be done a number of different ways such as building a list and then using the .ToArray() method on the list. That is a common method I myself use, but in this case I am going to do it without a list. This means I need to do it in two passes. I'm going to count the number of transforms for children, initialize an array, and then copy GameObjects into the array.
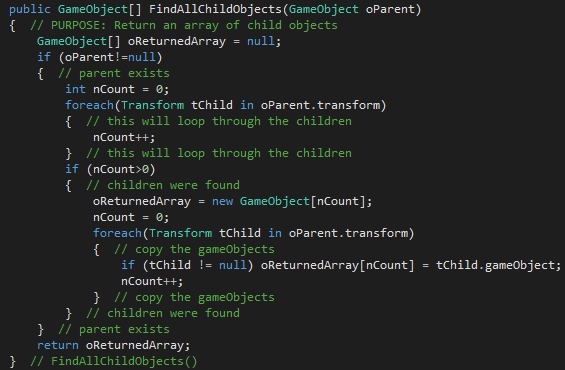
Setting the textures on a material
What if you want to set the textures on a material at runtime? Rather than having a material for every texture, could you not simply change the texture itself?
Yes!
An important consideration with this is it will change the texture for ALL objects that share the same material. You can create a new instance of the material though and assign a texture and it would not impact all other objects. It would for all intents and purposes count as another material though and is another batch or draw call.
I am going to make a simple method here to show off setting values of textures. We will assume that the shader being used is the new Unity Standard Shader in this example. The shader being used will determine the name you have to use when setting the texture.
The method above is setup to support either the Standard or the Standard (Specular Setup) shader depending upon which boolean value is chosen.
You can tell the properties of a shader that can be accessed via code by clicking the little menu in upper right when you are on a material and choosing edit shader. Here are all of the properties for those two shaders.
Standard Shader
Standard Shader (Specular Setup)
Order of Operation - Event Life Cycle
Knowing what order various events occur is important. Here is a flow chart that explains that as pulled from the docs.unity3d.org site.
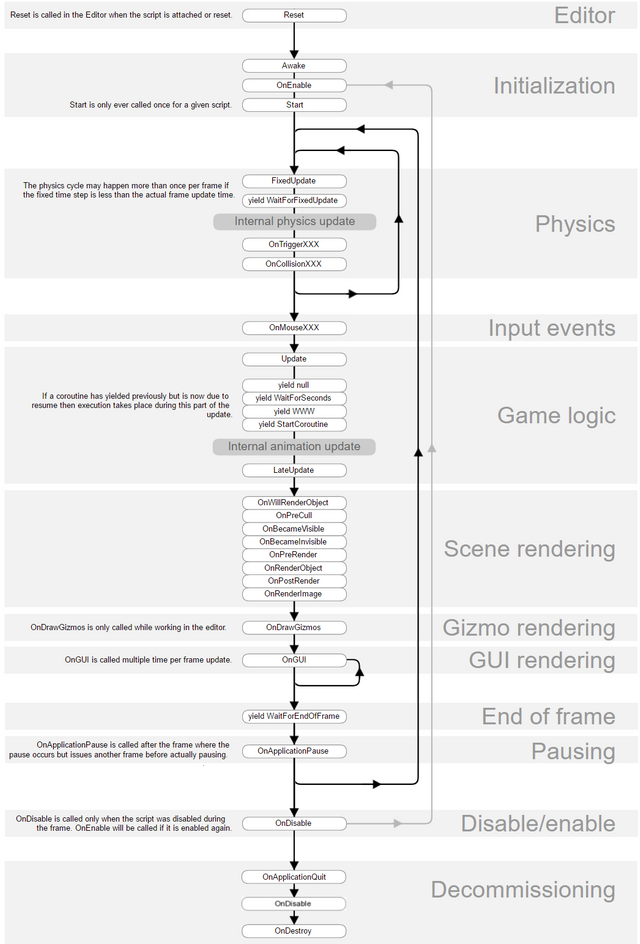
Conclusion
That is some information that I myself have ended up searching for on more than one occasion as I forgot about it. now it is in one location and hopefully it is useful to those of you here.
As with all my tutorials please up vote this post if you find it valuable. If you have anything specific you would like me to make a tutorial about please ask.
Thank you for your time... Happy developing, and happy steeming.
Great tutorials! I also love making stuff with Unity!
Thanks for all your hard work and thanks for putting all of this together!
I will be referring to your unity post for sometime (:
You are welcome. I'll keep making them as long as people vote on them. With a limit of 4 blog posts per day I tend to focus on things people indicate they want to see. If you have anything specific you want me to go over I will if you ask, otherwise I'll just keep basically doing brain dumps as I think of them.
I get games into the hall of fame in Game Dev Studio all the time. I am pretty sure I am the best game developer alive although I have no idea where to start. Luckily for me I found these guides and I can finally put all those complicated Japanese named guys to shame.
Go get em. ;)