Let's Learn How to Create a Discord Bot
If you are in the Discord App you might have seen a lot of Bot around whether its Banjo, Tatsumaki etc. You might be wondering how these bots got there, the answer is to write an application and run it on your local server or a public server to make it active. In this post, we will learn how to create a discord bot using Node.js.
Little about Node.js
If you are not familiar with Node.js, then its very easy to get started with Node.js as there are so many articles out there on web to get you started. One such article I have written on my blog Getting Started with Node.js. Few paragraphs about Node.js taken from the post.
Node.js uses v8 javascript engine which is the same engine used by Chrome browser. It is event driven and has non-blocking standard libraries which means if you are doing any I/O bound operations, it will happen asynchronously. Node.js is not a framework neither it is a programming language, it is just a run time environment for developing server-side web applications. You can use Node.js for creating I/O bound applications, data streaming applications, single page applications etc, but it is not advisable to use Node.js for CPU intensive applications.
When we are discussing Node.js Basics then you should be familiar about NPM. NPM is a node package manager which handles and resolves dependencies. It makes easy for JavaScript developers to share and re-use code and it makes it easy to update the code that you're sharing. It is been called as an online repository for Node.js packages/modules. To install modules using npm you need to run below command npm install package_name.
Node.js is very easy to install. Installers can be downloaded from Node.js Download Page. In order to structure your program into different files, Node.js provides you with the simple module system. To use this module system you need to use require() which is used to imports the contents from another JavaScript file.
To get started we need to install Node.js from Nodejs.org. Once installed you can check the version of Node.js installed using command node -v in the Node.js Command Prompt.
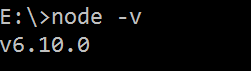
Create new Discord App
Now its time to create a new Discord App. At first you need to visit Discord App Developer URL and create a new App. I have given my bot name as iamabot.
You can add the app description later, and no need to add the redirect now.
Next, since the bot will be operated as a user, you need to create it by clicking on Create a Bot User.
Once you do that you will get a User ID and a Token ID (Keep the Token ID Safe) and click on Public Bot (can be added on any server).
Our Bot has been created, now it's time to write some code in Node.js.
Node.js Application
At first, you need to create a package.json file. The content of the Package.json is shown below. We will be using discord.js node.js module to interact with Discord App.
{
"name": "discordapp",
"version": "1.0.0",
"description": "A discord Bot",
"main": "index.js",
"scripts": {
"start": "node index.js",
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"discordie": "^0.11.0"
}
}
The index.js file content is
var Discordie = require('discordie');
var request = require('request');
var Events = Discordie.Events;
var client = new Discordie();
client.connect({
token: 'Your Bot Token'
})
client.Dispatcher.on(Events.GATEWAY_READY, e => {
console.log('Connected as: '+ client.User.username);
});
client.Dispatcher.on(Events.MESSAGE_CREATE, e => {
var content = e.message.content;
if(content.indexOf("$price ") == 0) {
var coin = content.replace("$price ", "");
var value = '';
try{
request('http://api.coinmarketcap.com/v1/ticker/' + coin + '/',
function(error,res,body) {
var obj = JSON.parse(body);
console.log(obj[0]);
if(obj[0] === undefined)
{
e.message.channel.sendMessage("You have entered a wrong id");
}
else
{
value = coin.toUpperCase() +
" : Current Price " + obj[0].price_usd +
" | 24hr Percentage Change " + obj[0].percent_change_24h;
e.message.channel.sendMessage(value);
}
});
}
catch (err) {
e.message.channel.sendMessage("Wrong ID, Have a Great Day");
}
}
});
In the above code at first, you need to connect to the Discord client using the token of your app. Now once it is connected it will emit an event and write it to the console that my bot is connected. Next, we will check which cryptocurrency the user has typed after the $price and get the price of that cryptocurrency using the coinmarketcap api.
Now run the index.js file using command node index.js as shown below:
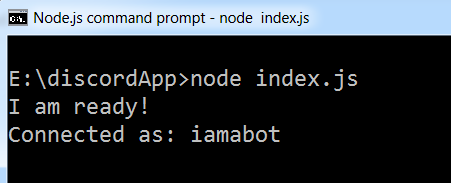
Add bot to the Server
Once Connected we need to add this bot to our server. For this, you need to take the Client ID of the bot and add it to the below url
https://discordapp.com/oauth2/authorize?&client_id=ClientId&scope=bot
When you open the above link it will ask which server you want to add the bot too. Note that you can only add this bot to that server which you are managing, other servers will not be shown.
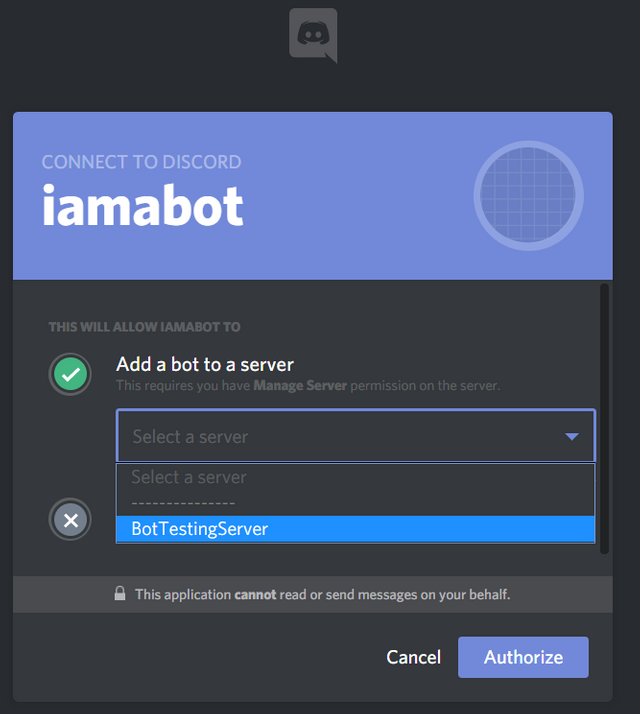
Once added you can see it on the right-hand side, now time to play with our new bot. See in the below pic, it's giving me the output.
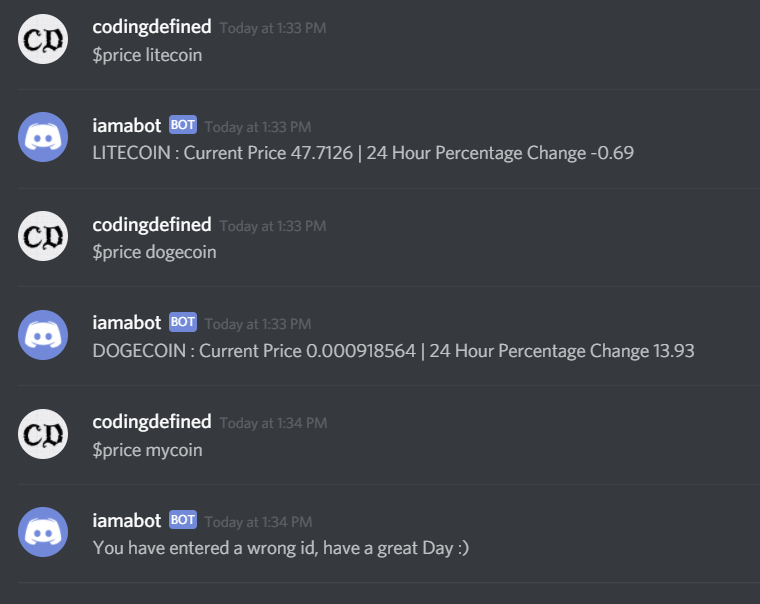
There are a lot of improvements which need to be done on this bot. If you have any suggestions or comments, please do comment.
Fantastic post! I always wondered how these fun bots were created. Thank you for the incredibly informative tutorial!
Thanks @karencarrens, it is just a simple bot which will give you the price. We can do a lot of things in this bot :)
You've received a FULL upvote from #TheUnmentionables - a SteemIt community full of members who like to kick ass, take names, and occasionally do it wearing (or forgetting to wear) our unmentionables...
Click the Image to Join Our Discord Server:
Please upvote this comment so we can help our members grow faster!
I officially call 25% of your earnings for the idea ;)
That's true, more than the earnings it is a learning which I have shared fully 100% :)
Shame on you @unmentionable! 😜
Very useful, Upvoted and following :)
Thanks @techtek :)
very nessery post ...very wall..
Thanks @johnmarteen :)
Hi @codingdefined,
Congrats! Your post has been featured in The Daily Qurator# 17
Dr. TLK :)
Thanks @theleapingkoala, its a good feeling to be a part of the curation list :)
You are very welcome :)
You are doing an amazing job brother for the Unmentionables group. I love the bots you implemented and created. They take so much work of the zoo-keepers and simplify the workload in the group. Plus bots are pretty funny. I asked him funny questions and got some funny responses. lol
Keep up the amazing job you are doing. I don't know anything about programming or bots so I really can't suggest anything useful. Have an amazing day brother. :)
Thanks @awakentolife, I am also in the learner phase. Might be one day I can make something useful for everyone.
You already did. You made some great bots for the group. Everybody is in learner phase their entire life. There is always something new to learn in life. It is just life. :)
That's absolutely true my friend :)
Congratulations to you , Thanks for sharing your knowledge with others
Thanks @mahimeena
Amazing information about bots . I don't know anything about coding and progamming . But from this i know quite about bots.
Yes @pawanregmi28, you can get started with the Bot very easily. Just wanted to share how easy is to set it up.
Congrats on the @curie nomination; 22% is a pretty big upvote on that trail. You've been doing great work, keep it up!
Thanks @olyup, it means a lot :)