Suesa's Adventures in Programming (Part 1 of ?)
By geralt on pixabay.com
As some of you know, I recently finished my B.Sc. in biology. As fewer of you know, I will start my Master's not this, but next year. This leaves me some time for other things (like working part-time in a biopharmaceutical lab, being the COO of Utopian, you know, mundane stuff).
For my biopharmaceutical lab job, I needed to be enrolled as a student, because else they'd have to pay me more, and thus not hire me. Capitalism in action.
This left me with the question what I should enroll in. Of course, I wouldn't need to actually pass the class, but the thought of not accomplishing anything useful frustrated me, which led to the decision of enrolling as a computer science student and taking programming classes.
Now, the classes use SML, a functional programming language that I won't really use in my life.
But
SML is a good basis to get a general understanding for how programming works. It's very mathematical and will (hopefully) teach me how to think in code.
And because nothing is really accomplished if it hasn't been told to anyone, I've decided to share some of the things I'm doing for those classes. On this blog, because I don't think it's high quality enough for my main blog.
Still, I'll write this with the assumption that those reading it can't code, because why would you want to read this otherwise?
- Task 1: Write a function that gives you the highest of three numbers. Use conditionals.
A "conditional" is the "if - then - else" construct, and one of the things that are easiest to understand (in my opinion), because it's basically "normal" language. But be careful, in case you're dating a programmer because the conditional might get you some unwanted results.
"Go to the supermarket. If
they have eggs then
bring 10 else
try another supermarket." Will get you the 10 eggs.
"Go to the supermarket. Buy milk, if
they have eggs then
bring 10, else
try another supermarket." Will get you 10 cartons of milk, if the supermarket had eggs.
For my "give me the highest number" function, I chose x,y,z
as variables, then checked if x is bigger than both y and z. If it was not, I compared y and z to see which of those is higher. Easy.
- Task 2: Calculate the cross sum of a number, once using a recursion and once using a tail recursion."
What's a recursion? Basically, you calculate something, take the result, put it in your original statement, and do the whole process all over again, until you reach a specific result.
A tail recursion is similar, but you don't have to "remember" the result you got before, which uses up fewer resources.
What I did here first is the recursion. x div 10
means I divide x by 10, and all I get is the number with the decimals shaved off. For example, 21 div 10
would give me a 2
.
x mod 10
is similar, just the other way around: It gives me what's left after the divison. 21 mod 10
would give me 1
.
So, quer
(for "Quersumme", which is German for "cross sum") first checks if x div 10
is smaller than 1
(which would be the case for all (positive) numbers between 1 and 9). If that's the case, it gives back exactly this number.
If not, the function uses itself again, but changes x
to x div 10
(example: 12
becomes 1
) and adds x mod 10
(in this example, creating the formula quer (1) + 2
).
Then it starts over. Now, 1 div 10
is smaller than 1
, so we keep the 1
. We now have 1+2
. The result? 3
. We successfully calculated the cross sum!
The tail recursion quer2
does something similar, with one small difference: We don't add a + something
to the function, which allows us to throw everything that happened before out.
In the first step, it again tests if x mod 10
is smaller than 1
and gives out x
if that's the case. So far, so good.
But if x mod 10
isn't smaller than 1
, the"help function" quer'
comes into play.
What does quer'
do? In contrary to quer
and quer2
it has two variables, x
and y
.
If you take a peek at the examples that come after, you'll see that I set the value of y
to 0
.
Now, let's take an x for which x div 10 >= 1
and see what quer'
does with that.
quer'(12,0)
12 mod 10 < 1 ?
-> 12 mod 10 = 2, false!
-> change quer'(12,0) to quer'(12 div 10, 0 + 12 mod 10)
(repeat from the beginning with new values)
quer'(1,2)
-> 1 mod 10 < 1 ?
-> 1 mod 10 = 1, false!
-> change quer'(1,2) to quer'(1 div 10, 2 + 1 mod 10)
(repeat from the beginning with new values)
quer'(0,3)
-> 0 mod 10 < 1?
-> 0 mod 10 = 0, true!
-> 3 + 0 mod 10
-> 3
Aaaaand we have our result!
There's a couple more exercises I already did, but I think I should split it up in more posts, as it might get a bit overloaded.
Feel free to ask questions in the comments.
Very cool to see that you've taken up programming! Interesting choice of language to start with. I've never actually seen this one, but it seems that it is popular in the theoretical realm of things :)
Good luck on your adventures!
The choice of SML is to have a pure functional language where it is rather unlikely that any students have previous experience with it. Thus giving everyone some fair ground to learn together.
Languages like python, haskell or scheme might be popular enough to be known by many people. Whereas scheme is still pretty nice for teaching.
Apparently next year we'll learn C and Java. Mostly Java. We'll see :D
My 2c worth. Python is a useful language to learn as it is so widely used as an automation tool for so many applications. You could use it in your masters, as well as automation with the Steem based platform and many other applications you might not think of just yet. Once you learn SML, the other languages should be relatively easy to learn.
There are many useful languages out there, depending mostly on what you want to do with them. Python, R and Matlab are very interesting for biology, that is for sure.
SME (subject-matter expert) that has hands-on experience about software development and programming is pretty rare but really needed in most industries. Keep it up because the industries that aim to make a big impact (not sure if I could say the world 😂) needs that.
That's what I thought, too many people focus only on their field of expertise, and neglect everything else. Especially in our time, programming is something that one should at least know some basics of, imo.
Also, try to learn low-level architecture a little bit like "how the program gets run", "what's the magic behind that", etc. Sometimes it really helps a lot when facing strange cases.
Always keeping my eyes and ears open :)
Tbh, I've seen biology students fail to use "ctrl+x, ctrl+v". I think I'm rather... advanced compared to that.
Sadly.
For keyboard shortcut, I understand that 😄. Probably they get used working on their phone or tablet which doesn't have keys like ctrl, alt, and Esc. Only shift and space that matters 😅
Just info, this is why most of the web or mobile apps provide auto-copy text/link/password.
Well, I only have one question. Can this programming language be used for anything, and in any type of project?
SML is a relatively pure language, so it is with less clutter, great for teaching but nit good for real world problems. It can be used but it would be a poor choice.
But I suppose then that the one who learns the SML language can program whatever, given the difficulty that it presents.
It is a normal functional language :) Not harder than any other ;)
Well, all serious computer science students I've talked to so far (you know, those who don't just take the programming courses but also all the other stuff attached to the degree) think that SML is horrible to actually work with. I haven't really met many people who've used. I guess it's a bit impractical for actual projects and more suitable to understand the concepts of programming.
That is, it is more advisable to use SML more to practice programming. It shows that it is very complicated to do.
I'd say the best way to learn programming is not about the language but about the concepts. Once you understand them, all languages become tools and learning a new one is as easy as learning it's quirks and syntax. But if all you know is a hammer, everything becomes a nail.
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
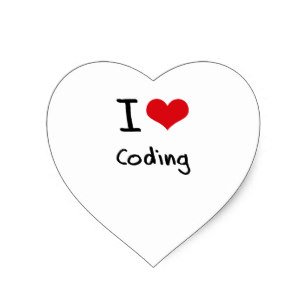
Reply !stop to disable the comment. Thanks!
Hey, that's interesting! I started to learn some coding myself, too, recently, just some basic HTML, CSS, JS, and now C++.
Just gotta buy a notebook to write stuff up and through to remember better and practice, practice, practice.
To listen to the audio version of this article click on the play image.
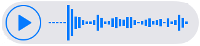
Brought to you by @tts. If you find it useful please consider upvoting this reply.