Let's Build a Modern Website from Scratch #2 - Preparing the Build System
Hello, everyone! Welcome back to this series of tutorials in which we are going to build a modern looking website for a web design company from the ground up, using latest tools in front-end development.
In the introduction post, I showed you how the final version is going to look like.
Website content
It will have landing page with hero section on the top, services and recent work. About page will contain some basic information about the company and the team members area. We'll create blog page where they can share latest news or industry trends. Portfolio page will showcase their most important projects. And of course, we will create contact page with contact form, contact information and a Google map.
In the first lesson we created our initial folder and file structure that looks like this:
webstudios\
app\
css\
fonts\
images\
js\
scss\
index.html
package.json
Now we have to introduce build system in our application.
Gulp
In a modern web development workflow some kind of build system is practically mandatory. It gives us benefits like live reloading the browser as we type in text editor, compiling SASS files into CSS, running the local server, optimizing images, minifying files and much more.
In our project we will use Gulp
which runs on node. It will automate our process for us and rerun it every time we make a change in code.
Unlike Grunt which uses json
syntax for writing tasks, Gulp is based on pure Javascript. We need to create gulpfile.js
in the root folder of our project. Near the top of the file we need to define dependencies such as gulp or any other we're gonna use. We use Require.js syntax for that and it looks like this:
var gulp = require('gulp');
Here are the packages we're interested in at the moment:
var gulp = require('gulp'),
prefix = require('gulp-autoprefixer');
sass = require('gulp-sass'),
plumber = require('gulp-plumber'),
browserSync = require('browser-sync');
gulp-autoprefixer is a plugin that adds necessary prefixes to CSS rules so the code is working the same in all browsers and it's supporting old ones. That way we don't have to write all the prefixes ourselves. So instead of typing:
.container {
display: -webkit-box;
display: -ms-flexbox;
display: -webkit-flex;
display: flex;
}
We just type:
.container {
display: flex;
}
to make our container use flexbox layout model.
gulp-plumber prevents our pipe process from breaking on error. In other words, we won't have to restart our gulp watch every time an error occurs.
browser-sync reloads the browser automatically when he notice any changes in the files we specified.
gulp-sass as you might guess, compiles .scss
files into .css
files that browser can read.
The tasks for deployment (publishing optimized website after it's finished) we will add in one of future lessons.
Let's install these four packages by going to our project root folder using terminal and typing:
npm install gulp-auroprefixer gulp-plumber browser-sync gulp-sass --save-dev
Make sure to add --save-dev
at the end to add these packages as dev-dependencies inside our package.json
file.
Now that we have installed the dependencies, let's see how our gulpfile.js
looks like (For each task you can see a possible template on the links above):
// gulpfile.js
// process sass
gulp.task('sass', function () {
return gulp.src('app/scss/**/*.scss')
.pipe(plumber({
errorHandler: function(err) {
console.log(err);
this.emit('end') }
}))
.pipe(sass())
.pipe(prefix('last 2 versions'))
.pipe(gulp.dest('app/css'))
.pipe(browserSync.stream())
});
// live reload
gulp.task('browserSync', function () {
browserSync({
server: {
baseDir: 'app'
}
})
});
// watch
gulp.task('watch', ['browserSync', 'sass'], function () {
gulp.watch('app/scss/**/*.scss', ['sass']);
gulp.watch("app/*.html").on('change', browserSync.reload);
});
Google fonts
Let's make our website more beautiful by installing couple of open source Google web fonts. You can find them on this address. Generally, you don't want to use too many different fonts when building a website or web app. Rather, choose two or three fonts (even three is a crowd sometimes), one for the headlines, another for content, articles and similar. That way it's more consistent and has a visual hierarchy.
Browse through the catalog and choose the ones you like. Those extravagant and unusual ones can be tempting, but you should stick to the popular fonts, located near the top. For this project, I will use Open sans, Lato
and Lora
. Customize the selection by choosing different font styles and weights and copy the import link that should look something like this:
@import url('https://fonts.googleapis.com/css?family=Lato:400,400i,700,700i|Lora:400,400i,700,700i|Open+Sans:400,400i,700,700i');
Now inside the scss
folder let's create new file and call it _base.scss
. Inside this file we will write some general styles for our website referring to all pages. Make sure to write a comment at the start of each file describing what's in it. For example:
/*======================================
= General styles =
======================================*/
And paste the font import link inside.
Settings file
Last thing we're gonna do in this lesson is create _settings.scss
file for the SASS variables. Variables give your app consistency when it comes to colors, font sizes, margins and other. This is one of the many advantages of SASS comparing to plain CSS. You can create variable by adding $
in from of the variable name:
$content-margin: 24px;
So, let's create some variables for the colors, margins and fonts. This is how our color palette looks like
// _settings.scss
// colors
$black: #000;
$lighter-black: #222;
$dark-grey: #666;
$grey: #AAA;
$light-grey: #d5d5d5;
$darker-white: #f5f5f5;
$white: #fff;
$dark: #252a30;
$red: #DB2E5F;
$light-red: #F54778;
$dark-red: #BD2852;
// fonts and margins
$base-font-size: 16px;
$baseline: $base-font-size * 1.5;
$theme-margin: $baseline * 2;
$medium-padding: 16px;
$mobile-padding: 8px;
$container: 1170px;
// font-family
$font-body: "Open sans", Lato, "Helvetica Neue", sans-serif;
$font-h: Lato, "Open sans", "Helvetica Neue";
$font-italic: Lora, serif;
It's up to you how you want to call your variables. You can use the style above and name it, let's say, $dark-grey
or you can name them based on their purpose or role, like $light-text-color
, $dark-border
, etc.
Next time...
.. we will start coding the homepage! See you then.
Thanks for reading! Upvote and resteem the post if you like it. And make sure to follow @alcibiades to stay updated about future lessons. I'm going to make a video series about this topic, but more on that soon.
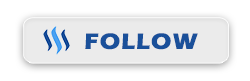
+1 For using a build management software like Gulp :) You also gained a new follower!