Discord Embed Reply with User Info | Steem Discord Bot Python #7
Welcome back, developers.
Greetings to all Steem enthusiasts! Today, We will learn how to access Steem global properties, convert vests to Steem, and set the embedded Discord message.
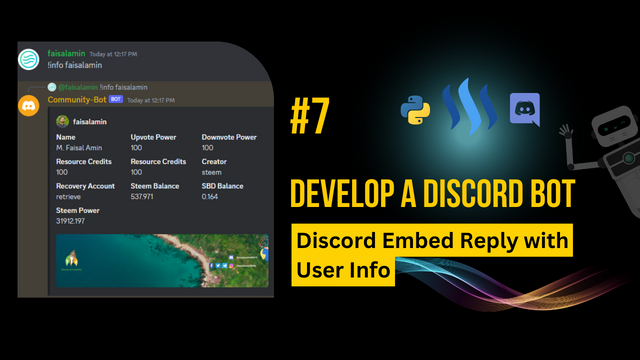
Summary |
---|
We established the necessary environment for developing a Python-based Discord bot during our first lecture. In our second lecture we create a discord bot token, configure the bot and generate the invite link. We used the invite link and added the bot to our server. In our third lecture, we will bring the Community-Bot online in our local environment.
In the 4th lecture, we successfully developed our initial bot command, !info. We also learned how to create functions and retrieve data using the requests library in 5th lecture. We implemented the !info command in 6th lecture which is used fetch the information of any steem user. Today we will extract some useful data and use the Discord Embed message to present it beautifully.
Procedure |
---|
- We will be setting an embedded message in Discord. It is a simple task, but before we proceed, we need to obtain the Steem Global Properties. Let's create an async function called get_steem_props in steemfun.py. This function will be extremely useful and can be utilized throughout the entire project.
async def get_steem_props(self):
api = self.utils.sds_base + "/steem_requests_api/getSteemProps"
response = requests.get(api).json()
return map_sds_response(response)
- By using this function we need to create a method that will be used to convert the Vests to Steem Power.
async def vest_to_steem(self, vests):
steem_props = await self.get_steem_props()
# noinspection PyTypeChecker
return vests * steem_props['steem_per_share']
- Now, we must navigate to our !info command code and retrieve the user's data. However, this time we will generate an embed message. To ensure the main code remains organized and easy to maintain, we will create a method specifically for generating the embedded message.
# !info command
if command == utils.commands[0]:
if len(props) >= 2:
username = str(props[1]).lower().replace('@', '')
await message.channel.typing()
try:
account_data = await steemfun.get_account_ext(username)
embed = await steemfun.generate_info_embed(account_data)
await message.reply(embed=embed)
except Exception as e:
await message.channel.send("Error: " + str(e))
- Add the stemit_base URL in utils.py.
# steemit base url
self.steemit_base = 'https://steemit.com'
- Here we are utilizing the generate_info_embed method. Rest assured, we are currently constructing it in the steemfun.py file. Within this method, we will manage any errors and provide a discord embed message as output. Retrieving the cover_image and user name may result in an error, as some users may not have set these attributes. Therefore, we need to implement a try-except block to handle this situation.
async def generate_info_embed(self, account_data):
embed = discord.Embed()
name = account_data['name']
if account_data['posting_json_metadata']:
posting_json = json.loads(account_data['posting_json_metadata'])
if posting_json['profile']:
try:
user_cover = posting_json['profile']['cover_image']
user_name = posting_json['profile']['name']
if user_cover is not None:
embed.set_image(url=user_cover)
if user_name is not None:
embed.add_field(name='Name', value=user_name)
except Exception:
pass
user_avatar = 'https://steemitimages.com/u/' + name + '/avatar/small'
profile_url = self.utils.steemit_base + '/@' + name
steem_power = await self.vest_to_steem(account_data['vests_own'])
embed.set_author(name=name, url=profile_url, icon_url=user_avatar)
embed.add_field(name='Upvote Power', value=account_data['upvote_mana_percent'])
embed.add_field(name='Downvote Power', value=account_data['downvote_mana_percent'])
embed.add_field(name='Resource Credits', value=account_data['rc_mana_percent'])
embed.add_field(name='Resource Credits', value=account_data['rc_mana_percent'])
embed.add_field(name='Creator', value=account_data['creator'])
embed.add_field(name='Recovery Account', value=account_data['recovery_account'])
embed.add_field(name='Steem Balance', value=account_data['balance_steem'])
embed.add_field(name='SBD Balance', value=account_data['balance_sbd'])
# fixing 3 decimal points of Steem Power
embed.add_field(name='Steem Power', value=f"{steem_power:.3f}")
return embed
- We have successfully imported this method into the main.py file, allowing us to use it without encountering any errors. Let's execute the project and verify its functionality.
Github |
---|
Steem Discord Bot Series |
---|
Lecture #1: Develop a Discord bot for your Steem Community
Lecture #2: How to Add a Bot to Your Discord Server
Lecture #3: Bring the Steem Discord Bot Online
Lecture #4: First Bot Command
lecture #5: Retrieving Steemit Account Information using SDS
Lecture #6: Implementing !info Command
SteemPro Official |
---|
Download SteemPro Mobile
https://play.google.com/store/apps/details?id=com.steempro.mobileVisit here.
https://www.steempro.comSteemPro Discord
Official Discord Server
Cc: @blacks
Cc: @rme
Cc: @hungry-griffin
Cc: @steemchiller
Cc: @steemcurator01
Cc: @pennsif
Cc: @future.witness
Cc: @stephenkendal
Cc: @justyy
Best Regards @faisalamin